XBee API operation library for mbed
Embed:
(wiki syntax)
Show/hide line numbers
XBeeAPI.h
00001 #ifndef UK_AC_HERTS_SMARTLAB_XBEE_API 00002 #define UK_AC_HERTS_SMARTLAB_XBEE_API 00003 00004 #include "mbed.h" 00005 #include "SerialData.h" 00006 #include "CoreAPI.h" 00007 00008 /** XBee API library entry poiont. 00009 * Example: 00010 * @code 00011 * #include "mbed.h" 00012 * #include "XBeeAPI.h" 00013 * 00014 * XBeeAPI xbee(p9, p10, true); 00015 * Address add(0x0013A200,0x406CABD,0x0456); 00016 * XBeeTx16Request tx16(0x00, &add, OptionsBase::DEFAULT, "Hello From XBEE API", 0, 19); 00017 * XBeeRx16Indicator rx16(NULL); 00018 * 00019 * int main() 00020 * { 00021 * xbee.setVerifyChecksum(false); 00022 * 00023 * // option 1 00024 * while(1) { 00025 * XBeeRx16Indicator * response = xbee.getXBeeRx16(); 00026 * if (response != NULL) { 00027 * tx16.setPayload(response->getReceivedData(), 0, response->getReceivedDataLength()); 00028 * xbee.send(&tx16); 00029 * } 00030 * } 00031 * 00032 * // option 2 00033 * while(1) { 00034 * 00035 * APIFrame * frame = xbee.getResponse(); 00036 * while(frame != NULL) { 00037 * switch (frame->getFrameType()) { 00038 * case APIFrame::Tx64_Request : 00039 * break; 00040 * case APIFrame::Tx16_Request : 00041 * break; 00042 * case APIFrame::AT_Command: 00043 * break; 00044 * case APIFrame::AT_Command_Queue_Parameter_Value: 00045 * break; 00046 * case APIFrame::ZigBee_Transmit_Request: 00047 * break; 00048 * case APIFrame::Explicit_Addressing_ZigBee_Command_Frame: 00049 * break; 00050 * case APIFrame::Remote_Command_Request : 00051 * break; 00052 * case APIFrame::Create_Source_Route : 00053 * break; 00054 * case APIFrame::Register_Joining_Device: 00055 * break; 00056 * case APIFrame::Rx64_Receive_Packet : 00057 * break; 00058 * case APIFrame::Rx16_Receive_Packet: 00059 * rx16.convert(frame); 00060 * tx16.setPayload(rx16.getReceivedData(), 0, rx16.getReceivedDataLength()); 00061 * xbee.send(&tx16); 00062 * break; 00063 * case APIFrame::Rx64_IO_Data_Sample_Rx_Indicator: 00064 * break; 00065 * case APIFrame::Rx16_IO_Data_Sample_Rx_Indicator : 00066 * xbee.send(&tx16); 00067 * break; 00068 * case APIFrame::AT_Command_Response: 00069 * break; 00070 * case APIFrame::XBee_Transmit_Status : 00071 * break; 00072 * case APIFrame::Modem_Status: 00073 * break; 00074 * case APIFrame::ZigBee_Transmit_Status : 00075 * break; 00076 * case APIFrame::ZigBee_Receive_Packet: 00077 * break; 00078 * case APIFrame::ZigBee_Explicit_Rx_Indicator : 00079 * break; 00080 * case APIFrame::ZigBee_IO_Data_Sample_Rx_Indicator : 00081 * break; 00082 * case APIFrame::XBee_Sensor_Read_Indicato : 00083 * break; 00084 * case APIFrame::Node_Identification_Indicator: 00085 * break; 00086 * case APIFrame::Remote_Command_Response: 00087 * break; 00088 * case APIFrame::Over_the_Air_Firmware_Update_Status : 00089 * break; 00090 * case APIFrame::Route_Record_Indicator : 00091 * break; 00092 * case APIFrame::Device_Authenticated_Indicator: 00093 * break; 00094 * case APIFrame::Many_to_One_Route_Request_Indicator: 00095 * break; 00096 * } 00097 * 00098 * frame = xbee.getResponse(); 00099 * } 00100 * } 00101 * } 00102 * @endcode 00103 */ 00104 class XBeeAPI: public CoreAPI 00105 { 00106 public: 00107 00108 /** Create a XBeeAPI instance, with baud rate 9600 and no escape. 00109 * 00110 * @param tx data transmission line 00111 * @param rx data receiving line 00112 * 00113 */ 00114 XBeeAPI(PinName tx, PinName rx); 00115 00116 /** Create a XBeeAPI instance, with baud rate 9600. 00117 * 00118 * @param tx data transmission line 00119 * @param rx data receiving line 00120 * @param isEscape API escaped operating mode (AP = 2) works similarly to API mode. The only difference is that when working in API escaped mode, some bytes of the API frame specific data must be escaped. 00121 * 00122 */ 00123 XBeeAPI(PinName tx, PinName rx, bool isEscape); 00124 00125 /** Create a XBeeAPI instance. 00126 * 00127 * @param tx data transmission line 00128 * @param rx data receiving line 00129 * @param baudRate baud rate 00130 * @param isEscape API escaped operating mode (AP = 2) works similarly to API mode. The only difference is that when working in API escaped mode, some bytes of the API frame specific data must be escaped. 00131 * 00132 */ 00133 XBeeAPI(PinName tx, PinName rx, int baudRate, bool isEscape); 00134 00135 /** Create a SerialData instance with other serial interface. 00136 * 00137 * @param serial class that implementes ISerial interface. 00138 * @param baudRate baud rate 00139 * @param isEscape API escaped operating mode (AP = 2) works similarly to API mode. The only difference is that when working in API escaped mode, some bytes of the API frame specific data must be escaped. 00140 * 00141 */ 00142 XBeeAPI(ISerial * serial, bool isEscape); 00143 }; 00144 00145 #endif
Generated on Tue Jul 12 2022 11:17:05 by
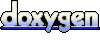