This is the final version of Mini Gateway for Automation and Security desgined for Renesas GR Peach Design Contest
Dependencies: GR-PEACH_video GraphicsFramework HTTPServer R_BSP mbed-rpc mbed-rtos Socket lwip-eth lwip-sys lwip FATFileSystem
Fork of mbed-os-example-mbed5-blinky by
Drivers
Data Structures | |
class | AnalogIn |
An analog input, used for reading the voltage on a pin. More... | |
class | AnalogOut |
An analog output, used for setting the voltage on a pin. More... | |
class | BusIn |
A digital input bus, used for reading the state of a collection of pins. More... | |
class | BusInOut |
A digital input output bus, used for setting the state of a collection of pins. More... | |
class | BusOut |
A digital output bus, used for setting the state of a collection of pins. More... | |
class | CANMessage |
CANMessage class. More... | |
class | CAN |
A can bus client, used for communicating with can devices. More... | |
class | DigitalIn |
A digital input, used for reading the state of a pin. More... | |
class | DigitalInOut |
A digital input/output, used for setting or reading a bi-directional pin. More... | |
class | DigitalOut |
A digital output, used for setting the state of a pin. More... | |
class | DirHandle |
Represents a directory stream. More... | |
class | Ethernet |
An ethernet interface, to use with the ethernet pins. More... | |
class | FileHandle |
An OO equivalent of the internal FILEHANDLE variable and associated _sys_* functions. More... | |
class | FileSystemLike |
A filesystem-like object is one that can be used to open files though it by fopen("/name/filename", mode) More... | |
class | I2C |
An I2C Master, used for communicating with I2C slave devices. More... | |
class | I2CSlave |
An I2C Slave, used for communicating with an I2C Master device. More... | |
class | InterruptIn |
A digital interrupt input, used to call a function on a rising or falling edge. More... | |
class | InterruptManager |
Use this singleton if you need to chain interrupt handlers. More... | |
class | LocalFileSystem |
A filesystem for accessing the local mbed Microcontroller USB disk drive. More... | |
class | LowPowerTicker |
Low Power Ticker. More... | |
class | LowPowerTimeout |
Low Power Timout. More... | |
class | LowPowerTimer |
Low power timer. More... | |
class | PortIn |
A multiple pin digital input. More... | |
class | PortInOut |
A multiple pin digital in/out used to set/read multiple bi-directional pins. More... | |
class | PortOut |
A multiple pin digital out. More... | |
class | PwmOut |
A pulse-width modulation digital output. More... | |
class | RawSerial |
A serial port (UART) for communication with other serial devices This is a variation of the Serial class that doesn't use streams, thus making it safe to use in interrupt handlers with the RTOS. More... | |
class | Serial |
A serial port (UART) for communication with other serial devices. More... | |
class | SerialBase |
A base class for serial port implementations Can't be instantiated directly (use Serial or RawSerial) More... | |
class | SPI |
A SPI Master, used for communicating with SPI slave devices. More... | |
class | SPISlave |
A SPI slave, used for communicating with a SPI Master device. More... | |
class | Stream |
File stream. More... | |
class | Ticker |
A Ticker is used to call a function at a recurring interval. More... | |
class | Timeout |
A Timeout is used to call a function at a point in the future. More... | |
class | Timer |
A general purpose timer. More... | |
class | TimerEvent |
Base abstraction for timer interrupts. More... | |
Functions | |
float | read () |
Read the input voltage, represented as a float in the range [0.0, 1.0]. | |
unsigned short | read_u16 () |
Read the input voltage, represented as an unsigned short in the range [0x0, 0xFFFF]. | |
operator float () | |
An operator shorthand for read() | |
void | write (float value) |
Set the output voltage, specified as a percentage (float) | |
void | write_u16 (unsigned short value) |
Set the output voltage, represented as an unsigned short in the range [0x0, 0xFFFF]. | |
float | read () |
Return the current output voltage setting, measured as a percentage (float) | |
AnalogOut & | operator= (float percent) |
An operator shorthand for write() | |
operator float () | |
An operator shorthand for read() | |
int | read () |
Read the value of the input bus. | |
void | mode (PinMode pull) |
Set the input pin mode. | |
int | mask () |
Binary mask of bus pins connected to actual pins (not NC pins) If bus pin is in NC state make corresponding bit will be cleared (set to 0), else bit will be set to 1. | |
operator int () | |
A shorthand for read() | |
DigitalIn & | operator[] (int index) |
Access to particular bit in random-iterator fashion. | |
void | write (int value) |
Write the value to the output bus. | |
int | read () |
Read the value currently output on the bus. | |
void | output () |
Set as an output. | |
void | input () |
Set as an input. | |
void | mode (PinMode pull) |
Set the input pin mode. | |
int | mask () |
Binary mask of bus pins connected to actual pins (not NC pins) If bus pin is in NC state make corresponding bit will be cleared (set to 0), else bit will be set to 1. | |
BusInOut & | operator= (int v) |
A shorthand for write() | |
DigitalInOut & | operator[] (int index) |
Access to particular bit in random-iterator fashion. | |
operator int () | |
A shorthand for read() | |
void | write (int value) |
Write the value to the output bus. | |
int | read () |
Read the value currently output on the bus. | |
int | mask () |
Binary mask of bus pins connected to actual pins (not NC pins) If bus pin is in NC state make corresponding bit will be cleared (set to 0), else bit will be set to 1. | |
BusOut & | operator= (int v) |
A shorthand for write() | |
DigitalOut & | operator[] (int index) |
Access to particular bit in random-iterator fashion. | |
operator int () | |
A shorthand for read() | |
CANMessage (int _id, const char *_data, char _len=8, CANType _type=CANData, CANFormat _format=CANStandard) | |
Creates CAN message with specific content. | |
CANMessage (int _id, CANFormat _format=CANStandard) | |
Creates CAN remote message. | |
int | frequency (int hz) |
Set the frequency of the CAN interface. | |
int | write (CANMessage msg) |
Write a CANMessage to the bus. | |
int | read (CANMessage &msg, int handle=0) |
Read a CANMessage from the bus. | |
void | reset () |
Reset CAN interface. | |
void | monitor (bool silent) |
Puts or removes the CAN interface into silent monitoring mode. | |
int | mode (Mode mode) |
Change CAN operation to the specified mode. | |
int | filter (unsigned int id, unsigned int mask, CANFormat format=CANAny, int handle=0) |
Filter out incomming messages. | |
unsigned char | rderror () |
Returns number of read errors to detect read overflow errors. | |
unsigned char | tderror () |
Returns number of write errors to detect write overflow errors. | |
void | attach (Callback< void()> func, IrqType type=RxIrq) |
Attach a function to call whenever a CAN frame received interrupt is generated. | |
template<typename T > | |
void | attach (T *obj, void(T::*method)(), IrqType type=RxIrq) |
Attach a member function to call whenever a CAN frame received interrupt is generated. | |
template<typename T > | |
void | attach (T *obj, void(*method)(T *), IrqType type=RxIrq) |
Attach a member function to call whenever a CAN frame received interrupt is generated. | |
DigitalIn (PinName pin, PinMode mode) | |
Create a DigitalIn connected to the specified pin. | |
int | read () |
Read the input, represented as 0 or 1 (int) | |
void | mode (PinMode pull) |
Set the input pin mode. | |
int | is_connected () |
Return the output setting, represented as 0 or 1 (int) | |
operator int () | |
An operator shorthand for read() | |
DigitalInOut (PinName pin, PinDirection direction, PinMode mode, int value) | |
Create a DigitalInOut connected to the specified pin. | |
void | write (int value) |
Set the output, specified as 0 or 1 (int) | |
int | read () |
Return the output setting, represented as 0 or 1 (int) | |
void | output () |
