LED Driver, 6 digits @ 8 segm, 8 LEDs, 16 Keys. SPI Interface
Embed:
(wiki syntax)
Show/hide line numbers
STLED316S.cpp
00001 /* mbed STLED316S Library, for STLED316S LED controller 00002 * Copyright (c) 2016, v01: WH, Initial version 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 #include "mbed.h" 00023 #include "STLED316S.h" 00024 #include "Font_7Seg.h" 00025 00026 /** Constructor for class for driving STLED316S LED controller with SPI bus interface device. 00027 * @brief Supports 1..6 digits @ 8 segments and 8 LEDs. 00028 * Also supports a scanned keyboard of upto 16 keys. 00029 * 00030 * @param PinName mosi, miso, sclk, cs SPI bus pins 00031 * @param Mode selects 1..6 Digits of 8 Segments (default 6 Digits of 8 Segments) 00032 */ 00033 STLED316S::STLED316S(PinName mosi, PinName miso, PinName sclk, PinName cs, Mode mode) : _spi(mosi,miso,sclk), _cs(cs), _mode(mode) { 00034 00035 _init(); 00036 } 00037 00038 /** Init the SPI interface and the controller 00039 * @param none 00040 * @return none 00041 */ 00042 void STLED316S::_init(){ 00043 char config; 00044 00045 //init SPI 00046 _cs=1; 00047 _spi.format(8,3); //STLED316S uses mode 3 (Clock High on Idle, Data latched on second (=rising) edge) 00048 _spi.frequency(500000); 00049 00050 //init controller 00051 _brt_mode = GlobalBright; //STLED316S_BRT_GLOB; 00052 // _brt_mode = IndivBright; //STLED316S_BRT_INDIV; 00053 _bright = STLED316S_BRT_DEF; 00054 00055 config = ((_mode << STLED316S_CONF_GRID_SHFT) & STLED316S_CONF_GRID_MSK) | 00056 ((_brt_mode << STLED316S_CONF_BRT_MODE_SHFT) & STLED316S_CONF_BRT_MODE_MSK) | 00057 ((_bright << STLED316S_CONF_BRT_GLOB_SHFT) & STLED316S_CONF_BRT_GLOB_MSK); 00058 00059 _writeReg(STLED316S_IDX(STLED316S_CONF_PAGE,STLED316S_CONF_ADDR), config); // Config set command 00060 // printf("Cmd= 0x%02X, Conf= 0x%02X\r\n", STLED316S_ADDR_WR_CMD | STLED316S_IDX(STLED316S_CONF_PAGE,STLED316S_CONF_ADDR), config); // Debug 00061 00062 _writeReg(STLED316S_IDX(STLED316S_DSP_PAGE,STLED316S_DSP_ON_ADDR)); // Display On command 00063 } 00064 00065 00066 /** Clear the screen and locate to 0 00067 */ 00068 void STLED316S::cls() { 00069 00070 _cs=0; 00071 wait_us(1); 00072 _spi.write(_flip(STLED316S_ADDR_WR_CMD | STLED316S_IDX(STLED316S_DIG_PAGE,STLED316S_DIG2_ADDR))); // Address set cmd, 0 00073 00074 for (int cnt=0; cnt<STLED316S_DISPLAY_MEM; cnt++) { 00075 _spi.write(0x00); // data 00076 } 00077 00078 wait_us(1); 00079 _cs=1; 00080 } 00081 00082 /** Set Brightness for all Digits and LEDs (value is used in GlobalBright mode) 00083 * 00084 * @param char brightness (3 significant bits, valid range 0..7 (1/16 .. 14/14 dutycycle) 00085 * @return none 00086 */ 00087 void STLED316S::setBrightness(char brightness){ 00088 char config; 00089 00090 _bright = brightness & STLED316S_BRT_MSK; // mask invalid bits 00091 00092 config = ((_mode << STLED316S_CONF_GRID_SHFT) & STLED316S_CONF_GRID_MSK) | 00093 ((_brt_mode << STLED316S_CONF_BRT_MODE_SHFT) & STLED316S_CONF_BRT_MODE_MSK) | 00094 ((_bright << STLED316S_CONF_BRT_GLOB_SHFT) & STLED316S_CONF_BRT_GLOB_MSK); 00095 00096 _writeReg(STLED316S_IDX(STLED316S_CONF_PAGE,STLED316S_CONF_ADDR), config); // Config set command 00097 // printf("Cmd= 0x%02X, Conf= 0x%02X\r\n", STLED316S_ADDR_WR_CMD | STLED316S_IDX(STLED316S_CONF_PAGE,STLED316S_CONF_ADDR), config); // Debug 00098 } 00099 00100 00101 /** Set Individual LED Brightness (value is used in IndivBright mode) 00102 * 00103 * @param LedData_t leds pattern of LED data 00104 * @param char led_brt (3 significant bits, valid range 0..7 (1/16 .. 