XBee API operation library for mbed
XBeeAPI Class Reference
XBee API library entry poiont. More...
#include <XBeeAPI.h>
Inherits CoreAPI.
Public Member Functions | |
XBeeAPI (PinName tx, PinName rx) | |
Create a XBeeAPI instance, with baud rate 9600 and no escape. | |
XBeeAPI (PinName tx, PinName rx, bool isEscape) | |
Create a XBeeAPI instance, with baud rate 9600. | |
XBeeAPI (PinName tx, PinName rx, int baudRate, bool isEscape) | |
Create a XBeeAPI instance. | |
XBeeAPI (ISerial *serial, bool isEscape) | |
Create a SerialData instance with other serial interface. | |
void | setVerifyChecksum (bool isCheck) |
Set whether to verify checksum during receiving, default is not verify. | |
void | send (APIFrame *request) |
A general function to send frame out. | |
APIFrame * | getResponse () |
Read the next avaliable API frame, and the type of fram can be retrieved from getFrameType(). | |
XBeeRx64Indicator * | getXBeeRx64 () |
Read the next avaliable API frame. | |
XBeeRx16Indicator * | getXBeeRx16 () |
Read the next avaliable API frame. | |
XBeeRx64IOSampleIndicator * | getXBeeRx64IOSample () |
Read the next avaliable API frame. | |
XBeeRx16IOSampleIndicator * | getXBeeRx16IOSample () |
Read the next avaliable API frame. | |
XBeeTxStatusIndicator * | getXBeeTxStatus () |
Read the next avaliable API frame. | |
ATCommandIndicator * | getATCommand () |
Read the next avaliable API frame. | |
ModemStatusIndicator * | getModemStatus () |
Read the next avaliable API frame. | |
ZigBeeTxStatusIndicator * | getZigBeeTxStatus () |
Read the next avaliable API frame. | |
ZigBeeRxIndicator * | getZigBeeRx () |
Read the next avaliable API frame. | |
ZigBeeExplicitRxIndicator * | getZigBeeExplicitRx () |
Read the next avaliable API frame. | |
ZigBeeIOSampleIndicator * | getZigBeeIOSample () |
Read the next avaliable API frame. | |
SensorReadIndicator * | getSensorRead () |
Read the next avaliable API frame. | |
NodeIdentificationIndicator * | getNodeIdentification () |
Read the next avaliable API frame. | |
RemoteCommandIndicator * | getRemoteCommand () |
Read the next avaliable API frame. | |
RouteRecordIndicator * | getRouteRecord () |
Read the next avaliable API frame. | |
ManyToOneRouteIndicator * | getManyToOneRoute () |
Read the next avaliable API frame. | |
ATCommandIndicator * | setPinFunction (Pin *pin, unsigned char function) |
RemoteCommandIndicator * | setRemotePinFunction (Address *remoteAddress, Pin *pin, unsigned char function) |
bool | forceXBeeLocalIOSample () |
The command will immediately return an "OK" response. | |
IOSamples * | forceZigBeeLocalIOSample () |
Return 1 IO sample from the local module. | |
IOSamples * | forceXBeeRemoteIOSample (Address *remote) |
Return 1 IO sample only, Samples before TX (IT) does not affect. | |
IOSamples * | forceZigBeeRemoteIOSample (Address *remote) |
Return 1 IO sample only. | |
Protected Member Functions | |
int | readByte () |
Read one byte payload, which allready handle the escape char, if less than 0 means error occured. | |
void | writeByte (unsigned char data) |
Write one byte to the payload, which allready handle the escape char. | |
void | packetProcess () |
Processing API frame. | |
int | getLength () |
Get the next avaliable API frame length. | |
void | readPayLoad (int length) |
Read the next avaliable API frame data. |
Detailed Description
XBee API library entry poiont.