Set as an output. | |
void | input () |
Set as an input. | |
void | mode (PinMode pull) |
Set the input pin mode. | |
int | is_connected () |
Return the output setting, represented as 0 or 1 (int) | |
DigitalInOut & | operator= (int value) |
A shorthand for write() | |
operator int () | |
A shorthand for read() | |
DigitalOut (PinName pin, int value) | |
Create a DigitalOut connected to the specified pin. | |
void | write (int value) |
Set the output, specified as 0 or 1 (int) | |
int | read () |
Return the output setting, represented as 0 or 1 (int) | |
int | is_connected () |
Return the output setting, represented as 0 or 1 (int) | |
DigitalOut & | operator= (int value) |
A shorthand for write() | |
operator int () | |
A shorthand for read() | |
virtual struct dirent * | readdir ()=0 |
Return the directory entry at the current position, and advances the position to the next entry. | |
virtual void | rewinddir ()=0 |
Resets the position to the beginning of the directory. | |
virtual off_t | telldir () |
Returns the current position of the DirHandle. | |
virtual void | seekdir (off_t location) |
Sets the position of the DirHandle. | |
virtual void | lock () |
Acquire exclusive access to this object. | |
virtual void | unlock () |
Release exclusive access to this object. | |
virtual | ~Ethernet () |
Powers the hardware down. | |
int | write (const char *data, int size) |
Writes into an outgoing ethernet packet. | |
int | send () |
Send an outgoing ethernet packet. | |
int | receive () |
Recevies an arrived ethernet packet. | |
int | read (char *data, int size) |
Read from an recevied ethernet packet. | |
void | address (char *mac) |
Gives the ethernet address of the mbed. | |
int | link () |
Returns if an ethernet link is pressent or not. | |
void | set_link (Mode mode) |
Sets the speed and duplex parameters of an ethernet link. | |
virtual int | close ()=0 |
Close the file. | |
virtual ssize_t | read (void *buffer, size_t length)=0 |
Function read Reads the contents of the file into a buffer. | |
virtual int | isatty ()=0 |
Check if the handle is for a interactive terminal device. | |
virtual off_t | lseek (off_t offset, int whence)=0 |
Move the file position to a given offset from a given location. | |
virtual int | fsync ()=0 |
Flush any buffers associated with the FileHandle, ensuring it is up to date on disk. | |
virtual void | lock () |
Acquire exclusive access to this object. | |
virtual void | unlock () |
Release exclusive access to this object. | |
virtual FileHandle * | open (const char *filename, int flags)=0 |
Opens a file from the filesystem. | |
virtual int | remove (const char *filename) |
Remove a file from the filesystem. | |
virtual int | rename (const char *oldname, const char *newname) |
Rename a file in the filesystem. | |
virtual DirHandle * | opendir (const char *name) |
Opens a directory in the filesystem and returns a DirHandle representing the directory stream. | |
virtual int | mkdir (const char *name, mode_t mode) |
Creates a directory in the filesystem. | |
I2C (PinName sda, PinName scl) | |
Create an I2C Master interface, connected to the specified pins. | |
void | frequency (int hz) |
Set the frequency of the I2C interface. | |
int | read (int address, char *data, int length, bool repeated=false) |
Read from an I2C slave. | |
int | read (int ack) |
Read a single byte from the I2C bus. | |
int | write (int address, const char *data, int length, bool repeated=false) |
Write to an I2C slave. | |
int | write (int data) |
Write single byte out on the I2C bus. | |
void | start (void) |
Creates a start condition on the I2C bus. | |
void | stop (void) |
Creates a stop condition on the I2C bus. | |
virtual void | lock (void) |
Acquire exclusive access to this I2C bus. | |
virtual void | unlock (void) |
Release exclusive access to this I2C bus. | |
int | transfer (int address, const char *tx_buffer, int tx_length, char *rx_buffer, int rx_length, const event_callback_t &callback, int event=I2C_EVENT_TRANSFER_COMPLETE, bool repeated=false) |
Start non-blocking I2C transfer. | |
void | abort_transfer () |
Abort the on-going I2C transfer. | |
I2CSlave (PinName sda, PinName scl) | |
Create an I2C Slave interface, connected to the specified pins. | |
void | frequency (int hz) |
Set the frequency of the I2C interface. | |
int | receive (void) |
Checks to see if this I2C Slave has been addressed. | |
int | read (char *data, int length) |
Read from an I2C master. | |
int | read (void) |
Read a single byte from an I2C master. | |
int | write (const char *data, int length) |
Write to an I2C master. | |
int | write (int data) |
Write a single byte to an I2C master. | |
void | address (int address) |
Sets the I2C slave address. | |
void | stop (void) |
Reset the I2C slave back into the known ready receiving state. | |
int | read () |
Read the input, represented as 0 or 1 (int) | |
operator int () | |
An operator shorthand for read() | |
void | rise (Callback< void()> func) |
Attach a function to call when a rising edge occurs on the input. | |
template<typename T , typename M > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","The rise function does not support cv-qualifiers. Replaced by ""rise(callback(obj, method)).") void rise(T *obj | |
Attach a member function to call when a rising edge occurs on the input. | |
void | fall (Callback< void()> func) |
Attach a function to call when a falling edge occurs on the input. | |
template<typename T , typename M > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","The fall function does not support cv-qualifiers. Replaced by ""fall(callback(obj, method)).") void fall(T *obj | |
Attach a member function to call when a falling edge occurs on the input. | |
void | enable_irq () |
Enable IRQ. | |
void | disable_irq () |
Disable IRQ. | |
static void | destroy () |
Destroy the current instance of the interrupt manager. | |
pFunctionPointer_t | add_handler (void(*function)(void), IRQn_Type irq) |
Add a handler for an interrupt at the end of the handler list. | |
pFunctionPointer_t | add_handler_front (void(*function)(void), IRQn_Type irq) |
Add a handler for an interrupt at the beginning of the handler list. | |
template<typename T > | |
pFunctionPointer_t | add_handler (T *tptr, void(T::*mptr)(void), IRQn_Type irq) |
Add a handler for an interrupt at the end of the handler list. | |
template<typename T > | |
pFunctionPointer_t | add_handler_front (T *tptr, void(T::*mptr)(void), IRQn_Type irq) |
Add a handler for an interrupt at the beginning of the handler list. | |
bool | remove_handler (pFunctionPointer_t handler, IRQn_Type irq) |
Remove a handler from an interrupt. | |
virtual FileHandle * | open (const char *name, int flags) |
Opens a file from the filesystem. | |
virtual int | remove (const char *filename) |
Remove a file from the filesystem. | |
virtual DirHandle * | opendir (const char *name) |
Opens a directory in the filesystem and returns a DirHandle representing the directory stream. | |
int | read () |
Read the value currently output on the port. | |
void | mode (PinMode mode) |
Set the input pin mode. | |
operator int () | |
A shorthand for read() | |
void | write (int value) |
Write the value to the output port. | |
int | read () |
Read the value currently output on the port. | |
void | output () |
Set as an output. | |
void | input () |
Set as an input. | |
void | mode (PinMode mode) |
Set the input pin mode. | |
PortInOut & | operator= (int value) |
A shorthand for write() | |
operator int () | |
A shorthand for read() | |
void | write (int value) |
Write the value to the output port. | |
int | read () |
Read the value currently output on the port. | |
PortOut & | operator= (int value) |
A shorthand for write() | |
operator int () | |
A shorthand for read() | |
void | write (float value) |
Set the ouput duty-cycle, specified as a percentage (float) | |
float | read () |
Return the current output duty-cycle setting, measured as a percentage (float) | |
void | period (float seconds) |
Set the PWM period, specified in seconds (float), keeping the duty cycle the same. | |
void | period_ms (int ms) |