14/14 dutycycle) 00105 * @return none 00106 */ 00107 void STLED316S::setLedBrightness(LedData_t leds, char led_brt) { 00108 char brt_old; 00109 00110 //Sanity check 00111 led_brt &= STLED316S_BRT_MSK; 00112 00113 if (leds & STLED316S_LED_L1) { // LED_1 00114 brt_old = _readReg(STLED316S_IDX(STLED316S_LED_BRT_PAGE,STLED316S_LED1_2_BRT_ADDR)); // LED Brt read command 00115 _writeReg(STLED316S_IDX(STLED316S_LED_BRT_PAGE,STLED316S_LED1_2_BRT_ADDR), (brt_old & 0xF0) | led_brt); // LED Brt set command 00116 } 00117 00118 if (leds & STLED316S_LED_L2) { // LED_2 00119 brt_old = _readReg(STLED316S_IDX(STLED316S_LED_BRT_PAGE,STLED316S_LED1_2_BRT_ADDR)); // LED Brt read command 00120 _writeReg(STLED316S_IDX(STLED316S_LED_BRT_PAGE,STLED316S_LED1_2_BRT_ADDR), (brt_old & 0x0F) | (led_brt << 4)); // LED Brt set command 00121 } 00122 00123 if (leds & STLED316S_LED_L3) { // LED_3 00124 brt_old = _readReg(STLED316S_IDX(STLED316S_LED_BRT_PAGE,STLED316S_LED3_4_BRT_ADDR)); // LED Brt read command 00125 _writeReg(STLED316S_IDX(STLED316S_LED_BRT_PAGE,STLED316S_LED3_4_BRT_ADDR), (brt_old & 0xF0) | led_brt); // LED Brt set command 00126 } 00127 00128 if (leds & STLED316S_LED_L4) { // LED_4 00129 brt_old = _readReg(STLED316S_IDX(STLED316S_LED_BRT_PAGE,STLED316S_LED3_4_BRT_ADDR)); // LED Brt read command 00130 _writeReg(STLED316S_IDX(STLED316S_LED_BRT_PAGE,STLED316S_LED3_4_BRT_ADDR), (brt_old & 0x0F) | (led_brt << 4)); // LED Brt set command 00131 } 00132 00133 if (leds & STLED316S_LED_L5) { // LED_5 00134 brt_old = _readReg(STLED316S_IDX(STLED316S_LED_BRT_PAGE,STLED316S_LED5_6_BRT_ADDR)); // LED Brt read command 00135 _writeReg(STLED316S_IDX(STLED316S_LED_BRT_PAGE,STLED316S_LED5_6_BRT_ADDR), (brt_old & 0xF0) | led_brt); // LED Brt set command 00136 } 00137 00138 if (leds & STLED316S_LED_L6) { // LED_6 00139 brt_old = _readReg(STLED316S_IDX(STLED316S_LED_BRT_PAGE,STLED316S_LED5_6_BRT_ADDR)); // LED Brt read command 00140 _writeReg(STLED316S_IDX(STLED316S_LED_BRT_PAGE,STLED316S_LED5_6_BRT_ADDR), (brt_old & 0x0F) | (led_brt << 4)); // LED Brt set command 00141 } 00142 00143 if (leds & STLED316S_LED_L7) { // LED_7 00144 brt_old = _readReg(STLED316S_IDX(STLED316S_LED_BRT_PAGE,STLED316S_LED7_8_BRT_ADDR)); // LED Brt read command 00145 _writeReg(STLED316S_IDX(STLED316S_LED_BRT_PAGE,STLED316S_LED7_8_BRT_ADDR), (brt_old & 0xF0) | led_brt); // LED Brt set command 00146 } 00147 00148 if (leds & STLED316S_LED_L8) { // LED_8 00149 brt_old = _readReg(STLED316S_IDX(STLED316S_LED_BRT_PAGE,STLED316S_LED7_8_BRT_ADDR)); // LED Brt read command 00150 _writeReg(STLED316S_IDX(STLED316S_LED_BRT_PAGE,STLED316S_LED7_8_BRT_ADDR), (brt_old & 0x0F) | (led_brt << 4)); // LED Brt set command 00151 } 00152 } 00153 00154 00155 /** Set Individual Digit Brightness (value is used in IndivBright mode) 00156 * 00157 * @param LedData_t digits pattern of Digit data 00158 * @param char dig_brt (3 significant bits, valid range 0..7 (1/16 .. 