LED Driver, 6 digits @ 8 segm, 8 LEDs, 16 Keys. SPI Interface
Embed:
(wiki syntax)
Show/hide line numbers
STLED316S.h
00001 /* mbed STLED316S Library, for STLED316S LED controller 00002 * Copyright (c) 2016, v01: WH, Initial version 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 #ifndef STLED316S_H 00024 #define STLED316S_H 00025 00026 // Select one of the testboards for the STM STLED316S LED controller 00027 #include "STLED316S_Config.h" 00028 00029 #include "Font_7Seg.h" 00030 00031 /** An interface for driving STLED316S LED controller 00032 * 00033 * @code 00034 * #include "mbed.h" 00035 * #include "STLED316S.h" 00036 * 00037 * DisplayData_t size is 1 bytes (1 grid @ 8 segments) thru 6 bytes (6 grids @ 8 segments) 00038 * STLED316S::DisplayData_t all_str = {0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF}; 00039 * STLED316S::DisplayData_t bye_str = {LO(C7_B), LO(C7_Y), LO(C7_E), 0x00, 0x00, 0x00}; 00040 * STLED316S::LedData_t all_led = {0xFF}; 00041 * 00042 * // KeyData_t size is 2 bytes 00043 * STLED316S::KeyData_t keydata; 00044 * 00045 * // STLED316S declaration, Default setting 6 Grids @ 8 Segments 00046 * STLED316S STLED316S(p5,p6,p7, p8); 00047 * 00048 * int main() { 00049 * 00050 * STLED316S.cls(); 00051 * STLED316S.writeData(all_str); 00052 * STLED316S.writeLedData(all_led); 00053 * wait(2); 00054 * 00055 * STLED316S.setBrightMode(STLED316S::GlobalBright); 00056 * STLED316S.setBrightness(STLED316S_BRT3); 00057 * wait(1); 00058 * STLED316S.setBrightness(STLED316S_BRT0); 00059 * wait(1); 00060 * STLED316S.setBrightness(STLED316S_BRT3); 00061 * 00062 * while (1) { 00063 * // Check and read keydata 00064 * if (STLED316S.getKeys(&keydata)) { 00065 * //pc.printf("Keydata 0..1 = 0x%02x 0x%02x\r\n", keydata[0], keydata[1]); 00066 * 00067 * if (keydata[0] == 0x01) { // Key1&KS1 00068 * wait(1); 00069 * STLED316S.setLed(STLED316S_LED_L1); 00070 * wait(1); 00071 * STLED316S.clrLed(STLED316S_LED_L1); 00072 * } 00073 * } 00074 * } 00075 * } 00076 * @endcode 00077 */ 00078 00079 //STLED316S Display and Keymatrix data 00080 #define STLED316S_MAX_NR_GRIDS 6 00081 #define STLED316S_BYTES_PER_GRID 1 00082 //Significant bits Keymatrix data 00083 #define STLED316S_KEY_MSK 0xFF 00084 00085 //Memory size in bytes for Display and Keymatrix 00086 #define STLED316S_DISPLAY_MEM (STLED316S_MAX_NR_GRIDS * STLED316S_BYTES_PER_GRID) 00087 #define STLED316S_KEY_MEM 2 00088 00089 //Address set commands 00090 #define STLED316S_CMD_MSK 0x7F 00091 #define STLED316S_DATA_WR 0x00 00092 #define STLED316S_DATA_RD 0x40 00093 #define STLED316S_ADDR_INC 0x00 00094 #define STLED316S_ADDR_FIXED 0x20 00095 #define STLED316S_PAGE_MSK 0x18 00096 #define STLED316S_PAGE_SHFT 3 00097 #define STLED316S_ADDR_MSK 0x07 00098 #define STLED316S_ADDR_SHFT 0 00099 #define STLED316S_IDX_MSK (STLED316S_PAGE_MSK | STLED316S_ADDR_MSK) 00100 00101 //Read and Write commands 00102 #define STLED316S_ADDR_WR_CMD (STLED316S_DATA_WR | STLED316S_ADDR_INC) 00103 #define STLED316S_ADDR_RD_CMD (STLED316S_DATA_RD | STLED316S_ADDR_INC) 00104 00105 //Combined Page and Address parameters 00106 #define STLED316S_IDX(page, addr) ( ((page << STLED316S_PAGE_SHFT) & STLED316S_PAGE_MSK) | ((addr << STLED316S_ADDR_SHFT) & STLED316S_ADDR_MSK) ) 00107 