Set the PWM period, specified in milli-seconds (int), keeping the duty cycle the same. | |
void | period_us (int us) |
Set the PWM period, specified in micro-seconds (int), keeping the duty cycle the same. | |
void | pulsewidth (float seconds) |
Set the PWM pulsewidth, specified in seconds (float), keeping the period the same. | |
void | pulsewidth_ms (int ms) |
Set the PWM pulsewidth, specified in milli-seconds (int), keeping the period the same. | |
void | pulsewidth_us (int us) |
Set the PWM pulsewidth, specified in micro-seconds (int), keeping the period the same. | |
PwmOut & | operator= (float value) |
A operator shorthand for write() | |
operator float () | |
An operator shorthand for read() | |
int | putc (int c) |
Write a char to the serial port. | |
int | getc () |
Read a char from the serial port. | |
int | puts (const char *str) |
Write a string to the serial port. | |
virtual void | lock (void) |
Acquire exclusive access to this serial port. | |
virtual void | unlock (void) |
Release exclusive access to this serial port. | |
Serial (PinName tx, PinName rx, const char *name=NULL, int baud=MBED_CONF_PLATFORM_DEFAULT_SERIAL_BAUD_RATE) | |
Create a Serial port, connected to the specified transmit and receive pins. | |
Serial (PinName tx, PinName rx, int baud) | |
Create a Serial port, connected to the specified transmit and receive pins, with the specified baud. | |
virtual void | lock () |
Acquire exclusive access to this serial port. | |
virtual void | unlock () |
Release exclusive access to this serial port. | |
void | format (int bits=8, Parity parity=SerialBase::None, int stop_bits=1) |
Set the transmission format used by the serial port. | |
int | readable () |
Determine if there is a character available to read. | |
int | writeable () |
Determine if there is space available to write a character. | |
void | attach (Callback< void()> func, IrqType type=RxIrq) |
Attach a function to call whenever a serial interrupt is generated. | |
template<typename T > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","The attach function does not support cv-qualifiers. Replaced by ""attach(callback(obj, method), type).") void attach(T *obj | |
Attach a member function to call whenever a serial interrupt is generated. | |
virtual void | lock (void) |
Acquire exclusive access to this serial port. | |
virtual void | unlock (void) |
Release exclusive access to this serial port. | |
void | set_flow_control (Flow type, PinName flow1=NC, PinName flow2=NC) |
Set the flow control type on the serial port. | |
int | write (const uint8_t *buffer, int length, const event_callback_t &callback, int event=SERIAL_EVENT_TX_COMPLETE) |
Begin asynchronous write using 8bit buffer. | |
int | write (const uint16_t *buffer, int length, const event_callback_t &callback, int event=SERIAL_EVENT_TX_COMPLETE) |
Begin asynchronous write using 16bit buffer. | |
void | abort_write () |
Abort the on-going write transfer. | |
int | read (uint8_t *buffer, int length, const event_callback_t &callback, int event=SERIAL_EVENT_RX_COMPLETE, unsigned char char_match=SERIAL_RESERVED_CHAR_MATCH) |
Begin asynchronous reading using 8bit buffer. | |
int | read (uint16_t *buffer, int length, const event_callback_t &callback, int event=SERIAL_EVENT_RX_COMPLETE, unsigned char char_match=SERIAL_RESERVED_CHAR_MATCH) |
Begin asynchronous reading using 16bit buffer. | |
void | abort_read () |
Abort the on-going read transfer. | |
int | set_dma_usage_tx (DMAUsage usage) |
Configure DMA usage suggestion for non-blocking TX transfers. | |
int | set_dma_usage_rx (DMAUsage usage) |
Configure DMA usage suggestion for non-blocking RX transfers. | |
void | format (int bits, int mode=0) |
Configure the data transmission format. | |
void | frequency (int hz=1000000) |
Set the spi bus clock frequency. | |
virtual int | write (int value) |
Write to the SPI Slave and return the response. | |
virtual void | lock (void) |
Acquire exclusive access to this SPI bus. | |
virtual void | unlock (void) |
Release exclusive access to this SPI bus. | |
template<typename Type > | |
int | transfer (const Type *tx_buffer, int tx_length, Type *rx_buffer, int rx_length, const event_callback_t &callback, int event=SPI_EVENT_COMPLETE) |
Start non-blocking SPI transfer using 8bit buffers. | |
void | abort_transfer () |
Abort the on-going SPI transfer, and continue with transfer's in the queue if any. | |
void | clear_transfer_buffer () |
Clear the transaction buffer. | |
void | abort_all_transfers () |
Clear the transaction buffer and abort on-going transfer. | |
int | set_dma_usage (DMAUsage usage) |
Configure DMA usage suggestion for non-blocking transfers. | |
void | irq_handler_asynch (void) |
SPI IRQ handler. | |
int | transfer (const void *tx_buffer, int tx_length, void *rx_buffer, int rx_length, unsigned char bit_width, const event_callback_t &callback, int event) |
Common transfer method. | |
int | queue_transfer (const void *tx_buffer, int tx_length, void *rx_buffer, int rx_length, unsigned char bit_width, const event_callback_t &callback, int event) |
void | start_transfer (const void *tx_buffer, int tx_length, void *rx_buffer, int rx_length, unsigned char bit_width, const event_callback_t &callback, int event) |
Configures a callback, spi peripheral and initiate a new transfer. | |
void | start_transaction (transaction_t *data) |
Start a new transaction. | |
void | dequeue_transaction () |
Dequeue a transaction. | |
void | format (int bits, int mode=0) |
Configure the data transmission format. | |
void | frequency (int hz=1000000) |
Set the spi bus clock frequency. | |
int | receive (void) |
Polls the SPI to see if data has been received. | |
int | read (void) |
Retrieve data from receive buffer as slave. | |
void | reply (int value) |
Fill the transmission buffer with the value to be written out as slave on the next received message from the master. | |
virtual int | close () |
Close the file. | |
virtual ssize_t | write (const void *buffer, size_t length) |
Write the contents of a buffer to the file. | |
virtual ssize_t | read (void *buffer, size_t length) |
Function read Reads the contents of the file into a buffer. | |
virtual off_t | lseek (off_t offset, int whence) |
Move the file position to a given offset from a given location. | |
virtual int | isatty () |
Check if the handle is for a interactive terminal device. | |
virtual int | fsync () |
Flush any buffers associated with the FileHandle, ensuring it is up to date on disk. | |
void | attach (Callback< void()> func, float t) |
Attach a function to be called by the Ticker, specifiying the interval in seconds. | |
template<typename T , typename M > | |
MBED_DEPRECATED_SINCE ("mbed-os-5.1","The attach function does not support cv-qualifiers. Replaced by ""attach(callback(obj, method), t).") void attach(T *obj | |
Attach a member function to be called by the Ticker, specifiying the interval in seconds. | |
void | start () |
Start the timer. | |
void | stop () |
Stop the timer. | |
void | reset () |
Reset the timer to 0. | |
float | read () |
Get the time passed in seconds. | |
int | read_ms () |
Get the time passed in mili-seconds. | |
int | read_us () |
Get the time passed in micro-seconds. | |
operator float () | |
An operator shorthand for read() | |
static void | irq (uint32_t id) |
The handler registered with the underlying timer interrupt. | |
virtual | ~TimerEvent () |
Destruction removes it... | |
Variables | |
int | _nc_mask |
Mask of bus's NC pins If bit[n] is set to 1 - pin is connected if bit[n] is cleared - pin is not connected (NC) | |
int | _nc_mask |
Mask of bus's NC pins If bit[n] is set to 1 - pin is connected if bit[n] is cleared - pin is not connected (NC) | |
int | _nc_mask |
Mask of bus's NC pins If bit[n] is set to 1 - pin is connected if bit[n] is cleared - pin is not connected (NC) | |
timestamp_t | _delay |
Time delay (in microseconds) for re-setting the multi-shot callback. | |
Callback< void()> | _function |
Callback. |
Function Documentation
void abort_all_transfers | ( | ) | [inherited] |
void abort_read | ( | void | ) | [inherited] |
Abort the on-going read transfer.