Example:
#include "mbed.h" #include "XBeeAPI.h" XBeeAPI xbee(p9, p10, true); Address add(0x0013A200,0x406CABD,0x0456); XBeeTx16Request tx16(0x00, &add, OptionsBase::DEFAULT, "Hello From XBEE API", 0, 19); XBeeRx16Indicator rx16(NULL); int main() { xbee.setVerifyChecksum(false); // option 1 while(1) { XBeeRx16Indicator * response = xbee.getXBeeRx16(); if (response != NULL) { tx16.setPayload(response->getReceivedData(), 0, response->getReceivedDataLength()); xbee.send(&tx16); } } // option 2 while(1) { APIFrame * frame = xbee.getResponse(); while(frame != NULL) { switch (frame->getFrameType()) { case APIFrame::Tx64_Request : break; case APIFrame::Tx16_Request : break; case APIFrame::AT_Command: break; case APIFrame::AT_Command_Queue_Parameter_Value: break; case APIFrame::ZigBee_Transmit_Request: break; case APIFrame::Explicit_Addressing_ZigBee_Command_Frame: break; case APIFrame::Remote_Command_Request : break; case APIFrame::Create_Source_Route : break; case APIFrame::Register_Joining_Device: break; case APIFrame::Rx64_Receive_Packet : break; case APIFrame::Rx16_Receive_Packet: rx16.convert(frame); tx16.setPayload(rx16.getReceivedData(), 0, rx16.getReceivedDataLength()); xbee.send(&tx16); break; case APIFrame::Rx64_IO_Data_Sample_Rx_Indicator: break; case APIFrame::Rx16_IO_Data_Sample_Rx_Indicator : xbee.send(&tx16); break; case APIFrame::AT_Command_Response: break; case APIFrame::XBee_Transmit_Status : break; case APIFrame::Modem_Status: break; case APIFrame::ZigBee_Transmit_Status : break; case APIFrame::ZigBee_Receive_Packet: break; case APIFrame::ZigBee_Explicit_Rx_Indicator : break; case APIFrame::ZigBee_IO_Data_Sample_Rx_Indicator : break; case APIFrame::XBee_Sensor_Read_Indicato : break; case APIFrame::Node_Identification_Indicator: break; case APIFrame::Remote_Command_Response: break; case APIFrame::Over_the_Air_Firmware_Update_Status : break; case APIFrame::Route_Record_Indicator : break; case APIFrame::Device_Authenticated_Indicator: break; case APIFrame::Many_to_One_Route_Request_Indicator: break; } frame = xbee.getResponse(); } } }
Definition at line 104 of file XBeeAPI.h.
Constructor & Destructor Documentation
XBeeAPI | ( | PinName | tx, |
PinName | rx | ||
) |
Create a XBeeAPI instance, with baud rate 9600 and no escape.
- Parameters:
-
tx data transmission line rx data receiving line
Definition at line 3 of file XBeeAPI.cpp.
XBeeAPI | ( | PinName | tx, |
PinName | rx, | ||
bool | isEscape | ||
) |
Create a XBeeAPI instance, with baud rate 9600.
- Parameters:
-
tx data transmission line rx data receiving line isEscape API escaped operating mode (AP = 2) works similarly to API mode. The only difference is that when working in API escaped mode, some bytes of the API frame specific data must be escaped.
Definition at line 7 of file XBeeAPI.cpp.
XBeeAPI | ( | PinName | tx, |
PinName | rx, | ||
int | baudRate, | ||
bool | isEscape | ||
) |
Create a XBeeAPI instance.
- Parameters:
-
tx data transmission line rx data receiving line baudRate baud rate isEscape API escaped operating mode (AP = 2) works similarly to API mode. The only difference is that when working in API escaped mode, some bytes of the API frame specific data must be escaped.
Definition at line 11 of file XBeeAPI.cpp.
Create a SerialData instance with other serial interface.
- Parameters:
-
serial class that implementes ISerial interface. baudRate baud rate isEscape API escaped operating mode (AP = 2) works similarly to API mode. The only difference is that when working in API escaped mode, some bytes of the API frame specific data must be escaped.
Definition at line 15 of file XBeeAPI.cpp.
Member Function Documentation
bool forceXBeeLocalIOSample | ( | ) | [inherited] |
The command will immediately return an "OK" response.
The data will follow in the normal API format for DIO data event.
- Returns:
- true if the command is "OK", false if no IO is enabled.
Definition at line 514 of file CoreAPI.cpp.
Return 1 IO sample only, Samples before TX (IT) does not affect.
<param name="remote"Remote address of the device>
- Returns:
Definition at line 537 of file CoreAPI.cpp.
IOSamples * forceZigBeeLocalIOSample | ( | ) | [inherited] |
Return 1 IO sample only.
- Parameters:
-
remote Remote address of the device
- Returns:
Definition at line 544 of file CoreAPI.cpp.
ATCommandIndicator * getATCommand | ( | ) | [inherited] |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 156 of file CoreAPI.cpp.
int getLength | ( | ) | [protected, inherited] |
Get the next avaliable API frame length.
Definition at line 89 of file CoreAPI.cpp.
ManyToOneRouteIndicator * getManyToOneRoute | ( | ) | [inherited] |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 256 of file CoreAPI.cpp.
ModemStatusIndicator * getModemStatus | ( | ) | [inherited] |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 166 of file CoreAPI.cpp.
NodeIdentificationIndicator * getNodeIdentification | ( | ) | [inherited] |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 226 of file CoreAPI.cpp.