00108 00109 //Digit Data address (Digit2..Digit7) 00110 #define STLED316S_DIG_PAGE 0 00111 #define STLED316S_DIG2_ADDR 0x00 00112 #define STLED316S_DIG3_ADDR 0x01 00113 #define STLED316S_DIG4_ADDR 0x02 00114 #define STLED316S_DIG5_ADDR 0x03 00115 #define STLED316S_DIG6_ADDR 0x04 00116 #define STLED316S_DIG7_ADDR 0x05 00117 00118 //LED Data address (also Digit1 Data address) 00119 #define STLED316S_DIG1_LED_PAGE 1 00120 #define STLED316S_DIG1_LED_ADDR 0x00 00121 00122 //LED Data used to select individual LEDs 00123 #define STLED316S_LED_L1 0x01 00124 #define STLED316S_LED_L2 0x02 00125 #define STLED316S_LED_L3 0x04 00126 #define STLED316S_LED_L4 0x08 00127 #define STLED316S_LED_L5 0x10 00128 #define STLED316S_LED_L6 0x20 00129 #define STLED316S_LED_L7 0x40 00130 #define STLED316S_LED_L8 0x80 00131 #define STLED316S_LED_ALL 0xFF 00132 00133 //Digit Data used to select individual digits 00134 //#define STLED316S_DIG_D1 0x01 00135 #define STLED316S_DIG_D2 0x02 00136 #define STLED316S_DIG_D3 0x04 00137 #define STLED316S_DIG_D4 0x08 00138 #define STLED316S_DIG_D5 0x10 00139 #define STLED316S_DIG_D6 0x20 00140 #define STLED316S_DIG_D7 0x40 00141 #define STLED316S_DIG_ALL 0x7E 00142 00143 00144 //Key Data address 00145 #define STLED316S_KEY_PAGE 1 00146 #define STLED316S_KEY1_ADDR 0x01 00147 #define STLED316S_KEY2_ADDR 0x02 00148 00149 //Display On_Off address 00150 #define STLED316S_DSP_PAGE 1 00151 #define STLED316S_DSP_ON_ADDR 0x05 00152 #define STLED316S_DSP_OFF_ADDR 0x06 00153 00154 //Config set address 00155 #define STLED316S_CONF_PAGE 2 00156 #define STLED316S_CONF_ADDR 0x00 00157 00158 //Config parameters 00159 #define STLED316S_CONF_GRID_MSK 0x07 00160 #define STLED316S_CONF_GRID_SHFT 0 00161 #define STLED316S_CONF_BRT_MODE_MSK 0x18 00162 #define STLED316S_CONF_BRT_MODE_SHFT 3 00163 #define STLED316S_CONF_BRT_GLOB_MSK 0xE0 00164 #define STLED316S_CONF_BRT_GLOB_SHFT 5 00165 00166 //Grid Config parameters 00167 #define STLED316S_GR1_SEG8 0x00 00168 #define STLED316S_GR2_SEG8 0x01 00169 #define STLED316S_GR3_SEG8 0x02 00170 #define STLED316S_GR4_SEG8 0x03 //default 00171 #define STLED316S_GR5_SEG8 0x04 00172 #define STLED316S_GR6_SEG8 0x05 00173 00174 //Brightness Mode Config parameters 00175 #define STLED316S_BRT_INDIV 0x00 00176 #define STLED316S_BRT_GLOB 0x03 //default 00177 00178 //Digit Brightness address (used when Brightness mode is set as 'individual') 00179 #define STLED316S_DIG_BRT_PAGE 2 00180 #define STLED316S_DIG2_3_BRT_ADDR 0x01 00181 #define STLED316S_DIG4_5_BRT_ADDR 0x02 00182 #define STLED316S_DIG6_7_BRT_ADDR 0x03 00183 00184 //LED Brightness address (used when Brightness mode is set as 'individual') 00185 #define STLED316S_LED_BRT_PAGE 3 00186 #define STLED316S_LED1_2_BRT_ADDR 0x00 00187 #define STLED316S_LED3_4_BRT_ADDR 0x01 00188 #define STLED316S_LED5_6_BRT_ADDR 0x02 00189 #define STLED316S_LED7_8_BRT_ADDR 0x03 00190 00191 //Brightness control parameters (used for both Brightness modes 'global' and 'individual') 00192 #define STLED316S_BRT_MSK 0x07 00193 00194 #define STLED316S_BRT0 0x00 //Pulsewidth 1/16 00195 #define STLED316S_BRT1 0x01 00196 #define STLED316S_BRT2 0x02 00197 #define STLED316S_BRT3 0x03 00198 #define STLED316S_BRT4 0x04 00199 #define STLED316S_BRT5 0x05 00200 #define STLED316S_BRT6 0x06 00201 #define STLED316S_BRT7 0x07 //Pulsewidth 14/16 (default) 00202 00203 #define STLED316S_BRT_DEF STLED316S_BRT3 00204 00205 00206 00207 /** A class for driving STM STLED316S LED controller 00208 * 00209 * @brief Supports 1..6 Grids @ 8 Segments and 8 LEDs. 00210 * Also supports a scanned keyboard of upto 16 keys. 00211 * SPI bus interface device. 