Definition at line 184 of file SerialBase.cpp.
void abort_transfer | ( | void | ) | [inherited] |
void abort_transfer | ( | void | ) | [inherited] |
void abort_write | ( | void | ) | [inherited] |
Abort the on-going write transfer.
Definition at line 179 of file SerialBase.cpp.
pFunctionPointer_t add_handler | ( | T * | tptr, |
void(T::*)(void) | mptr, | ||
IRQn_Type | irq | ||
) | [inherited] |
Add a handler for an interrupt at the end of the handler list.
- Parameters:
-
tptr pointer to the object that has the handler function mptr pointer to the actual handler function irq interrupt number
- Returns:
- The function object created for 'tptr' and 'mptr'
Definition at line 102 of file InterruptManager.h.
pFunctionPointer_t add_handler | ( | void(*)(void) | function, |
IRQn_Type | irq | ||
) | [inherited] |
Add a handler for an interrupt at the end of the handler list.
- Parameters:
-
function the handler to add irq interrupt number
- Returns:
- The function object created for 'function'
Definition at line 74 of file InterruptManager.h.
pFunctionPointer_t add_handler_front | ( | void(*)(void) | function, |
IRQn_Type | irq | ||
) | [inherited] |
Add a handler for an interrupt at the beginning of the handler list.
- Parameters:
-
function the handler to add irq interrupt number
- Returns:
- The function object created for 'function'
Definition at line 87 of file InterruptManager.h.
pFunctionPointer_t add_handler_front | ( | T * | tptr, |
void(T::*)(void) | mptr, | ||
IRQn_Type | irq | ||
) | [inherited] |
Add a handler for an interrupt at the beginning of the handler list.
- Parameters:
-
tptr pointer to the object that has the handler function mptr pointer to the actual handler function irq interrupt number
- Returns:
- The function object created for 'tptr' and 'mptr'
Definition at line 117 of file InterruptManager.h.
void address | ( | int | address ) | [inherited] |
Sets the I2C slave address.
- Parameters:
-
address The address to set for the slave (ignoring the least signifcant bit). If set to 0, the slave will only respond to the general call address.
Definition at line 32 of file I2CSlave.cpp.
void address | ( | char * | mac ) | [inherited] |
Gives the ethernet address of the mbed.
- Parameters:
-
mac Must be a pointer to a 6 byte char array to copy the ethernet address in.
Definition at line 48 of file Ethernet.cpp.
void attach | ( | T * | obj, |
void(*)(T *) | method, | ||
IrqType | type = RxIrq |
||
) | [inherited] |
Attach a member function to call whenever a CAN frame received interrupt is generated.
- Parameters:
-
obj pointer to the object to call the member function on method pointer to the member function to be called event Which CAN interrupt to attach the member function to (CAN::RxIrq for message received, TxIrq for transmitted or aborted, EwIrq for error warning, DoIrq for data overrun, WuIrq for wake-up, EpIrq for error passive, AlIrq for arbitration lost, BeIrq for bus error)
void attach | ( | Callback< void()> | func, |
IrqType | type = RxIrq |
||
) | [inherited] |
Attach a function to call whenever a CAN frame received interrupt is generated.
- Parameters:
-
func A pointer to a void function, or 0 to set as none event Which CAN interrupt to attach the member function to (CAN::RxIrq for message received, CAN::TxIrq for transmitted or aborted, CAN::EwIrq for error warning, CAN::DoIrq for data overrun, CAN::WuIrq for wake-up, CAN::EpIrq for error passive, CAN::AlIrq for arbitration lost, CAN::BeIrq for bus error)
void attach | ( | T * | obj, |
void(T::*)() | method, | ||
IrqType | type = RxIrq |
||
) | [inherited] |
Attach a member function to call whenever a CAN frame received interrupt is generated.
- Parameters:
-
obj pointer to the object to call the member function on method pointer to the member function to be called event Which CAN interrupt to attach the member function to (CAN::RxIrq for message received, TxIrq for transmitted or aborted, EwIrq for error warning, DoIrq for data overrun, WuIrq for wake-up, EpIrq for error passive, AlIrq for arbitration lost, BeIrq for bus error)
void attach | ( | Callback< void()> | func, |
float | t | ||
) | [inherited] |
void attach | ( | Callback< void()> | func, |
IrqType | type = RxIrq |
||
) | [inherited] |
Attach a function to call whenever a serial interrupt is generated.
- Parameters:
-
func A pointer to a void function, or 0 to set as none type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty)
Definition at line 71 of file SerialBase.cpp.
CANMessage | ( | int | _id, |
CANFormat | _format = CANStandard |
||
) | [inherited] |
CANMessage | ( | int | _id, |
const char * | _data, | ||
char | _len = 8 , |
||
CANType | _type = CANData , |
||
CANFormat | _format = CANStandard |
||
) | [inherited] |
void clear_transfer_buffer | ( | ) | [inherited] |
int close | ( | ) | [protected, virtual, inherited] |
Close the file.
- Returns:
- Zero on success, -1 on error.
Implements FileHandle.
Definition at line 63 of file Stream.cpp.
virtual int close | ( | ) | [pure virtual, inherited] |
void dequeue_transaction | ( | ) | [protected, inherited] |
void destroy | ( | ) | [static, inherited] |
Destroy the current instance of the interrupt manager.