14/14 dutycycle) 00159 * @return none 00160 */ 00161 void STLED316S::setDigitBrightness(LedData_t digits, char dig_brt) { 00162 char brt_old; 00163 00164 //Sanity check 00165 dig_brt &= STLED316S_BRT_MSK; 00166 00167 if (digits & STLED316S_DIG_D2) { // DIGIT_2 00168 brt_old = _readReg(STLED316S_IDX(STLED316S_DIG_BRT_PAGE,STLED316S_DIG2_3_BRT_ADDR)); // Digit Brt read command 00169 _writeReg(STLED316S_IDX(STLED316S_DIG_BRT_PAGE,STLED316S_DIG2_3_BRT_ADDR), (brt_old & 0xF0) | dig_brt); // Digit Brt set command 00170 } 00171 00172 if (digits & STLED316S_DIG_D3) { // DIGIT_3 00173 brt_old = _readReg(STLED316S_IDX(STLED316S_DIG_BRT_PAGE,STLED316S_DIG2_3_BRT_ADDR)); // Digit Brt read command 00174 _writeReg(STLED316S_IDX(STLED316S_DIG_BRT_PAGE,STLED316S_DIG2_3_BRT_ADDR), (brt_old & 0x0F) | (dig_brt << 4)); // Digit Brt set command 00175 } 00176 00177 if (digits & STLED316S_DIG_D4) { // DIGIT_4 00178 brt_old = _readReg(STLED316S_IDX(STLED316S_DIG_BRT_PAGE,STLED316S_DIG4_5_BRT_ADDR)); // Digit Brt read command 00179 _writeReg(STLED316S_IDX(STLED316S_DIG_BRT_PAGE,STLED316S_DIG4_5_BRT_ADDR), (brt_old & 0xF0) | dig_brt); // Digit Brt set command 00180 } 00181 00182 if (digits & STLED316S_DIG_D5) { // DIGIT_5 00183 brt_old = _readReg(STLED316S_IDX(STLED316S_DIG_BRT_PAGE,STLED316S_DIG4_5_BRT_ADDR)); // Digit Brt read command 00184 _writeReg(STLED316S_IDX(STLED316S_DIG_BRT_PAGE,STLED316S_DIG4_5_BRT_ADDR), (brt_old & 0x0F) | (dig_brt << 4)); // Digit Brt set command 00185 } 00186 00187 if (digits & STLED316S_DIG_D6) { // DIGIT_6 00188 brt_old = _readReg(STLED316S_IDX(STLED316S_DIG_BRT_PAGE,STLED316S_DIG6_7_BRT_ADDR)); // Digit Brt read command 00189 _writeReg(STLED316S_IDX(STLED316S_DIG_BRT_PAGE,STLED316S_DIG6_7_BRT_ADDR), (brt_old & 0xF0) | dig_brt); // Digit Brt set command 00190 } 00191 00192 if (digits & STLED316S_DIG_D7) { // DIGIT_7 00193 brt_old = _readReg(STLED316S_IDX(STLED316S_DIG_BRT_PAGE,STLED316S_DIG6_7_BRT_ADDR)); // Digit Brt read command 00194 _writeReg(STLED316S_IDX(STLED316S_DIG_BRT_PAGE,STLED316S_DIG6_7_BRT_ADDR), (brt_old & 0x0F) | (dig_brt << 4)); // Digit Brt set command 00195 } 00196 } 00197 00198 00199 /** Set the Display mode On/off 00200 * 00201 * @param bool display mode 00202 */ 00203 void STLED316S::setDisplay(bool on) { 00204 00205 if (on) { 00206 _writeReg(STLED316S_IDX(STLED316S_DSP_PAGE,STLED316S_DSP_ON_ADDR)); // Display On command 00207 } 00208 else { 00209 _writeReg(STLED316S_IDX(STLED316S_DSP_PAGE,STLED316S_DSP_OFF_ADDR)); // Display Off command 00210 } 00211 } 00212 00213 /** Set Brightness mode 00214 * 00215 * @param BrightMode brt_mode (value is IndivBright or GlobalBright) 00216 * @return none 00217 */ 00218 void STLED316S::setBrightMode(BrightMode brt_mode) { 00219 char config; 00220 00221 _brt_mode = brt_mode; // mask invalid bits 00222 00223 config = ((_mode << STLED316S_CONF_GRID_SHFT) & STLED316S_CONF_GRID_MSK) | 00224 ((_brt_mode << STLED316S_CONF_BRT_MODE_SHFT) & STLED316S_CONF_BRT_MODE_MSK) | 00225 ((_bright << STLED316S_CONF_BRT_GLOB_SHFT) & STLED316S_CONF_BRT_GLOB_MSK); 00226 00227 _writeReg(STLED316S_IDX(STLED316S_CONF_PAGE,STLED316S_CONF_ADDR), config); // Config set command 00228 // printf("Cmd= 0x%02X, Conf= 