00212 */ 00213 class STLED316S { 00214 public: 00215 00216 /** Enums for Display mode */ 00217 enum Mode { 00218 Grid1_Seg8 = STLED316S_GR1_SEG8, 00219 Grid2_Seg8 = STLED316S_GR2_SEG8, 00220 Grid3_Seg8 = STLED316S_GR3_SEG8, 00221 Grid4_Seg8 = STLED316S_GR4_SEG8, 00222 Grid5_Seg8 = STLED316S_GR5_SEG8, 00223 Grid6_Seg8 = STLED316S_GR6_SEG8 00224 }; 00225 00226 /** Enums for Brightness mode */ 00227 enum BrightMode { 00228 IndivBright = STLED316S_BRT_INDIV, 00229 GlobalBright = STLED316S_BRT_GLOB 00230 }; 00231 00232 /** Datatypes for display and keymatrix data */ 00233 typedef char DisplayData_t[STLED316S_DISPLAY_MEM]; 00234 typedef char LedData_t; 00235 typedef char KeyData_t[STLED316S_KEY_MEM]; 00236 00237 /** Constructor for class for driving STLED316S LED controller 00238 * 00239 * @brief Supports 1..6 Grids @ 8 Segments and 8 LEDs. 00240 * Also supports a scanned keyboard of upto 16 keys. 00241 * SPI bus interface device. 00242 * 00243 * @param PinName mosi, miso, sclk, cs SPI bus pins 00244 * @param Mode selects either Grids/Segments (default 6 Grids @ 8 Segments) 00245 */ 00246 STLED316S(PinName mosi, PinName miso, PinName sclk, PinName cs, Mode mode=Grid6_Seg8); 00247 00248 /** Clear the screen and locate to 0 00249 */ 00250 void cls(); 00251 00252 /** Write databyte to STLED316S 00253 * @param int address display memory location to write byte 00254 * @param char data byte written at given address 00255 * @return none 00256 */ 00257 void writeData(int address, char data); 00258 00259 /** Write Display datablock to STLED316S 00260 * @param DisplayData_t data Array of STLED316S_DISPLAY_MEM (=6) bytes for displaydata (starting at address 0) 00261 * @param length number bytes to write (valid range 0..STLED316S_DISPLAY_MEM (=6), starting at address 0) 00262 * @return none 00263 */ 00264 void writeData(DisplayData_t data, int length = STLED316S_DISPLAY_MEM); 00265 00266 /** Write LED data to STLED316S 00267 * @param LedData_t leds LED data 00268 * @return none 00269 */ 00270 void writeLedData(LedData_t leds); 00271 00272 /** Set LED 00273 * 00274 * @param LedData_t leds pattern of LED data 00275 * @return none 00276 */ 00277 void setLed(LedData_t leds); 00278 00279 /** Clr LED 00280 * 00281 * @param LedData_t leds pattern of LED data 00282 * @return none 00283 */ 00284 void clrLed(LedData_t leds); 00285 00286 00287 /** Read keydata block from STLED316S 00288 * @param *keydata Ptr to Array of STLED316S_KEY_MEM (=2) bytes for keydata 00289 * @return bool keypress True when at least one key was pressed 00290 * 00291 * Note: Due to the hardware configuration the STLED316S key matrix scanner will detect multiple keys pressed at same time, 00292 * but this may result in some spurious keys also being set in keypress data array. 00293 * It may be best to ignore all keys in those situations. That option is implemented in this method depending on #define setting. 