Definition at line 57 of file InterruptManager.cpp.
DigitalIn | ( | PinName | pin, |
PinMode | mode | ||
) | [inherited] |
Create a DigitalIn connected to the specified pin.
- Parameters:
-
pin DigitalIn pin to connect to mode the initial mode of the pin
Definition at line 68 of file DigitalIn.h.
DigitalInOut | ( | PinName | pin, |
PinDirection | direction, | ||
PinMode | mode, | ||
int | value | ||
) | [inherited] |
Create a DigitalInOut connected to the specified pin.
- Parameters:
-
pin DigitalInOut pin to connect to direction the initial direction of the pin mode the initial mode of the pin value the initial value of the pin if is an output
Definition at line 51 of file DigitalInOut.h.
DigitalOut | ( | PinName | pin, |
int | value | ||
) | [inherited] |
Create a DigitalOut connected to the specified pin.
- Parameters:
-
pin DigitalOut pin to connect to value the initial pin value
Definition at line 63 of file DigitalOut.h.
void disable_irq | ( | ) | [inherited] |
Disable IRQ.
This method depends on hw implementation, might disable one port interrupts. For further information, check gpio_irq_disable().
Definition at line 92 of file InterruptIn.cpp.
void enable_irq | ( | ) | [inherited] |
Enable IRQ.
This method depends on hw implementation, might enable one port interrupts. For further information, check gpio_irq_enable().
Definition at line 86 of file InterruptIn.cpp.
void fall | ( | Callback< void()> | func ) | [inherited] |
Attach a function to call when a falling edge occurs on the input.
- Parameters:
-
func A pointer to a void function, or 0 to set as none
Definition at line 65 of file InterruptIn.cpp.
int filter | ( | unsigned int | id, |
unsigned int | mask, | ||
CANFormat | format = CANAny , |
||
int | handle = 0 |
||
) | [inherited] |
void format | ( | int | bits, |
int | mode = 0 |
||
) | [inherited] |
void format | ( | int | bits, |
int | mode = 0 |
||
) | [inherited] |
Configure the data transmission format.
- Parameters:
-
bits Number of bits per SPI frame (4 - 16) mode Clock polarity and phase mode (0 - 3)
mode | POL PHA -----+-------- 0 | 0 0 1 | 0 1 2 | 1 0 3 | 1 1
Definition at line 33 of file SPISlave.cpp.
void format | ( | int | bits = 8 , |
Parity | parity = SerialBase::None , |
||
int | stop_bits = 1 |
||
) | [inherited] |
Set the transmission format used by the serial port.
- Parameters:
-
bits The number of bits in a word (5-8; default = 8) parity The parity used (SerialBase::None, SerialBase::Odd, SerialBase::Even, SerialBase::Forced1, SerialBase::Forced0; default = SerialBase::None) stop The number of stop bits (1 or 2; default = 1)
Definition at line 50 of file SerialBase.cpp.
void frequency | ( | int | hz = 1000000 ) |
[inherited] |
void frequency | ( | int | hz ) | [inherited] |
void frequency | ( | int | hz = 1000000 ) |
[inherited] |
Set the spi bus clock frequency.
- Parameters:
-
hz SCLK frequency in hz (default = 1MHz)
Definition at line 39 of file SPISlave.cpp.
void frequency | ( | int | hz ) | [inherited] |
Set the frequency of the I2C interface.
- Parameters:
-
hz The bus frequency in hertz
Definition at line 28 of file I2CSlave.cpp.
int frequency | ( | int | hz ) | [inherited] |
int fsync | ( | ) | [protected, virtual, inherited] |
Flush any buffers associated with the FileHandle, ensuring it is up to date on disk.
- Returns:
- 0 on success or un-needed, -1 on error
Implements FileHandle.
Definition at line 105 of file Stream.cpp.
virtual int fsync | ( | ) | [pure virtual, inherited] |
Flush any buffers associated with the FileHandle, ensuring it is up to date on disk.
- Returns:
- 0 on success or un-needed, -1 on error
Implemented in Stream.
int getc | ( | ) | [inherited] |
Read a char from the serial port.
- Returns:
- The char read from the serial port
Definition at line 30 of file RawSerial.cpp.
I2C | ( | PinName | sda, |
PinName | scl | ||
) | [inherited] |
I2CSlave | ( | PinName | sda, |
PinName | scl | ||
) | [inherited] |
Create an I2C Slave interface, connected to the specified pins.
Definition at line 22 of file I2CSlave.cpp.
void input | ( | ) | [inherited] |
Set as an input.
Definition at line 87 of file DigitalInOut.h.
void input | ( | ) | [inherited] |
Set as an input.
Definition at line 86 of file BusInOut.cpp.
void input | ( | ) | [inherited] |
Set as an input.
Definition at line 75 of file PortInOut.h.
void irq | ( | uint32_t | id ) | [static, inherited] |
The handler registered with the underlying timer interrupt.
Definition at line 33 of file TimerEvent.cpp.
void irq_handler_asynch | ( | void | ) | [protected, inherited] |
int is_connected | ( | ) | [inherited] |
Return the output setting, represented as 0 or 1 (int)
- Returns:
- Non zero value if pin is connected to uc GPIO 0 if gpio object was initialized with NC
Definition at line 109 of file DigitalInOut.h.
int is_connected | ( | ) | [inherited] |
Return the output setting, represented as 0 or 1 (int)
- Returns:
- Non zero value if pin is connected to uc GPIO 0 if gpio object was initialized with NC
Definition at line 99 of file DigitalIn.h.
int is_connected | ( | ) | [inherited] |
Return the output setting, represented as 0 or 1 (int)
- Returns:
- Non zero value if pin is connected to uc GPIO 0 if gpio object was initialized with NC
Definition at line 95 of file DigitalOut.h.
int isatty | ( | ) | [protected, virtual, inherited] |
Check if the handle is for a interactive terminal device.
If so, line buffered behaviour is used by default
- Returns:
- 1 if it is a terminal, 0 otherwise
Implements FileHandle.
Definition at line 101 of file Stream.cpp.
virtual int isatty | ( | ) | [pure virtual, inherited] |
Check if the handle is for a interactive terminal device.
If so, line buffered behaviour is used by default
- Returns:
- 1 if it is a terminal, 0 otherwise
Implemented in Stream.
int link | ( | ) | [inherited] |
Returns if an ethernet link is pressent or not.
It takes a wile after Ethernet initializion to show up.
- Returns:
- 0 if no ethernet link is pressent, 1 if an ethernet link is pressent.
Example:
// Using the Ethernet link function #include "mbed.h" Ethernet eth; int main() { wait(1); // Needed after startup. if (eth.link()) { printf("online\n"); } else { printf("offline\n"); } }
Definition at line 52 of file Ethernet.cpp.
void lock | ( | void | ) | [virtual, inherited] |
void lock | ( | void | ) | [virtual, inherited] |
virtual void lock | ( | void | ) | [protected, virtual, inherited] |
Acquire exclusive access to this object.
Definition at line 97 of file DirHandle.h.
void lock | ( | void | ) | [protected, virtual, inherited] |
Acquire exclusive access to this serial port.
Reimplemented from SerialBase.