RemoteCommandIndicator * getRemoteCommand | ( | ) | [inherited] |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 236 of file CoreAPI.cpp.
APIFrame * getResponse | ( | ) | [inherited] |
Read the next avaliable API frame, and the type of fram can be retrieved from getFrameType().
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 68 of file CoreAPI.cpp.
RouteRecordIndicator * getRouteRecord | ( | ) | [inherited] |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 246 of file CoreAPI.cpp.
SensorReadIndicator * getSensorRead | ( | ) | [inherited] |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 216 of file CoreAPI.cpp.
XBeeRx16Indicator * getXBeeRx16 | ( | ) | [inherited] |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 116 of file CoreAPI.cpp.
XBeeRx16IOSampleIndicator * getXBeeRx16IOSample | ( | ) | [inherited] |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 136 of file CoreAPI.cpp.
XBeeRx64Indicator * getXBeeRx64 | ( | ) | [inherited] |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 106 of file CoreAPI.cpp.
XBeeRx64IOSampleIndicator * getXBeeRx64IOSample | ( | ) | [inherited] |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 126 of file CoreAPI.cpp.
XBeeTxStatusIndicator * getXBeeTxStatus | ( | ) | [inherited] |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 146 of file CoreAPI.cpp.
ZigBeeExplicitRxIndicator * getZigBeeExplicitRx | ( | ) | [inherited] |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 196 of file CoreAPI.cpp.
ZigBeeIOSampleIndicator * getZigBeeIOSample | ( | ) | [inherited] |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 206 of file CoreAPI.cpp.
ZigBeeRxIndicator * getZigBeeRx | ( | ) | [inherited] |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 186 of file CoreAPI.cpp.
ZigBeeTxStatusIndicator * getZigBeeTxStatus | ( | ) | [inherited] |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 176 of file CoreAPI.cpp.
void packetProcess | ( | ) | [protected, inherited] |
Processing API frame.
int readByte | ( | ) | [protected, inherited] |
Read one byte payload, which allready handle the escape char, if less than 0 means error occured.
- Returns:
- if less than 0 means error occured.
Definition at line 45 of file CoreAPI.cpp.
void readPayLoad | ( | int | length ) | [protected, inherited] |
Read the next avaliable API frame data.
Definition at line 98 of file CoreAPI.cpp.
void send | ( | APIFrame * | request ) | [inherited] |
A general function to send frame out.
- Parameters:
-
request any API frame
Definition at line 29 of file CoreAPI.cpp.
ATCommandIndicator * setPinFunction | ( | Pin * | pin, |
unsigned char | function | ||
) | [inherited] |
- Parameters:
-
function DISABLED = 0x00, RESERVED_FOR_PIN_SPECIFIC_ALTERNATE_FUNCTIONALITIES = 0x01, ANALOG_INPUT_SINGLE_ENDED = 0x02, DIGITAL_INPUT_MONITORED = 0x03, DIGITAL_OUTPUT_DEFAULT_LOW = 0x04, DIGITAL_OUTPUT_DEFAULT_HIGH = 0x05, ALTERNATE_FUNCTIONALITIES_WHERE_APPLICABLE = 0x06//0x06~0x09
Definition at line 494 of file CoreAPI.cpp.
RemoteCommandIndicator * setRemotePinFunction | ( | Address * | remoteAddress, |
Pin * | pin, | ||
unsigned char | function | ||
) | [inherited] |
- Parameters:
-
function DISABLED = 0x00, RESERVED_FOR_PIN_SPECIFIC_ALTERNATE_FUNCTIONALITIES = 0x01, ANALOG_INPUT_SINGLE_ENDED = 0x02, DIGITAL_INPUT_MONITORED = 0x03, DIGITAL_OUTPUT_DEFAULT_LOW = 0x04, DIGITAL_OUTPUT_DEFAULT_HIGH = 0x05, ALTERNATE_FUNCTIONALITIES_WHERE_APPLICABLE = 0x06//0x06~0x09
Definition at line 504 of file CoreAPI.cpp.
void setVerifyChecksum | ( | bool | isCheck ) | [inherited] |
Set whether to verify checksum during receiving, default is not verify.
- Parameters:
-
isCheck true only to process API frame when checksum matches. false ignore the checksum.
Definition at line 24 of file CoreAPI.cpp.
void writeByte | ( | unsigned char | data ) | [protected, inherited] |
Write one byte to the payload, which allready handle the escape char.
- Parameters:
-
data one byte [0x00-0xFF]
Definition at line 55 of file CoreAPI.cpp.
Generated on Tue Jul 12 2022 11:17:05 by
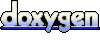