Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
Core.h
00001 // This file is part of Eigen, a lightweight C++ template library 00002 // for linear algebra. 00003 // 00004 // Copyright (C) 2008 Gael Guennebaud <gael.guennebaud@inria.fr> 00005 // Copyright (C) 2007-2011 Benoit Jacob <jacob.benoit.1@gmail.com> 00006 // 00007 // This Source Code Form is subject to the terms of the Mozilla 00008 // Public License v. 2.0. If a copy of the MPL was not distributed 00009 // with this file, You can obtain one at http://mozilla.org/MPL/2.0/. 00010 00011 #ifndef EIGEN_CORE_H 00012 #define EIGEN_CORE_H 00013 00014 // first thing Eigen does: stop the compiler from committing suicide 00015 #include "src/Core/util/DisableStupidWarnings.h" 00016 00017 // then include this file where all our macros are defined. It's really important to do it first because 00018 // it's where we do all the alignment settings (platform detection and honoring the user's will if he 00019 // defined e.g. EIGEN_DONT_ALIGN) so it needs to be done before we do anything with vectorization. 00020 #include "src/Core/util/Macros.h" 00021 00022 // Disable the ipa-cp-clone optimization flag with MinGW 6.x or newer (enabled by default with -O3) 00023 // See http://eigen.tuxfamily.org/bz/show_bug.cgi?id=556 for details. 00024 #if defined(__MINGW32__) && EIGEN_GNUC_AT_LEAST(4,6) 00025 #pragma GCC optimize ("-fno-ipa-cp-clone") 00026 #endif 00027 00028 #include <complex> 00029 00030 // this include file manages BLAS and MKL related macros 00031 // and inclusion of their respective header files 00032 #include "src/Core/util/MKL_support.h" 00033 00034 // if alignment is disabled, then disable vectorization. Note: EIGEN_ALIGN is the proper check, it takes into 00035 // account both the user's will (EIGEN_DONT_ALIGN) and our own platform checks 00036 #if !EIGEN_ALIGN 00037 #ifndef EIGEN_DONT_VECTORIZE 00038 #define EIGEN_DONT_VECTORIZE 00039 #endif 00040 #endif 00041 00042 #ifdef _MSC_VER 00043 #include <malloc.h> // for _aligned_malloc -- need it regardless of whether vectorization is enabled 00044 #if (_MSC_VER >= 1500) // 2008 or later 00045 // Remember that usage of defined() in a #define is undefined by the standard. 00046 // a user reported that in 64-bit mode, MSVC doesn't care to define _M_IX86_FP. 00047 #if (defined(_M_IX86_FP) && (_M_IX86_FP >= 2)) || defined(_M_X64) 00048 #define EIGEN_SSE2_ON_MSVC_2008_OR_LATER 00049 #endif 00050 #endif 00051 #else 00052 // Remember that usage of defined() in a #define is undefined by the standard 00053 #if (defined __SSE2__) && ( (!defined __GNUC__) || (defined __INTEL_COMPILER) || EIGEN_GNUC_AT_LEAST(4,2) ) 00054 #define EIGEN_SSE2_ON_NON_MSVC_BUT_NOT_OLD_GCC 00055 #endif 00056 #endif 00057 00058 #ifndef EIGEN_DONT_VECTORIZE 00059 00060 #if defined (EIGEN_SSE2_ON_NON_MSVC_BUT_NOT_OLD_GCC) || defined(EIGEN_SSE2_ON_MSVC_2008_OR_LATER) 00061 00062 // Defines symbols for compile-time detection of which instructions are 00063 // used. 00064 // EIGEN_VECTORIZE_YY is defined if and only if the instruction set YY is used 00065 #define EIGEN_VECTORIZE 00066 #define EIGEN_VECTORIZE_SSE 00067 #define EIGEN_VECTORIZE_SSE2 00068 00069 // Detect sse3/ssse3/sse4: 00070 // gcc and icc defines __SSE3__, ... 00071 // there is no way to know about this on msvc. You can define EIGEN_VECTORIZE_SSE* if you 00072 // want to force the use of those instructions with msvc. 00073 #ifdef __SSE3__ 00074 #define EIGEN_VECTORIZE_SSE3 00075 #endif 00076 #ifdef __SSSE3__ 00077 #define EIGEN_VECTORIZE_SSSE3 00078 #endif 00079 #ifdef __SSE4_1__ 00080 #define EIGEN_VECTORIZE_SSE4_1 00081 #endif 00082 #ifdef __SSE4_2__ 00083 #define EIGEN_VECTORIZE_SSE4_2 00084 #endif 00085 00086 // include files 00087 00088 // This extern "C" works around a MINGW-w64 compilation issue 00089 // https://sourceforge.net/tracker/index.php?func=detail&aid=3018394&group_id=202880&atid=983354 00090 // In essence, intrin.h is included by windows.h and also declares intrinsics (just as emmintrin.h etc. below do). 