0x%02X\r\n", STLED316S_ADDR_WR_CMD | STLED316S_IDX(STLED316S_CONF_PAGE,STLED316S_CONF_ADDR), config); // Debug 00229 } 00230 00231 00232 /** Write databyte to STLED316S 00233 * @param int address display memory location to write byte 00234 * @param char data byte written at given address 00235 * @return none 00236 */ 00237 void STLED316S::writeData(int address, char data) { 00238 _cs=0; 00239 wait_us(1); 00240 00241 _spi.write(_flip(STLED316S_ADDR_WR_CMD | STLED316S_IDX(STLED316S_DIG_PAGE, address))); // Address set cmd 00242 _spi.write(_flip(data)); // data 00243 00244 wait_us(1); 00245 _cs=1; 00246 } 00247 00248 /** Write Display datablock to STLED316S 00249 * @param DisplayData_t data Array of STLED316S_DISPLAY_MEM (=6) bytes for displaydata (starting at address 0) 00250 * @param length number bytes to write (valide range 0..STLED316S_DISPLAY_MEM (=6), starting at address 0) 00251 * @return none 00252 */ 00253 void STLED316S::writeData(DisplayData_t data, int length) { 00254 _cs=0; 00255 wait_us(1); 00256 00257 _spi.write(_flip(STLED316S_ADDR_WR_CMD | STLED316S_IDX(STLED316S_DIG_PAGE, 0x00))); // Set Address at 0 00258 00259 // printf("Cmd= 0x%02X\r\n", STLED316S_ADDR_WR_CMD | STLED316S_IDX(STLED316S_DIG_PAGE, 0x00)); // Debug 00260 00261 // sanity check 00262 if (length < 0) {length = 0;} 00263 if (length > STLED316S_DISPLAY_MEM) {length = STLED316S_DISPLAY_MEM;} 00264 00265 // for (int idx=0; idx<STLED316S_DISPLAY_MEM; idx++) { 00266 for (int idx=0; idx<length; idx++) { 00267 _spi.write(_flip(data[idx])); // data 00268 00269 // printf("Data= 0x%02X\r\n", data[idx]); // Debug 00270 } 00271 00272 wait_us(1); 00273 _cs=1; 00274 } 00275 00276 00277 /** Write LED data to STLED316S 00278 * @param LedData_t leds LED data 00279 * @return none 00280 */ 00281 void STLED316S::writeLedData(LedData_t leds) { 00282 _cs=0; 00283 wait_us(1); 00284 00285 _leds = leds; 00286 00287 _spi.write(_flip(STLED316S_ADDR_WR_CMD | STLED316S_IDX(STLED316S_DIG1_LED_PAGE, STLED316S_DIG1_LED_ADDR))); // Set Address 00288 // printf("Cmd= 0x%02Xr\n", STLED316S_ADDR_WR_CMD | STLED316S_IDX(STLED316S_DIG1_LED_PAGE, STLED316S_DIG1_LED_ADDR)); // Debug 00289 00290 _spi.write(_flip(leds)); // data 00291 // printf("Data 0x%02X\r\n", leds); // Debug 00292 00293 wait_us(1); 00294 _cs=1; 00295 } 00296 00297 00298 /** Set LED 00299 * 00300 * @param LedData_t leds pattern of LED data 00301 * @return none 00302 */ 00303 void STLED316S::setLed(LedData_t leds){ 00304 00305 _leds |= leds; 00306 writeLedData(_leds); 00307 } 00308 00309 /** Clr LED 00310 * 00311 * @param LedData_t leds pattern of LED data 00312 * @return none 00313 */ 00314 void STLED316S::clrLed(LedData_t leds){ 00315 _leds &= ~leds; 00316 writeLedData(_leds); 00317 } 00318 00319 /** Read keydata block from STLED316S 00320 * @param *keydata Ptr to Array of STLED316S_KEY_MEM (=5) bytes for keydata 00321 * @return bool keypress True when at least one key was pressed 00322 * 00323 * Note: Due to the hardware configuration the STLED316S key matrix scanner will detect multiple keys pressed at same time, 00324 * but this may also result in some spurious keys being set in keypress data array. 00325 * It may be best to ignore all keys in those situations. That option is implemented in this method depending on #define setting. 