Definition at line 81 of file RawSerial.cpp.
virtual void lock | ( | void | ) | [protected, virtual, inherited] |
Acquire exclusive access to this object.
Reimplemented in Serial.
Definition at line 129 of file FileHandle.h.
void lock | ( | void | ) | [protected, virtual, inherited] |
Acquire exclusive access to this serial port.
Reimplemented from SerialBase.
Definition at line 39 of file Serial.cpp.
void lock | ( | void | ) | [protected, virtual, inherited] |
Acquire exclusive access to this serial port.
Reimplemented in RawSerial, and Serial.
Definition at line 118 of file SerialBase.cpp.
off_t lseek | ( | off_t | offset, |
int | whence | ||
) | [protected, virtual, inherited] |
Move the file position to a given offset from a given location.
- Parameters:
-
offset The offset from whence to move to whence SEEK_SET for the start of the file, SEEK_CUR for the current file position, or SEEK_END for the end of the file.
- Returns:
- new file position on success, -1 on failure or unsupported
Implements FileHandle.
Definition at line 97 of file Stream.cpp.
virtual off_t lseek | ( | off_t | offset, |
int | whence | ||
) | [pure virtual, inherited] |
Move the file position to a given offset from a given location.
- Parameters:
-
offset The offset from whence to move to whence SEEK_SET for the start of the file, SEEK_CUR for the current file position, or SEEK_END for the end of the file.
- Returns:
- new file position on success, -1 on failure or unsupported
Implemented in Stream.
int mask | ( | ) | [inherited] |
Binary mask of bus pins connected to actual pins (not NC pins) If bus pin is in NC state make corresponding bit will be cleared (set to 0), else bit will be set to 1.
- Returns:
- Binary mask of connected pins
Definition at line 86 of file BusInOut.h.
int mask | ( | ) | [inherited] |
int mask | ( | ) | [inherited] |
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"The attach function does not support cv-qualifiers. Replaced by ""attach(callback(obj, method), t)." | |||
) | [inherited] |
Attach a member function to be called by the Ticker, specifiying the interval in seconds.
- Parameters:
-
obj pointer to the object to call the member function on method pointer to the member function to be called t the time between calls in seconds
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"The rise function does not support cv-qualifiers. Replaced by ""rise(callback(obj, method))." | |||
) | [inherited] |
Attach a member function to call when a rising edge occurs on the input.
- Parameters:
-
obj pointer to the object to call the member function on method pointer to the member function to be called
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"The fall function does not support cv-qualifiers. Replaced by ""fall(callback(obj, method))." | |||
) | [inherited] |
Attach a member function to call when a falling edge occurs on the input.
- Parameters:
-
obj pointer to the object to call the member function on method pointer to the member function to be called
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.1" | , |
"The attach function does not support cv-qualifiers. Replaced by ""attach(callback(obj, method), type)." | |||
) | [inherited] |
Attach a member function to call whenever a serial interrupt is generated.
- Parameters:
-
obj pointer to the object to call the member function on method pointer to the member function to be called type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty)
virtual int mkdir | ( | const char * | name, |
mode_t | mode | ||
) | [virtual, inherited] |
Creates a directory in the filesystem.
- Parameters:
-
name The name of the directory to create. mode The permissions to create the directory with.
- Returns:
- 0 on success, -1 on failure.
Definition at line 101 of file FileSystemLike.h.
void mode | ( | PinMode | pull ) | [inherited] |
Set the input pin mode.
- Parameters:
-
mode PullUp, PullDown, PullNone
Definition at line 96 of file BusInOut.cpp.
int mode | ( | Mode | mode ) | [inherited] |
void mode | ( | PinMode | pull ) | [inherited] |
void mode | ( | PinMode | pull ) | [inherited] |
Set the input pin mode.
- Parameters:
-
mode PullUp, PullDown, PullNone, OpenDrain
Definition at line 97 of file DigitalInOut.h.
void mode | ( | PinMode | pull ) | [inherited] |
Set the input pin mode.
- Parameters:
-
mode PullUp, PullDown, PullNone, OpenDrain
Definition at line 87 of file DigitalIn.h.
void mode | ( | PinMode | mode ) | [inherited] |
void mode | ( | PinMode | mode ) | [inherited] |
Set the input pin mode.
- Parameters:
-
mode PullUp, PullDown, PullNone, OpenDrain
Definition at line 85 of file PortInOut.h.
void monitor | ( | bool | silent ) | [inherited] |
virtual FileHandle* open | ( | const char * | filename, |
int | flags | ||
) | [pure virtual, inherited] |
Opens a file from the filesystem.
- Parameters:
-
filename The name of the file to open. flags One of O_RDONLY, O_WRONLY, or O_RDWR, OR'd with zero or more of O_CREAT, O_TRUNC, or O_APPEND.
- Returns:
- A pointer to a FileHandle object representing the file on success, or NULL on failure.
Implemented in LocalFileSystem.
FileHandle * open | ( | const char * | filename, |
int | flags | ||
) | [virtual, inherited] |
Opens a file from the filesystem.
- Parameters:
-
filename The name of the file to open. flags One of O_RDONLY, O_WRONLY, or O_RDWR, OR'd with zero or more of O_CREAT, O_TRUNC, or O_APPEND.
- Returns:
- A pointer to a FileHandle object representing the file on success, or NULL on failure.
Implements FileSystemLike.
Definition at line 239 of file LocalFileSystem.cpp.
virtual DirHandle* opendir | ( | const char * | name ) | [virtual, inherited] |
Opens a directory in the filesystem and returns a DirHandle representing the directory stream.
- Parameters:
-
name The name of the directory to open.
- Returns:
- A DirHandle representing the directory stream, or NULL on failure.
Reimplemented in LocalFileSystem.
Definition at line 90 of file FileSystemLike.h.
DirHandle * opendir | ( | const char * | name ) | [virtual, inherited] |
Opens a directory in the filesystem and returns a DirHandle representing the directory stream.
- Parameters:
-
name The name of the directory to open.
- Returns:
- A DirHandle representing the directory stream, or NULL on failure.
Reimplemented from FileSystemLike.
Definition at line 267 of file LocalFileSystem.cpp.
operator float | ( | ) | [inherited] |
An operator shorthand for read()
The float() operator can be used as a shorthand for read() to simplify common code sequences
Example:
float x = volume.read(); float x = volume; if(volume.read() > 0.25) { ... } if(volume > 0.25) { ... }
Definition at line 103 of file AnalogIn.h.
operator float | ( | ) | [inherited] |
operator float | ( | ) | [inherited] |
An operator shorthand for read()
Definition at line 121 of file AnalogOut.h.
operator float | ( | ) | [inherited] |
operator int | ( | ) | [inherited] |
A shorthand for read()
Definition at line 125 of file BusInOut.cpp.
operator int | ( | ) | [inherited] |
An operator shorthand for read()
Definition at line 98 of file InterruptIn.cpp.
operator int | ( | ) | [inherited] |
A shorthand for read()
Definition at line 131 of file DigitalInOut.h.
operator int | ( | ) | [inherited] |
A shorthand for read()
Definition at line 117 of file DigitalOut.h.
operator int | ( | ) | [inherited] |
A shorthand for read()
Definition at line 95 of file BusOut.cpp.
operator int | ( | ) | [inherited] |
An operator shorthand for read()
Definition at line 106 of file DigitalIn.h.
operator int | ( | ) | [inherited] |
A shorthand for read()
Definition at line 105 of file PortInOut.h.