00091 // However, intrin.h uses an extern "C" declaration, and g++ thus complains of duplicate declarations 00092 // with conflicting linkage. The linkage for intrinsics doesn't matter, but at that stage the compiler doesn't know; 00093 // so, to avoid compile errors when windows.h is included after Eigen/Core, ensure intrinsics are extern "C" here too. 00094 // notice that since these are C headers, the extern "C" is theoretically needed anyways. 00095 extern "C" { 00096 // In theory we should only include immintrin.h and not the other *mmintrin.h header files directly. 00097 // Doing so triggers some issues with ICC. However old gcc versions seems to not have this file, thus: 00098 #if defined(__INTEL_COMPILER) && __INTEL_COMPILER >= 1110 00099 #include <immintrin.h> 00100 #else 00101 #include <emmintrin.h> 00102 #include <xmmintrin.h> 00103 #ifdef EIGEN_VECTORIZE_SSE3 00104 #include <pmmintrin.h> 00105 #endif 00106 #ifdef EIGEN_VECTORIZE_SSSE3 00107 #include <tmmintrin.h> 00108 #endif 00109 #ifdef EIGEN_VECTORIZE_SSE4_1 00110 #include <smmintrin.h> 00111 #endif 00112 #ifdef EIGEN_VECTORIZE_SSE4_2 00113 #include <nmmintrin.h> 00114 #endif 00115 #endif 00116 } // end extern "C" 00117 #elif defined __ALTIVEC__ 00118 #define EIGEN_VECTORIZE 00119 #define EIGEN_VECTORIZE_ALTIVEC 00120 #include <altivec.h> 00121 // We need to #undef all these ugly tokens defined in <altivec.h> 00122 // => use __vector instead of vector 00123 #undef bool 00124 #undef vector 00125 #undef pixel 00126 #elif defined __ARM_NEON 00127 #define EIGEN_VECTORIZE 00128 #define EIGEN_VECTORIZE_NEON 00129 #include <arm_neon.h> 00130 #endif 00131 #endif 00132 00133 #if (defined _OPENMP) && (!defined EIGEN_DONT_PARALLELIZE) 00134 #define EIGEN_HAS_OPENMP 00135 #endif 00136 00137 #ifdef EIGEN_HAS_OPENMP 00138 #include <omp.h> 00139 #endif 00140 00141 // MSVC for windows mobile does not have the errno.h file 00142 #if !(defined(_MSC_VER) && defined(_WIN32_WCE)) && !defined(__ARMCC_VERSION) 00143 #define EIGEN_HAS_ERRNO 00144 #endif 00145 00146 #ifdef EIGEN_HAS_ERRNO 00147 #include <cerrno> 00148 #endif 00149 #include <cstddef> 00150 #include <cstdlib> 00151 #include <cmath> 00152 #include <cassert> 00153 #include <functional> 00154 #include <iosfwd> 00155 #include <cstring> 00156 #include <string> 00157 #include <limits> 00158 #include <climits> // for CHAR_BIT 00159 // for min/max: 00160 #include <algorithm> 00161 00162 // for outputting debug info 00163 #ifdef EIGEN_DEBUG_ASSIGN 00164 #include <iostream> 00165 #endif 00166 00167 // required for __cpuid, needs to be included after cmath 00168 #if defined(_MSC_VER) && (defined(_M_IX86)||defined(_M_X64)) && (!defined(_WIN32_WCE)) 00169 #include <intrin.h> 00170 #endif 00171 00172 #if defined(_CPPUNWIND) || defined(__EXCEPTIONS) 00173 #define EIGEN_EXCEPTIONS 00174 #endif 00175 00176 #ifdef EIGEN_EXCEPTIONS 00177 #include <new> 00178 #endif 00179 00180 /** \brief Namespace containing all symbols from the %Eigen library. */ 00181 namespace Eigen { 00182 00183 inline static const char *SimdInstructionSetsInUse(void) { 00184 #if defined(EIGEN_VECTORIZE_SSE4_2) 00185 return "SSE, SSE2, SSE3, SSSE3, SSE4.1, SSE4.2"; 00186 #elif defined(EIGEN_VECTORIZE_SSE4_1) 00187 return "SSE, SSE2, SSE3, SSSE3, SSE4.1"; 00188 #elif