LED Driver, 6 digits @ 8 segm, 8 LEDs, 16 Keys. SPI Interface
STLED316S_BOARD Class Reference
Constructor for class for driving STM STLED316S controller as used in ST316S test display. More...
#include <STLED316S.h>
Inherits STLED316S.
Public Types | |
enum | Icon |
Enums for LEDs. More... | |
enum | Mode |
Enums for Display mode. More... | |
enum | BrightMode |
Enums for Brightness mode. More... | |
typedef char | DisplayData_t [STLED316S_DISPLAY_MEM] |
Datatypes for display and keymatrix data. | |
Public Member Functions | |
STLED316S_BOARD (PinName mosi, PinName miso, PinName sclk, PinName cs) | |
Constructor for class for driving STLED316S controller as used in ST316S test display. | |
int | putc (int c) |
Write a character to the Display. | |
int | printf (const char *format,...) |
Write a formatted string to the Display. | |
void | locate (int column) |
Locate cursor to a screen column. | |
void | cls (bool clrAll=false) |
Clear the screen and locate to 0. | |
void | setIcon (Icon icon) |
Set Icon. | |
void | clrIcon (Icon icon) |
Clr Icon. | |
void | setUDC (unsigned char udc_idx, int udc_data) |
Set User Defined Characters (UDC) | |
int | columns () |
Number of screen columns. | |
void | writeData (int address, char data) |
Write databyte to STLED316S. | |
void | writeData (DisplayData_t data, int length=(ST316BOARD_NR_GRIDS)) |
Write Display datablock to STLED316S. | |
void | cls () |
Clear the screen and locate to 0. | |
void | writeLedData (LedData_t leds) |
Write LED data to STLED316S. | |
void | setLed (LedData_t leds) |
Set LED. | |
void | clrLed (LedData_t leds) |
Clr LED. | |
bool | getKeys (KeyData_t *keydata) |
Read keydata block from STLED316S. | |
void | setBrightness (char brightness=STLED316S_BRT_DEF) |
Set Brightness for all Digits and LEDs (value is used in GlobalBrightness mode) | |
void | setLedBrightness (LedData_t leds=STLED316S_LED_ALL, char led_brt=STLED316S_BRT_DEF) |
Set Individual LED Brightness (value is used in IndivBright mode) | |
void | setDigitBrightness (LedData_t digits=STLED316S_DIG_ALL, char dig_brt=STLED316S_BRT_DEF) |
Set Individual Digit Brightness (value is used in IndivBright mode) | |
void | setBrightMode (BrightMode brt_mode=GlobalBright) |
Set Brightness mode. | |
void | setDisplay (bool on) |
Set the Display mode On/off. | |
Protected Member Functions | |
virtual int | _putc (int value) |
Write a single character (Stream implementation) |
Detailed Description
Constructor for class for driving STM STLED316S controller as used in ST316S test display.
Supports 6 Digits of 8 Segments, 1 Grid of 3 LEDs. Also supports a scanned keyboard of 3 keys.
- Parameters:
-
PinName mosi, miso, sclk, cs SPI bus pins
Definition at line 392 of file STLED316S.h.
Member Typedef Documentation
typedef char DisplayData_t[STLED316S_DISPLAY_MEM] [inherited] |
Datatypes for display and keymatrix data.
Definition at line 233 of file STLED316S.h.
Member Enumeration Documentation
enum BrightMode [inherited] |
Enums for Brightness mode.
Definition at line 227 of file STLED316S.h.
enum Icon |
Enums for LEDs.
Definition at line 397 of file STLED316S.h.
enum Mode [inherited] |
Enums for Display mode.
Definition at line 217 of file STLED316S.h.
Constructor & Destructor Documentation
STLED316S_BOARD | ( | PinName | mosi, |
PinName | miso, | ||
PinName | sclk, | ||
PinName | cs | ||
) |
Constructor for class for driving STLED316S controller as used in ST316S test display.
Constructor for class for driving STM STLED316S controller as used in ST316S test display.
Supports 6 Digits of 8 Segments, 1 Grid of 3 LEDs. Also supports a scanned keyboard of 3 keys.
- Parameters:
-
PinName mosi, miso, sclk, cs SPI bus pins
Supports 6 Digits of 7 Segments and 3 LEDs. Also supports a scanned keyboard of 3.
- Parameters:
-
PinName mosi, miso, sclk, cs SPI bus pins
Definition at line 456 of file STLED316S.cpp.
Member Function Documentation
int _putc | ( | int | value ) | [protected, virtual] |
Write a single character (Stream implementation)
Definition at line 571 of file STLED316S.cpp.
void clrIcon | ( | Icon | icon ) |
Clr Icon.
- Parameters:
-
Icon icon Enums Icon has Grid position encoded in 8 MSBs, Icon pattern encoded in 16 LSBs
- Returns:
- none
Definition at line 541 of file STLED316S.cpp.
void clrLed | ( | LedData_t | leds ) | [inherited] |
Clr LED.
- Parameters:
-
LedData_t leds pattern of LED data
- Returns:
- none
Definition at line 314 of file STLED316S.cpp.
void cls | ( | bool | clrAll = false ) |
Clear the screen and locate to 0.
- Parameters:
-
bool clrAll Clear Icons also (default = false)
Definition at line 504 of file STLED316S.cpp.
void cls | ( | ) | [inherited] |
Clear the screen and locate to 0.