00326 */ 00327 bool STLED316S::getKeys(KeyData_t *keydata) { 00328 int keypress = 0; 00329 char data; 00330 00331 // Read keys 00332 _cs=0; 00333 wait_us(1); 00334 00335 // Enable Key Read mode 00336 _spi.write(_flip(STLED316S_ADDR_RD_CMD | STLED316S_IDX(STLED316S_KEY_PAGE, STLED316S_KEY1_ADDR))); // Set Address 00337 // printf("Cmd= 0x%02Xr\n", STLED316S_ADDR_RD_CMD | STLED316S_IDX(STLED316S_KEY_PAGE, STLED316S_KEY1_ADDR)); // Debug 00338 00339 for (int idx=0; idx < STLED316S_KEY_MEM; idx++) { 00340 data = _flip(_spi.write(0xFF)); // read keys and correct bitorder 00341 // printf("KeyData 0x%02X\r\n", data); // Debug 00342 00343 data = data & STLED316S_KEY_MSK; // Mask valid bits 00344 if (data != 0) { // Check for any pressed key 00345 for (int bit=0; bit < 8; bit++) { 00346 if (data & (1 << bit)) {keypress++;} // Test all significant bits 00347 } 00348 } 00349 00350 (*keydata)[idx] = data; // Store keydata after correcting bitorder 00351 } 00352 00353 wait_us(1); 00354 _cs=1; 00355 00356 #if(1) 00357 // Dismiss multiple keypresses at same time 00358 return (keypress == 1); 00359 #else 00360 // Allow multiple keypress and accept possible spurious keys 00361 return (keypress > 0); 00362 #endif 00363 } 00364 00365 00366 /** Helper to reverse all command or databits. The STLED316S expects LSB first, whereas SPI is MSB first 00367 * @param char data 00368 * @return bitreversed data 00369 */ 00370 char STLED316S::_flip(char data) { 00371 char value=0; 00372 00373 if (data & 0x01) {value |= 0x80;} ; 00374 if (data & 0x02) {value |= 0x40;} ; 00375 if (data & 0x04) {value |= 0x20;} ; 00376 if (data & 0x08) {value |= 0x10;} ; 00377 if (data & 0x10) {value |= 0x08;} ; 00378 if (data & 0x20) {value |= 0x04;} ; 00379 if (data & 0x40) {value |= 0x02;} ; 00380 if (data & 0x80) {value |= 0x01;} ; 00381 return value; 00382 } 00383 00384 00385 /** Write parameter to STLED316S Register 00386 * @param int idx Register address 00387 * @Param int data Parameter for Register 00388 * @return none 00389 */ 00390 void STLED316S::_writeReg(int idx, int data){ 00391 00392 _cs=0; 00393 wait_us(1); 00394 00395 _spi.write(_flip(STLED316S_ADDR_WR_CMD | (idx & STLED316S_IDX_MSK))); 00396 _spi.write(_flip(data) ); 00397 00398 // printf("Cmd= 0x%02X, Write= 0x%02X\r\n", STLED316S_ADDR_WR_CMD | (idx & STLED316S_IDX_MSK), data); // Debug 00399 00400 wait_us(1); 00401 _cs=1; 00402 } 00403 00404 00405 /** Write merged command and parameter to STLED316S 00406 * @param int cmd Command & Parameter byte 00407 * @return none 00408 */ 00409 void STLED316S::_writeReg(int cmd){ 00410 00411 _cs=0; 00412 wait_us(1); 00413 _spi.write(_flip(STLED316S_ADDR_WR_CMD | (cmd & STLED316S_IDX_MSK))); 00414 00415 // printf("Cmd= 0x%02X\r\n", STLED316S_ADDR_WR_CMD | (cmd & STLED316S_IDX_MSK)); // Debug 00416 00417 wait_us(1); 00418 _cs=1; 00419 } 00420 00421 00422 /** Read parameter from STLED316S Register 00423 * @param int idx Register address 00424 * @return char data from Register 00425 */ 00426 char STLED316S::_readReg(int idx){ 00427 char data; 00428 00429 _cs=0; 00430 wait_us(1); 00431 _spi.write(_flip(STLED316S_ADDR_RD_CMD | (idx & STLED316S_IDX_MSK))); 00432 00433 data = _flip(_spi.write(0xFF)); // read data and correct bitorder 00434 00435 // printf("Cmd= 0x%02X, Read= 0x%02X\r\n", STLED316S_ADDR_RD_CMD | (idx & STLED316S_IDX_MSK), data); // Debug 00436 00437 wait_us(1); 00438 _cs=1; 00439 00440 return data; 00441 } 00442 00443 00444 00445 #if(ST316BOARD_TEST == 1) 00446 // Derived class for STLED316S used in test display module 00447 // 00448 //#include "Font_7Seg.h" 00449 00450 /** Constructor for class for driving STM STLED316S controller as used in ST316S test display 00451 * 00452 * @brief Supports 6 Digits of 7 Segments and 3 LEDs. Also supports a scanned keyboard of 3. 00453 * 00454 * @param PinName mosi, miso, sclk, cs SPI bus pins 00455 */ 00456 STLED316S_BOARD::STLED316S_BOARD(PinName mosi, PinName miso, PinName sclk, PinName cs) : STLED316S(mosi, miso, sclk, cs, Grid6_Seg8) { 00457 _column = 0; 00458 _columns = ST316BOARD_NR_DIGITS; 00459 } 00460 00461 #if(0) 00462 #if DOXYGEN_ONLY 00463 /** Write a character to the Display 00464 * 00465 * @param c The character to write to the display 00466 */ 00467 int putc(int c); 00468 00469 /** Write a formatted string to the Display 00470 * 00471 * @param format A printf-style format string, followed by the 00472 * variables to use in formatting the string. 00473 */ 00474 int printf(const char* format, ...); 00475 #endif 00476 #endif 00477 00478 /** Locate cursor to a screen column 00479 * 00480 * @param column The horizontal position from the left, indexed from 0 00481 */ 00482 void STLED316S_BOARD::locate(int column) { 00483 //sanity check 00484 if (column < 0) {column = 0;} 00485 if (column > (_columns - 1)) {column = _columns - 1;} 00486 00487 _column = column; 00488 } 00489 00490 00491 /** Number of screen columns 00492 * 00493 * @param none 00494 * @return columns 00495 */ 00496 int STLED316S_BOARD::columns() { 00497 return _columns; 00498 } 00499 00500 00501 /** Clear the screen and locate to 0 00502 * @param bool clrAll Clear Icons also (default = false) 00503 */ 00504 void STLED316S_BOARD::cls(bool clrAll) { 00505 00506 if (clrAll) { 00507 //clear local buffer for LEDs/Icons 00508 setLed(0x00); 00509 } 00510 00511 //clear local buffer for digits 00512 for (int idx=0; idx < (ST316BOARD_NR_GRIDS * STLED316S_BYTES_PER_GRID); idx++) { 00513 _displaybuffer[idx] = 0x00; 00514 } 00515 writeData(_displaybuffer, (ST316BOARD_NR_GRIDS * STLED316S_BYTES_PER_GRID)); 00516 00517 _column = 0; 00518 } 00519 00520 00521 /** Set Icon 00522 * 00523 * @param Icon icon Enums Icon has Grid position encoded in 8 MSBs, Icon pattern encoded in 16 LSBs 00524 * @return none 00525 */ 00526 void STLED316S_BOARD::setIcon(Icon icon) { 00527 // int addr; 00528 int icn; 00529 00530 icn = icon & 0xFF; 00531 // addr = (icon >> 24) & 0xFF; 00532 00533 setLed(icn); 00534 } 00535 00536 /** Clr Icon 00537 * 00538 * @param Icon icon Enums Icon has Grid position encoded in 8 MSBs, Icon pattern encoded in 16 LSBs 00539 * @return none 00540 */ 00541 void STLED316S_BOARD::clrIcon(Icon icon) { 00542 // int addr; 00543 int icn; 00544 00545 icn = icon & 0xFF; 00546 // addr = (icon >> 24) & 0xFF; 00547 00548 clrLed(icn); 00549 } 00550 00551 00552 /** Set User Defined Characters (UDC) 00553 * 00554 * @param unsigned char udc_idx