defined(EIGEN_VECTORIZE_SSSE3) 00189 return "SSE, SSE2, SSE3, SSSE3"; 00190 #elif defined(EIGEN_VECTORIZE_SSE3) 00191 return "SSE, SSE2, SSE3"; 00192 #elif defined(EIGEN_VECTORIZE_SSE2) 00193 return "SSE, SSE2"; 00194 #elif defined(EIGEN_VECTORIZE_ALTIVEC) 00195 return "AltiVec"; 00196 #elif defined(EIGEN_VECTORIZE_NEON) 00197 return "ARM NEON"; 00198 #else 00199 return "None"; 00200 #endif 00201 } 00202 00203 } // end namespace Eigen 00204 00205 #define STAGE10_FULL_EIGEN2_API 10 00206 #define STAGE20_RESOLVE_API_CONFLICTS 20 00207 #define STAGE30_FULL_EIGEN3_API 30 00208 #define STAGE40_FULL_EIGEN3_STRICTNESS 40 00209 #define STAGE99_NO_EIGEN2_SUPPORT 99 00210 00211 #if defined EIGEN2_SUPPORT_STAGE40_FULL_EIGEN3_STRICTNESS 00212 #define EIGEN2_SUPPORT 00213 #define EIGEN2_SUPPORT_STAGE STAGE40_FULL_EIGEN3_STRICTNESS 00214 #elif defined EIGEN2_SUPPORT_STAGE30_FULL_EIGEN3_API 00215 #define EIGEN2_SUPPORT 00216 #define EIGEN2_SUPPORT_STAGE STAGE30_FULL_EIGEN3_API 00217 #elif defined EIGEN2_SUPPORT_STAGE20_RESOLVE_API_CONFLICTS 00218 #define EIGEN2_SUPPORT 00219 #define EIGEN2_SUPPORT_STAGE STAGE20_RESOLVE_API_CONFLICTS 00220 #elif defined EIGEN2_SUPPORT_STAGE10_FULL_EIGEN2_API 00221 #define EIGEN2_SUPPORT 00222 #define EIGEN2_SUPPORT_STAGE STAGE10_FULL_EIGEN2_API 00223 #elif defined EIGEN2_SUPPORT 00224 // default to stage 3, that's what it's always meant 00225 #define EIGEN2_SUPPORT_STAGE30_FULL_EIGEN3_API 00226 #define EIGEN2_SUPPORT_STAGE STAGE30_FULL_EIGEN3_API 00227 #else 00228 #define EIGEN2_SUPPORT_STAGE STAGE99_NO_EIGEN2_SUPPORT 00229 #endif 00230 00231 #ifdef EIGEN2_SUPPORT 00232 #undef minor 00233 #endif 00234 00235 // we use size_t frequently and we'll never remember to prepend it with std:: everytime just to 00236 // ensure QNX/QCC support 00237 using std::size_t; 00238 // gcc 4.6.0 wants std:: for ptrdiff_t 00239 using std::ptrdiff_t; 00240 00241 /** \defgroup Core_Module Core module 00242 * This is the main module of Eigen providing dense matrix and vector support 00243 * (both fixed and dynamic size) with all the features corresponding to a BLAS library 00244 * and much more... 00245 * 00246 * \code 00247 * #include <Eigen/Core> 00248 * \endcode 00249 */ 00250 00251 #include "src/Core/util/Constants.h" 00252 #include "src/Core/util/ForwardDeclarations.h" 00253 #include "src/Core/util/Meta.h" 00254 #include "src/Core/util/StaticAssert.h" 00255 #include "src/Core/util/XprHelper.h" 00256 #include "src/Core/util/Memory.h" 00257 00258 #include "src/Core/NumTraits.h" 00259 #include "src/Core/MathFunctions.h" 00260 #include "src/Core/GenericPacketMath.h" 00261 00262 #if defined EIGEN_VECTORIZE_SSE 00263 #include "src/Core/arch/SSE/PacketMath.h" 00264 #include "src/Core/arch/SSE/MathFunctions.h" 00265 #include "src/Core/arch/SSE/Complex.h" 00266 #elif defined EIGEN_VECTORIZE_ALTIVEC 00267 #include "src/Core/arch/AltiVec/PacketMath.h" 00268 #include "src/Core/arch/AltiVec/Complex.h" 00269 #elif defined EIGEN_VECTORIZE_NEON 00270 #include "src/Core/arch/NEON/PacketMath.h" 00271 #include "src/Core/arch/NEON/Complex.h" 00272 #endif 00273 00274 #include "src/Core/arch/Default/Settings.h" 00275 00276 #include "src/Core/Functors.h" 00277 #include "src/Core/DenseCoeffsBase.h" 00278 #include "src/Core/DenseBase.h" 00279 #include "src/Core/MatrixBase.h" 00280 #include "src/Core/EigenBase.h" 00281 00282 #ifndef EIGEN_PARSED_BY_DOXYGEN // work around Doxygen bug triggered by Assign.h r814874 00283 // at least confirmed with Doxygen 1.5.5 and 1.5.6 00284 #include "src/Core/Assign.h" 00285 #endif 00286 00287 #include "src/Core/util/BlasUtil.h" 00288 #include "src/Core/DenseStorage.h" 00289 #include "src/Core/NestByValue.h" 00290 #include "src/Core/ForceAlignedAccess.h" 00291 #include "src/Core/ReturnByValue.h" 00292 #include "src/Core/NoAlias.h" 00293 #include "src/Core/PlainObjectBase.h" 00294 #include "src/Core/Matrix.h" 00295 #include "src/Core/Array.h" 00296 #include "src/Core/CwiseBinaryOp.h" 00297 #include "src/Core/CwiseUnaryOp.h" 00298 #include "src/Core/CwiseNullaryOp.h" 00299 #include "src/Core/CwiseUnaryView.h" 00300 #include "src/Core/SelfCwiseBinaryOp.h" 00301 #include "src/Core/Dot.h" 00302 #include "src/Core/StableNorm.h" 00303 #include "src/Core/MapBase.h" 00304 #include "src/Core/Stride.h" 00305 #include "src/Core/Map.h" 00306 #include "src/Core/Block.h" 00307 #include "src/Core/VectorBlock.h" 00308 #include "src/Core/Ref.h" 00309 #include "src/Core/Transpose.h" 00310 #include "src/Core/DiagonalMatrix.h" 00311 #include "src/Core/Diagonal.h" 00312 #include "src/Core/DiagonalProduct.h" 00313 #include "src/Core/PermutationMatrix.h" 00314 #include "src/Core/Transpositions.h" 00315 #include "src/Core/Redux.h" 00316 #include "src/Core/Visitor.h" 00317 #include "src/Core/Fuzzy.h" 00318 #include "src/Core/IO.h" 00319 #include "src/Core/Swap.h" 00320 #include "src/Core/CommaInitializer.h" 00321 #include "src/Core/Flagged.h" 00322 #include "src/Core/ProductBase.h" 00323 #include "src/Core/GeneralProduct.h" 00324 #include "src/Core/TriangularMatrix.h" 00325 #include "src/Core/SelfAdjointView.h" 00326 #include "src/Core/products/GeneralBlockPanelKernel.h" 00327 #include "src/Core/products/Parallelizer.h" 00328 #include "src/Core/products/CoeffBasedProduct.h" 00329 #include "src/Core/products/GeneralMatrixVector.h" 00330 #include "src/Core/products/GeneralMatrixMatrix.h" 00331 #include "src/Core/SolveTriangular.h" 00332 #include "src/Core/products/GeneralMatrixMatrixTriangular.h" 00333 #include "src/Core/products/SelfadjointMatrixVector.h" 00334 #include "src/Core/products/SelfadjointMatrixMatrix.h" 00335 #include "src/Core/products/SelfadjointProduct.h" 00336 #include "src/Core/products/SelfadjointRank2Update.h" 00337 #include "src/Core/products/TriangularMatrixVector.h" 00338 #include "src/Core/products/TriangularMatrixMatrix.h" 00339 #include "src/Core/products/TriangularSolverMatrix.h" 00340 #include "src/Core/products/TriangularSolverVector.h" 00341 #include "src/Core/BandMatrix.h" 00342 #include "src/Core/CoreIterators.h" 00343 00344 #include "src/Core/BooleanRedux.h" 00345 #include "src/Core/Select.h" 00346 #include "src/Core/VectorwiseOp.h" 00347 #include "src/Core/Random.h" 00348 #include "src/Core/Replicate.h" 00349 #include "src/Core/Reverse.h" 00350 #include "src/Core/ArrayBase.h" 00351 #include "src/Core/ArrayWrapper.h" 00352 00353 #ifdef EIGEN_USE_BLAS 00354 #include "src/Core/products/GeneralMatrixMatrix_MKL.h" 00355 #include "src/Core/products/GeneralMatrixVector_MKL.h" 00356 #include "src/Core/products/GeneralMatrixMatrixTriangular_MKL.h" 00357 #include "src/Core/products/SelfadjointMatrixMatrix_MKL.h" 00358 #include "src/Core/products/SelfadjointMatrixVector_MKL.h" 00359 #include "src/Core/products/TriangularMatrixMatrix_MKL.h" 00360 #include "src/Core/products/TriangularMatrixVector_MKL.h" 00361 #include "src/Core/products/TriangularSolverMatrix_MKL.h" 00362 #endif // EIGEN_USE_BLAS 00363 00364 #ifdef EIGEN_USE_MKL_VML 00365 #include "src/Core/Assign_MKL.h" 00366 #endif 00367 00368 #include "src/Core/GlobalFunctions.h" 00369 00370 #include "src/Core/util/ReenableStupidWarnings.h" 00371 00372 #ifdef EIGEN2_SUPPORT 00373 #include "Eigen2Support" 00374 #endif 00375 00376 #endif // EIGEN_CORE_H
Generated on Tue Jul 12 2022 17:46:51 by
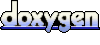