BusInOut & operator= | ( | int | v ) | [inherited] |
A shorthand for write()
Definition at line 106 of file BusInOut.cpp.
DigitalInOut& operator= | ( | int | value ) | [inherited] |
A shorthand for write()
Definition at line 116 of file DigitalInOut.h.
AnalogOut& operator= | ( | float | percent ) | [inherited] |
An operator shorthand for write()
Definition at line 107 of file AnalogOut.h.
DigitalOut& operator= | ( | int | value ) | [inherited] |
A shorthand for write()
Definition at line 102 of file DigitalOut.h.
BusOut & operator= | ( | int | v ) | [inherited] |
A shorthand for write()
Definition at line 76 of file BusOut.cpp.
PortInOut& operator= | ( | int | value ) | [inherited] |
A shorthand for write()
Definition at line 93 of file PortInOut.h.
PortOut& operator= | ( | int | value ) | [inherited] |
PwmOut& operator= | ( | float | value ) | [inherited] |
DigitalInOut & operator[] | ( | int | index ) | [inherited] |
Access to particular bit in random-iterator fashion.
Definition at line 118 of file BusInOut.cpp.
DigitalIn & operator[] | ( | int | index ) | [inherited] |
DigitalOut & operator[] | ( | int | index ) | [inherited] |
Access to particular bit in random-iterator fashion.
Definition at line 88 of file BusOut.cpp.
void output | ( | ) | [inherited] |
Set as an output.
Definition at line 67 of file PortInOut.h.
void output | ( | ) | [inherited] |
Set as an output.
Definition at line 79 of file DigitalInOut.h.
void output | ( | ) | [inherited] |
Set as an output.
Definition at line 76 of file BusInOut.cpp.
void period | ( | float | seconds ) | [inherited] |
void period_ms | ( | int | ms ) | [inherited] |
void period_us | ( | int | us ) | [inherited] |
void pulsewidth | ( | float | seconds ) | [inherited] |
void pulsewidth_ms | ( | int | ms ) | [inherited] |
void pulsewidth_us | ( | int | us ) | [inherited] |
int putc | ( | int | c ) | [inherited] |
Write a char to the serial port.
- Parameters:
-
c The char to write
- Returns:
- The written char or -1 if an error occured
Definition at line 37 of file RawSerial.cpp.
int puts | ( | const char * | str ) | [inherited] |
Write a string to the serial port.
- Parameters:
-
str The string to write
- Returns:
- 0 if the write succeeds, EOF for error
Definition at line 44 of file RawSerial.cpp.
int queue_transfer | ( | const void * | tx_buffer, |
int | tx_length, | ||
void * | rx_buffer, | ||
int | rx_length, | ||
unsigned char | bit_width, | ||
const event_callback_t & | callback, | ||
int | event | ||
) | [protected, inherited] |
- Parameters:
-
tx_buffer The TX buffer with data to be transfered. If NULL is passed, the default SPI value is sent tx_length The length of TX buffer in bytes rx_buffer The RX buffer which is used for received data. If NULL is passed, received data are ignored rx_length The length of RX buffer in bytes bit_width The buffers element width callback The event callback function event The logical OR of events to modify
- Returns:
- Zero if a transfer was added to the queue, or -1 if the queue is full
unsigned char rderror | ( | ) | [inherited] |
int read | ( | uint8_t * | buffer, |
int | length, | ||
const event_callback_t & | callback, | ||
int | event = SERIAL_EVENT_RX_COMPLETE , |
||
unsigned char | char_match = SERIAL_RESERVED_CHAR_MATCH |
||
) | [inherited] |
Begin asynchronous reading using 8bit buffer.
The completition invokes registred RX event callback.
- Parameters:
-
buffer The buffer where received data will be stored length The buffer length in bytes callback The event callback function event The logical OR of RX events char_match The matching character
Definition at line 207 of file SerialBase.cpp.
float read | ( | void | ) | [inherited] |
Return the current output voltage setting, measured as a percentage (float)
- Returns:
- A floating-point value representing the current voltage being output on the pin, measured as a percentage. The returned value will lie between 0.0f (representing 0v / 0%) and 1.0f (representing 3.3v / 100%).
- Note:
- This value may not match exactly the value set by a previous write().
Definition at line 98 of file AnalogOut.h.
ssize_t read | ( | void * | buffer, |
size_t | length | ||
) | [protected, virtual, inherited] |
Function read Reads the contents of the file into a buffer.
- Parameters:
-
buffer the buffer to read in to length the number of characters to read
- Returns:
- The number of characters read (zero at end of file) on success, -1 on error.
Implements FileHandle.
Definition at line 82 of file Stream.cpp.
int read | ( | int | ack ) | [inherited] |
int read | ( | int | address, |
char * | data, | ||
int | length, | ||
bool | repeated = false |
||
) | [inherited] |
Read from an I2C slave.
Performs a complete read transaction. The bottom bit of the address is forced to 1 to indicate a read.
- Parameters:
-
address 8-bit I2C slave address [ addr | 1 ] data Pointer to the byte-array to read data in to length Number of bytes to read repeated Repeated start, true - don't send stop at end
- Returns:
- 0 on success (ack), non-0 on failure (nack)
int read | ( | void | ) | [inherited] |
Retrieve data from receive buffer as slave.
- Returns:
- the data in the receive buffer
Definition at line 48 of file SPISlave.cpp.
virtual ssize_t read | ( | void * | buffer, |
size_t | length | ||
) | [pure virtual, inherited] |
Function read Reads the contents of the file into a buffer.
- Parameters:
-
buffer the buffer to read in to length the number of characters to read
- Returns:
- The number of characters read (zero at end of file) on success, -1 on error.
Implemented in Stream.
int read | ( | void | ) | [inherited] |
Read the input, represented as 0 or 1 (int)
- Returns:
- An integer representing the state of the input pin, 0 for logical 0, 1 for logical 1
Definition at line 78 of file DigitalIn.h.
int read | ( | void | ) | [inherited] |
int read | ( | char * | data, |
int | length | ||
) | [inherited] |
Read from an I2C master.
- Parameters:
-
data pointer to the byte array to read data in to length maximum number of bytes to read
- Returns:
- 0 on success, non-0 otherwise
Definition at line 41 of file I2CSlave.cpp.
int read | ( | void | ) | [inherited] |
Read a single byte from an I2C master.
- Returns:
- the byte read
Definition at line 45 of file I2CSlave.cpp.
int read | ( | uint16_t * | buffer, |
int | length, | ||
const event_callback_t & | callback, | ||
int | event = SERIAL_EVENT_RX_COMPLETE , |
||
unsigned char | char_match = SERIAL_RESERVED_CHAR_MATCH |
||
) | [inherited] |
Begin asynchronous reading using 16bit buffer.
The completition invokes registred RX event callback.