00294 */ 00295 bool getKeys(KeyData_t *keydata); 00296 00297 /** Set Brightness for all Digits and LEDs (value is used in GlobalBrightness mode) 00298 * 00299 * @param char brightness (3 significant bits, valid range 0..7 (1/16 .. 14/14 dutycycle) 00300 * @return none 00301 */ 00302 void setBrightness(char brightness = STLED316S_BRT_DEF); 00303 00304 /** Set Individual LED Brightness (value is used in IndivBright mode) 00305 * 00306 * @param LedData_t leds pattern of LED data 00307 * @param char led_brt (3 significant bits, valid range 0..7 (1/16 .. 14/14 dutycycle) 00308 * @return none 00309 */ 00310 void setLedBrightness(LedData_t leds = STLED316S_LED_ALL, char led_brt = STLED316S_BRT_DEF); 00311 00312 /** Set Individual Digit Brightness (value is used in IndivBright mode) 00313 * 00314 * @param LedData_t digits pattern of Digit data 00315 * @param char dig_brt (3 significant bits, valid range 0..7 (1/16 .. 14/14 dutycycle) 00316 * @return none 00317 */ 00318 void setDigitBrightness(LedData_t digits = STLED316S_DIG_ALL, char dig_brt = STLED316S_BRT_DEF); 00319 00320 /** Set Brightness mode 00321 * 00322 * @param BrightMode brt_mode (value is IndivBright or GlobalBright) 00323 * @return none 00324 */ 00325 void setBrightMode(BrightMode brt_mode = GlobalBright); 00326 00327 00328 /** Set the Display mode On/off 00329 * 00330 * @param bool display mode 00331 */ 00332 void setDisplay(bool on); 00333 00334 private: 00335 SPI _spi; 00336 DigitalOut _cs; 00337 Mode _mode; 00338 BrightMode _brt_mode; 00339 char _bright; 00340 LedData_t _leds; 00341 00342 /** Init the SPI interface and the controller 00343 * @param none 00344 * @return none 00345 */ 00346 void _init(); 00347 00348 /** Helper to reverse all command or databits. The STLED316S expects LSB first, whereas SPI is MSB first 00349 * @param char data 00350 * @return bitreversed data 00351 */ 00352 char _flip(char data); 00353 00354 /** Write parameter to STLED316S Register 00355 * @param int idx Register address 00356 * @param int data Parameter for Register 00357 * @return none 00358 */ 00359 void _writeReg(int idx, int data); 00360 00361 /** Write merged command and parameter to STLED316S 00362 * @param int cmd Command & Parameter byte 00363 * @return none 00364 */ 00365 void _writeReg(int cmd); 00366 00367 /** Read parameter from STLED316S Register 00368 * @param int idx Register address 00369 * @return char data from Register 00370 */ 00371 char _readReg(int idx); 00372 }; 00373 00374 00375 00376 #if(ST316BOARD_TEST == 1) 00377 // Derived class for STLED316S used in test display module 00378 // 00379 #include "Font_7Seg.h" 00380 00381 #define ST316BOARD_NR_GRIDS 6 00382 #define ST316BOARD_NR_DIGITS 6 00383 //#define ST316BOARD_DIG1_IDX 0 00384 #define ST316BOARD_NR_UDC 8 00385 00386 /** Constructor for class for driving STM STLED316S controller as used in ST316S test display 00387 * 00388 * @brief Supports 6 Digits of 8 Segments, 1 Grid of 3 LEDs. Also supports a scanned keyboard of 3 keys. 00389 * 00390 * @param PinName mosi, miso, sclk, cs SPI bus pins 00391 */ 00392 class STLED316S_BOARD : public STLED316S, public Stream { 00393 public: 00394 00395 /** Enums for LEDs */ 00396 // Grid encoded in 