Definition at line 68 of file STLED316S.cpp.
int columns | ( | ) |
Number of screen columns.
- Parameters:
-
none
- Returns:
- columns
Definition at line 496 of file STLED316S.cpp.
bool getKeys | ( | KeyData_t * | keydata ) | [inherited] |
Read keydata block from STLED316S.
- Parameters:
-
*keydata Ptr to Array of STLED316S_KEY_MEM (=2) bytes for keydata
- Returns:
- bool keypress True when at least one key was pressed
Note: Due to the hardware configuration the STLED316S key matrix scanner will detect multiple keys pressed at same time, but this may result in some spurious keys also being set in keypress data array. It may be best to ignore all keys in those situations. That option is implemented in this method depending on define setting.
- Parameters:
-
*keydata Ptr to Array of STLED316S_KEY_MEM (=5) bytes for keydata
- Returns:
- bool keypress True when at least one key was pressed
Note: Due to the hardware configuration the STLED316S key matrix scanner will detect multiple keys pressed at same time, but this may also result in some spurious keys being set in keypress data array. It may be best to ignore all keys in those situations. That option is implemented in this method depending on define setting.
Definition at line 327 of file STLED316S.cpp.
void locate | ( | int | column ) |
Locate cursor to a screen column.
- Parameters:
-
column The horizontal position from the left, indexed from 0
Definition at line 482 of file STLED316S.cpp.
int printf | ( | const char * | format, |
... | |||
) |
Write a formatted string to the Display.
- Parameters:
-
format A printf-style format string, followed by the variables to use in formatting the string.
int putc | ( | int | c ) |
Write a character to the Display.
- Parameters:
-
c The character to write to the display
void setBrightMode | ( | BrightMode | brt_mode = GlobalBright ) |
[inherited] |
Set Brightness mode.
- Parameters:
-
BrightMode brt_mode (value is IndivBright or GlobalBright)
- Returns:
- none
Definition at line 218 of file STLED316S.cpp.
void setBrightness | ( | char | brightness = STLED316S_BRT_DEF ) |
[inherited] |
Set Brightness for all Digits and LEDs (value is used in GlobalBrightness mode)
Set Brightness for all Digits and LEDs (value is used in GlobalBright mode)
- Parameters:
-
char brightness (3 significant bits, valid range 0..7 (1/16 .. 14/14 dutycycle)
- Returns:
- none
Definition at line 87 of file STLED316S.cpp.
void setDigitBrightness | ( | LedData_t | digits = STLED316S_DIG_ALL , |
char | dig_brt = STLED316S_BRT_DEF |
||
) | [inherited] |
Set Individual Digit Brightness (value is used in IndivBright mode)
- Parameters:
-
LedData_t digits pattern of Digit data char dig_brt (3 significant bits, valid range 0..7 (1/16 .. 14/14 dutycycle)
- Returns:
- none
Definition at line 161 of file STLED316S.cpp.
void setDisplay | ( | bool | on ) | [inherited] |
Set the Display mode On/off.
- Parameters:
-
bool display mode
Definition at line 203 of file STLED316S.cpp.
void setIcon | ( | Icon | icon ) |
Set Icon.
- Parameters:
-
Icon icon Enums Icon has Grid position encoded in 8 MSBs, Icon pattern encoded in 16 LSBs
- Returns:
- none
Definition at line 526 of file STLED316S.cpp.
void setLed | ( | LedData_t | leds ) | [inherited] |
Set LED.
- Parameters:
-
LedData_t leds pattern of LED data
- Returns:
- none
Definition at line 303 of file STLED316S.cpp.
void setLedBrightness | ( | LedData_t | leds = STLED316S_LED_ALL , |
char | led_brt = STLED316S_BRT_DEF |
||
) | [inherited] |
Set Individual LED Brightness (value is used in IndivBright mode)
- Parameters:
-
LedData_t leds pattern of LED data char led_brt (3 significant bits, valid range 0..7 (1/16 .. 14/14 dutycycle)
- Returns:
- none
Definition at line 107 of file STLED316S.cpp.
void setUDC | ( | unsigned char | udc_idx, |
int | udc_data | ||
) |
Set User Defined Characters (UDC)
- Parameters:
-
unsigned char udc_idx The Index of the UDC (0..7) int udc_data The bitpattern for the UDC (16 bits)
Definition at line 557 of file STLED316S.cpp.
void writeData | ( | int | address, |
char | data | ||
) |
Write databyte to STLED316S.
- Parameters:
-
int address display memory location to write byte char data byte written at given address
- Returns:
- none
Reimplemented from STLED316S.
Definition at line 473 of file STLED316S.h.
void writeData | ( | DisplayData_t | data, |
int | length = (ST316BOARD_NR_GRIDS) |
||
) |
Write Display datablock to STLED316S.
- Parameters:
-
DisplayData_t data Array of STLED316S_DISPLAY_MEM (=6) bytes for displaydata (starting at address 0) length number bytes to write (valid range 0..(ST316BOARD_NR_GRIDS) (=6), starting at address 0)
- Returns:
- none
Reimplemented from STLED316S.
Definition at line 482 of file STLED316S.h.
void writeLedData | ( | LedData_t | leds ) | [inherited] |
Write LED data to STLED316S.
- Parameters:
-
LedData_t leds LED data
- Returns:
- none
Definition at line 281 of file STLED316S.cpp.
Generated on Sat Jul 16 2022 20:44:27 by
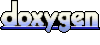