XBee API operation library for mbed
APIFrame Class Reference
An API frame is the structured data sent and received through the serial interface of the radio module when it is configured in API or API escaped operating modes. API frames are used to communicate with the module or with other modules in the network. More...
#include <APIFrame.h>
Inherits BufferedArray.
Inherited by RxBase, and TxBase.
Public Member Functions | |
unsigned char | getFrameType () |
Get the API frame type. | |
void | allocate (unsigned long length) |
Reset the raw data. | |
void | rewind () |
Reset the position and does not affect the content of the data. | |
void | set (unsigned char value) |
Write 8-bit data into current posiston and increase by 1. | |
void | sets (const unsigned char *value, unsigned long offset, unsigned long length) |
Write array of data into current posiston, and increase by the lenght. | |
void | set (unsigned long position, unsigned char value) |
Write 8-bit data into specific posiston and deos not affect the current position. | |
void | sets (unsigned long position, const unsigned char *value, unsigned long offset, unsigned long length) |
Write array of data into specific posiston and deos not affect the current position. | |
char | getCheckSum () |
Get checksum. | |
void | setCheckSum (unsigned char value) |
Set checksum. | |
bool | verifyChecksum () |
Check is the API frame's checksum is verified. | |
void | calculateChecksum () |
Calculate the checksum value. | |
unsigned char * | gets () |
Get the raw data. | |
unsigned char * | gets (unsigned long position) |
Get the raw data from a specific location. | |
unsigned char | get (unsigned long position) |
Get 1 byte data from a specific location. | |
int | getPosition () |
Get the current index. | |
void | setPosition (unsigned long position) |
Set the index within the max length of raw data. | |
Protected Attributes | |
unsigned int | expandSize |
initial size and automatically increase length. | |
unsigned char * | data |
Raw data. | |
unsigned long | index |
Current index of the data, could also used as data lendth. | |
unsigned long | max |
Max data size that the raw data can hold. |
Detailed Description
An API frame is the structured data sent and received through the serial interface of the radio module when it is configured in API or API escaped operating modes. API frames are used to communicate with the module or with other modules in the network.
Definition at line 7 of file APIFrame.h.
Member Function Documentation
void allocate | ( | unsigned long | length ) |
Reset the raw data.
- Parameters:
-
length current max size for the raw data
Reimplemented from BufferedArray.
Definition at line 26 of file APIFrame.cpp.
void calculateChecksum | ( | ) |
Calculate the checksum value.
Definition at line 101 of file APIFrame.cpp.
unsigned char get | ( | unsigned long | position ) | [inherited] |
Get 1 byte data from a specific location.
- Parameters:
-
position where to retrieve
- Returns:
- unsigned char.
Definition at line 39 of file BufferedArray.cpp.
char getCheckSum | ( | ) |
unsigned char getFrameType | ( | ) |
Get the API frame type.
- Returns:
- Tx64_Request =0x00, Tx16_Request =0x01, AT_Command = 0x08, AT_Command_Queue_Parameter_Value = 0x09, ZigBee_Transmit_Request = 0x10, Explicit_Addressing_ZigBee_Command_Frame = 0x11, Remote_Command_Request = 0x17, Create_Source_Route = 0x21, Register_Joining_Device = 0x24, Rx64_Receive_Packet = 0x80, Rx16_Receive_Packet = 0x81, Rx64_IO_Data_Sample_Rx_Indicator = 0x82, Rx16_IO_Data_Sample_Rx_Indicator = 0x83, AT_Command_Response = 0x88, XBee_Transmit_Status = 0x89, Modem_Status = 0x8A, ZigBee_Transmit_Status = 0x8B, ZigBee_Receive_Packet = 0x90, ZigBee_Explicit_Rx_Indicator = 0x91, ZigBee_IO_Data_Sample_Rx_Indicator = 0x92, XBee_Sensor_Read_Indicato = 0x94, Node_Identification_Indicator = 0x95, Remote_Command_Response = 0x97, Over_the_Air_Firmware_Update_Status = 0xA0, Route_Record_Indicator = 0xA1, Device_Authenticated_Indicator = 0xA2, Many_to_One_Route_Request_Indicator = 0xA3,
Definition at line 16 of file APIFrame.cpp.
int getPosition | ( | ) | [inherited] |
Get the current index.
- Returns:
- unsigned char array.
Definition at line 44 of file BufferedArray.cpp.
unsigned char * gets | ( | unsigned long | position ) | [inherited] |
Get the raw data from a specific location.
- Parameters:
-
position where to retrieve
- Returns:
- unsigned char array.
Definition at line 34 of file BufferedArray.cpp.
unsigned char * gets | ( | ) | [inherited] |
void rewind | ( | ) |
Reset the position and does not affect the content of the data.
- Parameters:
-
length current max size for the raw data
Reimplemented from BufferedArray.
Definition at line 32 of file APIFrame.cpp.
void set | ( | unsigned char | value ) |
Write 8-bit data into current posiston and increase by 1.
- Parameters:
-
value sigle byte
Reimplemented from BufferedArray.
Definition at line 51 of file APIFrame.cpp.
void set | ( | unsigned long | position, |
unsigned char | value | ||
) |
Write 8-bit data into specific posiston and deos not affect the current position.
- Parameters:
-
position where to write value sigle byte
Reimplemented from BufferedArray.
Definition at line 63 of file APIFrame.cpp.
void setCheckSum | ( | unsigned char | value ) |
void setPosition | ( | unsigned long | position ) | [inherited] |
Set the index within the max length of raw data.
- Parameters:
-
position where to begin read and write
Definition at line 49 of file BufferedArray.cpp.
void sets | ( | const unsigned char * | value, |
unsigned long | offset, | ||
unsigned long | length | ||
) |
Write array of data into current posiston, and increase by the lenght.
- Parameters:
-
value array of byte offset start point of the data length length to write
Reimplemented from BufferedArray.
Definition at line 57 of file APIFrame.cpp.
void sets | ( | unsigned long | position, |
const unsigned char * | value, | ||
unsigned long | offset, | ||
unsigned long | length | ||
) |
Write array of data into specific posiston and deos not affect the current position.
- Parameters:
-
position where to write value array of byte offset start point of the data length length to write
Reimplemented from BufferedArray.
Definition at line 69 of file APIFrame.cpp.
bool verifyChecksum | ( | ) |
Check is the API frame's checksum is verified.
- Returns:
- true checksum match, false checksum not match
Definition at line 85 of file APIFrame.cpp.
Field Documentation
unsigned char* data [protected, inherited] |
Raw data.
Definition at line 16 of file BufferedArray.h.
unsigned int expandSize [protected, inherited] |
initial size and automatically increase length.
Definition at line 13 of file BufferedArray.h.
unsigned long index [protected, inherited] |
Current index of the data, could also used as data lendth.
Definition at line 19 of file BufferedArray.h.
unsigned long max [protected, inherited] |
Max data size that the raw data can hold.
Definition at line 22 of file BufferedArray.h.
Generated on Tue Jul 12 2022 11:17:05 by
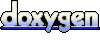