XBee API operation library for mbed
Embed:
(wiki syntax)
Show/hide line numbers
BufferedArray.cpp
00001 #include "BufferedArray.h" 00002 00003 BufferedArray::BufferedArray(unsigned int initialLength, unsigned int expandSize) 00004 { 00005 this->expandSize = expandSize; 00006 max = initialLength; 00007 data = new unsigned char[initialLength]; 00008 index = 0; 00009 } 00010 00011 BufferedArray::BufferedArray(BufferedArray * bufferedArray) 00012 { 00013 if (bufferedArray != NULL) { 00014 this->data = bufferedArray->data; 00015 this->index = bufferedArray->index; 00016 this->max = bufferedArray->max; 00017 this->expandSize = bufferedArray->expandSize; 00018 } 00019 } 00020 00021 BufferedArray::~BufferedArray() 00022 { 00023 if (data == NULL) 00024 return; 00025 00026 delete[] data; 00027 } 00028 00029 unsigned char * BufferedArray::gets() 00030 { 00031 return data; 00032 } 00033 00034 unsigned char * BufferedArray::gets(unsigned long position) 00035 { 00036 return data + position; 00037 } 00038 00039 unsigned char BufferedArray::get(unsigned long position) 00040 { 00041 return *(data + position); 00042 } 00043 00044 int BufferedArray::getPosition() 00045 { 00046 return index; 00047 } 00048 00049 void BufferedArray::setPosition(unsigned long position) 00050 { 00051 if (this->index > max) 00052 this->index = max; 00053 else this->index = position; 00054 } 00055 00056 void BufferedArray::allocate(unsigned long length) 00057 { 00058 if (length <= 0) 00059 return; 00060 00061 if (length > max) { 00062 if (data != NULL) 00063 delete[] data; 00064 data = new unsigned char[length]; 00065 } 00066 00067 rewind(); 00068 } 00069 00070 void BufferedArray::rewind() 00071 { 00072 index = 0; 00073 } 00074 00075 void BufferedArray::expandSpace(unsigned long length) 00076 { 00077 max += expandSize * (1 + length / expandSize); 00078 unsigned char * temp = new unsigned char[max]; 00079 memcpy(temp, data, index); 00080 delete[] data; 00081 data = temp; 00082 } 00083 00084 void BufferedArray::set(unsigned long position, unsigned char value) 00085 { 00086 if (position >= max) 00087 expandSpace(position - max + 1); 00088 00089 data[position] = value; 00090 } 00091 00092 void BufferedArray::set(unsigned char value) 00093 { 00094 set(index, value); 00095 index++; 00096 } 00097 00098 void BufferedArray::sets(const unsigned char * value, unsigned long offset, unsigned long length) 00099 { 00100 if (length <= 0) 00101 return; 00102 00103 sets(index, value, offset, length); 00104 index += length; 00105 } 00106 00107 void BufferedArray::sets(unsigned long position, const unsigned char * value, unsigned long offset, unsigned long length) 00108 { 00109 if (length <= 0) 00110 return; 00111 00112 if (position + length > max) 00113 expandSpace(position + length - max); 00114 00115 memcpy(data + position, value, length); 00116 }
Generated on Tue Jul 12 2022 11:17:04 by
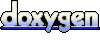