The Index of the UDC (0..7) 00555 * @param int udc_data The bitpattern for the UDC (16 bits) 00556 */ 00557 void STLED316S_BOARD::setUDC(unsigned char udc_idx, int udc_data) { 00558 00559 //Sanity check 00560 if (udc_idx > (ST316BOARD_NR_UDC-1)) { 00561 return; 00562 } 00563 // Mask out Icon bits? 00564 00565 _UDC_7S[udc_idx] = udc_data; 00566 } 00567 00568 00569 /** Write a single character (Stream implementation) 00570 */ 00571 int STLED316S_BOARD::_putc(int value) { 00572 00573 int addr; 00574 bool validChar = false; 00575 char pattern = 0x00; 00576 00577 if ((value == '\n') || (value == '\r')) { 00578 //No character to write 00579 validChar = false; 00580 00581 //Update Cursor 00582 _column = 0; 00583 } 00584 else if ((value == '.') || (value == ',')) { 00585 //No character to write 00586 validChar = false; 00587 pattern = S7_DP; // placeholder for all DPs 00588 00589 // Check to see that DP can be shown for current column 00590 if (_column > 0) { 00591 //Translate between _column and displaybuffer entries 00592 //Add DP to bitpattern of digit left of current column. 00593 addr = (_column - 1); 00594 00595 //Set bits for decimal point to write 00596 _displaybuffer[addr] = _displaybuffer[addr] | pattern; 00597 writeData(_displaybuffer, (ST316BOARD_NR_GRIDS * STLED316S_BYTES_PER_GRID)); 00598 00599 //No Cursor Update 00600 } 00601 } 00602 else if ((value >= 0) && (value < ST316BOARD_NR_UDC)) { 00603 //Character to write 00604 validChar = true; 00605 pattern = _UDC_7S[value]; 00606 } 00607 00608 #if (SHOW_ASCII == 1) 00609 //display all ASCII characters 00610 else if ((value >= FONT_7S_START) && (value <= FONT_7S_END)) { 00611 //Character to write 00612 validChar = true; 00613 pattern = FONT_7S[value - FONT_7S_START]; 00614 } // else 00615 #else 00616 //display only digits and hex characters 00617 else if (value == '-') { 00618 //Character to write 00619 validChar = true; 00620 pattern = C7_MIN; 00621 } 00622 else if ((value >= (int)'0') && (value <= (int) '9')) { 00623 //Character to write 00624 validChar = true; 00625 pattern = FONT_7S[value - (int) '0']; 00626 } 00627 else if ((value >= (int) 'A') && (value <= (int) 'F')) { 00628 //Character to write 00629 validChar = true; 00630 pattern = FONT_7S[10 + value - (int) 'A']; 00631 } 00632 else if ((value >= (int) 'a') && (value <= (int) 'f')) { 00633 //Character to write 00634 validChar = true; 00635 pattern = FONT_7S[10 + value - (int) 'a']; 00636 } //else 00637 #endif 00638 00639 if (validChar) { 00640 //Character to write 00641 00642 //Translate between _column and displaybuffer entries 00643 //_column == 0 => Grid0 => addr = 0 00644 // 00645 //_column == 5 => Grid5 => addr = 5 00646 addr = _column; 00647 _displaybuffer[addr] = pattern; 00648 00649 writeData(_displaybuffer, (ST316BOARD_NR_GRIDS * STLED316S_BYTES_PER_GRID)); 00650 00651 //Update Cursor 00652 _column++; 00653 if (_column > (ST316BOARD_NR_DIGITS - 1)) { 00654 _column = 0; 00655 } 00656 00657 } // if validChar 00658 00659 return value; 00660 } 00661 00662 // get a single character (Stream implementation) 00663 int STLED316S_BOARD::_getc() { 00664 return -1; 00665 } 00666 #endif
Generated on Sat Jul 16 2022 20:44:27 by
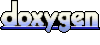