XBee API operation library for mbed
Embed:
(wiki syntax)
Show/hide line numbers
BufferedArray.h
00001 #ifndef UK_AC_HERTS_SMARTLAB_XBEE_BufferedArray 00002 #define UK_AC_HERTS_SMARTLAB_XBEE_BufferedArray 00003 00004 #include "mbed.h" 00005 00006 /** 00007 * Represent a generic, dynamic-length raw binary data buffer. 00008 */ 00009 class BufferedArray 00010 { 00011 protected : 00012 /// initial size and automatically increase length. 00013 unsigned int expandSize; 00014 00015 /// Raw data 00016 unsigned char * data; 00017 00018 /// Current index of the data, could also used as data lendth. 00019 unsigned long index; 00020 00021 /// Max data size that the raw data can hold. 00022 unsigned long max; 00023 00024 void expandSpace(unsigned long length); 00025 00026 public: 00027 BufferedArray(unsigned int initialLength, unsigned int expandSize); 00028 00029 BufferedArray(BufferedArray * bufferedArray); 00030 00031 ~BufferedArray(); 00032 00033 /** Get the raw data. 00034 * @returns unsigned char array. 00035 */ 00036 unsigned char * gets(); 00037 00038 /** Get the raw data from a specific location. 00039 * 00040 * @param position where to retrieve 00041 * 00042 * @returns unsigned char array. 00043 */ 00044 unsigned char * gets(unsigned long position); 00045 00046 /** Get 1 byte data from a specific location. 00047 * 00048 * @param position where to retrieve 00049 * 00050 * @returns unsigned char. 00051 */ 00052 unsigned char get(unsigned long position); 00053 00054 /** Get the current index. 00055 * @returns unsigned char array. 00056 */ 00057 int getPosition(); 00058 00059 /** Set the index within the max length of raw data. 00060 * @param position where to begin read and write 00061 */ 00062 void setPosition(unsigned long position); 00063 00064 /** Reset the raw data. 00065 * @param length current max size for the raw data 00066 */ 00067 void allocate(unsigned long length); 00068 00069 /** Reset the position and does not affect the content of the data. 00070 * @param length current max size for the raw data 00071 */ 00072 void rewind(); 00073 00074 /** Write 8-bit data into current posiston and increase by 1. 00075 * @param value sigle byte 00076 */ 00077 void set(unsigned char value); 00078 00079 /** Write array of data into current posiston, and increase by the lenght. 00080 * @param value array of byte 00081 * @param offset start point of the data 00082 * @param length length to write 00083 */ 00084 void sets(const unsigned char * value, unsigned long offset, unsigned long length); 00085 00086 /** Write 8-bit data into specific posiston and deos not affect the current position. 00087 * @param position where to write 00088 * @param value sigle byte 00089 */ 00090 void set(unsigned long position, unsigned char value); 00091 00092 /** Write array of data into specific posiston and deos not affect the current position. 00093 * @param position where to write 00094 * @param value array of byte 00095 * @param offset start point of the data 00096 * @param length length to write 00097 */ 00098 void sets(unsigned long position, const unsigned char * value, unsigned long offset, unsigned long length); 00099 }; 00100 00101 #endif
Generated on Tue Jul 12 2022 11:17:04 by
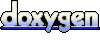