8 MSBs, LED pattern encoded in 16 LSBs 00397 enum Icon { 00398 LD1 = (0<<24) | STLED316S_LED_L1, 00399 LD2 = (0<<24) | STLED316S_LED_L2, 00400 LD3 = (0<<24) | STLED316S_LED_L3, 00401 }; 00402 00403 typedef short UDCData_t[ST316BOARD_NR_UDC]; 00404 00405 /** Constructor for class for driving STLED316S controller as used in ST316S test display 00406 * 00407 * @brief Supports 6 Digits of 8 Segments, 1 Grid of 3 LEDs. Also supports a scanned keyboard of 3 keys. 00408 * 00409 * @param PinName mosi, miso, sclk, cs SPI bus pins 00410 */ 00411 STLED316S_BOARD(PinName mosi, PinName miso, PinName sclk, PinName cs); 00412 00413 #if DOXYGEN_ONLY 00414 /** Write a character to the Display 00415 * 00416 * @param c The character to write to the display 00417 */ 00418 int putc(int c); 00419 00420 /** Write a formatted string to the Display 00421 * 00422 * @param format A printf-style format string, followed by the 00423 * variables to use in formatting the string. 00424 */ 00425 int printf(const char* format, ...); 00426 #endif 00427 00428 /** Locate cursor to a screen column 00429 * 00430 * @param column The horizontal position from the left, indexed from 0 00431 */ 00432 void locate(int column); 00433 00434 /** Clear the screen and locate to 0 00435 * @param bool clrAll Clear Icons also (default = false) 00436 */ 00437 void cls(bool clrAll = false); 00438 00439 /** Set Icon 00440 * 00441 * @param Icon icon Enums Icon has Grid position encoded in 8 MSBs, Icon pattern encoded in 16 LSBs 00442 * @return none 00443 */ 00444 void setIcon(Icon icon); 00445 00446 /** Clr Icon 00447 * 00448 * @param Icon icon Enums Icon has Grid position encoded in 8 MSBs, Icon pattern encoded in 16 LSBs 00449 * @return none 00450 */ 00451 void clrIcon(Icon icon); 00452 00453 /** Set User Defined Characters (UDC) 00454 * 00455 * @param unsigned char udc_idx The Index of the UDC (0..7) 00456 * @param int udc_data The bitpattern for the UDC (16 bits) 00457 */ 00458 void setUDC(unsigned char udc_idx, int udc_data); 00459 00460 00461 /** Number of screen columns 00462 * 00463 * @param none 00464 * @return columns 00465 */ 00466 int columns(); 00467 00468 /** Write databyte to STLED316S 00469 * @param int address display memory location to write byte 00470 * @param char data byte written at given address 00471 * @return none 00472 */ 00473 void writeData(int address, char data){ 00474 STLED316S::writeData(address, data); 00475 } 00476 00477 /** Write Display datablock to STLED316S 00478 * @param DisplayData_t data Array of STLED316S_DISPLAY_MEM (=6) bytes for displaydata (starting at address 0) 00479 * @param length number bytes to write (valid range 0..(ST316BOARD_NR_GRIDS) (=6), starting at address 0) 00480 * @return none 00481 */ 00482 void writeData(DisplayData_t data, int length = (ST316BOARD_NR_GRIDS)) { 00483 STLED316S::writeData(data, length); 00484 } 00485 00486 protected: 00487 // Stream implementation functions 00488 virtual int _putc(int value); 00489 virtual int _getc(); 00490 00491 private: 00492 int _column; 00493 int _columns; 00494 00495 DisplayData_t _displaybuffer; 00496 UDCData_t _UDC_7S; 00497 }; 00498 #endif 00499 00500 00501 #endif
Generated on Sat Jul 16 2022 20:44:27 by
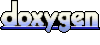