- Parameters:
-
buffer The buffer where received data will be stored length The buffer length in bytes callback The event callback function event The logical OR of RX events char_match The matching character
Definition at line 217 of file SerialBase.cpp.
float read | ( | void | ) | [inherited] |
int read | ( | void | ) | [inherited] |
Read the input, represented as 0 or 1 (int)
- Returns:
- An integer representing the state of the input pin, 0 for logical 0, 1 for logical 1
Definition at line 42 of file InterruptIn.cpp.
int read | ( | void | ) | [inherited] |
Return the output setting, represented as 0 or 1 (int)
- Returns:
- an integer representing the output setting of the pin, 0 for logical 0, 1 for logical 1
Definition at line 84 of file DigitalOut.h.
int read | ( | void | ) | [inherited] |
Read the value currently output on the bus.
- Returns:
- An integer with each bit corresponding to associated DigitalOut pin setting
Definition at line 64 of file BusOut.cpp.
float read | ( | void | ) | [inherited] |
Read the input voltage, represented as a float in the range [0.0, 1.0].
- Returns:
- A floating-point value representing the current input voltage, measured as a percentage
Definition at line 71 of file AnalogIn.h.
int read | ( | void | ) | [inherited] |
int read | ( | char * | data, |
int | size | ||
) | [inherited] |
Read from an recevied ethernet packet.
After receive returnd a number bigger than 0it is possible to read bytes from this packet. Read will write up to size bytes into data.
It is possible to use read multible times. Each time read will start reading after the last read byte before.
- Returns:
- The number of byte read.
Definition at line 44 of file Ethernet.cpp.
int read | ( | void | ) | [inherited] |
Read the value currently output on the port.
- Returns:
- An integer with each bit corresponding to associated port pin setting
Definition at line 61 of file PortInOut.h.
int read | ( | void | ) | [inherited] |
float read | ( | void | ) | [inherited] |
Return the current output duty-cycle setting, measured as a percentage (float)
- Returns:
- A floating-point value representing the current duty-cycle being output on the pin, measured as a percentage. The returned value will lie between 0.0f (representing on 0%) and 1.0f (representing on 100%).
- Note:
- This value may not match exactly the value set by a previous <write>.
int read | ( | void | ) | [inherited] |
Return the output setting, represented as 0 or 1 (int)
- Returns:
- an integer representing the output setting of the pin if it is an output, or read the input if set as an input
Definition at line 72 of file DigitalInOut.h.
int read | ( | CANMessage & | msg, |
int | handle = 0 |
||
) | [inherited] |
Read a CANMessage from the bus.
- Parameters:
-
msg A CANMessage to read to. handle message filter handle (0 for any message)
- Returns:
- 0 if no message arrived, 1 if message arrived
int read | ( | void | ) | [inherited] |
Read the value currently output on the bus.
- Returns:
- An integer with each bit corresponding to associated DigitalInOut pin setting
Definition at line 64 of file BusInOut.cpp.
int read_ms | ( | ) | [inherited] |
unsigned short read_u16 | ( | ) | [inherited] |
Read the input voltage, represented as an unsigned short in the range [0x0, 0xFFFF].
- Returns:
- 16-bit unsigned short representing the current input voltage, normalised to a 16-bit value
Definition at line 83 of file AnalogIn.h.
int read_us | ( | ) | [inherited] |
int readable | ( | ) | [inherited] |
Determine if there is a character available to read.
- Returns:
- 1 if there is a character available to read, 0 otherwise
Definition at line 56 of file SerialBase.cpp.
virtual struct dirent* readdir | ( | ) | [read, pure virtual, inherited] |
Return the directory entry at the current position, and advances the position to the next entry.
- Returns:
- A pointer to a dirent structure representing the directory entry at the current position, or NULL on reaching end of directory or error.
int receive | ( | void | ) | [inherited] |
Polls the SPI to see if data has been received.
- Returns:
- 0 if no data, 1 otherwise
Definition at line 44 of file SPISlave.cpp.
int receive | ( | void | ) | [inherited] |
Checks to see if this I2C Slave has been addressed.
- Returns:
- A status indicating if the device has been addressed, and how
- NoData - the slave has not been addressed
- ReadAddressed - the master has requested a read from this slave
- WriteAddressed - the master is writing to this slave
- WriteGeneral - the master is writing to all slave
Definition at line 37 of file I2CSlave.cpp.
int receive | ( | void | ) | [inherited] |
Recevies an arrived ethernet packet.
Receiving an ethernet packet will drop the last received ethernet packet and make a new ethernet packet ready to read. If no ethernet packet is arrived it will return 0.
- Returns:
- 0 if no ethernet packet is arrived, or the size of the arrived packet.
Definition at line 40 of file Ethernet.cpp.
virtual int remove | ( | const char * | filename ) | [virtual, inherited] |
Remove a file from the filesystem.
- Parameters:
-
filename the name of the file to remove. returns 0 on success, -1 on failure.
Reimplemented in LocalFileSystem.
Definition at line 68 of file FileSystemLike.h.
int remove | ( | const char * | filename ) | [virtual, inherited] |
Remove a file from the filesystem.
- Parameters:
-
filename the name of the file to remove. returns 0 on success, -1 on failure.
Reimplemented from FileSystemLike.
Definition at line 261 of file LocalFileSystem.cpp.
bool remove_handler | ( | pFunctionPointer_t | handler, |
IRQn_Type | irq | ||
) | [inherited] |
Remove a handler from an interrupt.
- Parameters:
-
handler the function object for the handler to remove irq the interrupt number
- Returns:
- true if the handler was found and removed, false otherwise
Definition at line 99 of file InterruptManager.cpp.
virtual int rename | ( | const char * | oldname, |
const char * | newname | ||
) | [virtual, inherited] |
Rename a file in the filesystem.
- Parameters:
-
oldname the name of the file to rename. newname the name to rename it to.
- Returns:
- 0 on success, -1 on failure.
Definition at line 79 of file FileSystemLike.h.
void reply | ( | int | value ) | [inherited] |
Fill the transmission buffer with the value to be written out as slave on the next received message from the master.
- Parameters:
-
value the data to be transmitted next
Definition at line 52 of file SPISlave.cpp.
void reset | ( | ) | [inherited] |
void reset | ( | ) | [inherited] |
virtual void rewinddir | ( | ) | [pure virtual, inherited] |
Resets the position to the beginning of the directory.
void rise | ( | Callback< void()> | func ) | [inherited] |
Attach a function to call when a rising edge occurs on the input.
- Parameters:
-
func A pointer to a void function, or 0 to set as none
Definition at line 53 of file InterruptIn.cpp.
virtual void seekdir | ( | off_t | location ) | [virtual, inherited] |
Sets the position of the DirHandle.
- Parameters:
-
location The location to seek to. Must be a value returned by telldir.
Definition at line 89 of file DirHandle.h.
int send | ( | ) | [inherited] |
Send an outgoing ethernet packet.
After filling in the data in an ethernet packet it must be send. Send will provide a new packet to write to.
- Returns:
- 0 if the sending was failed, or the size of the packet successfully sent.
Definition at line 36 of file Ethernet.cpp.
Serial | ( | PinName | tx, |
PinName | rx, | ||
int | baud | ||
) | [inherited] |
Create a Serial port, connected to the specified transmit and receive pins, with the specified baud.
- Parameters:
-
tx Transmit pin rx Receive pin baud The baud rate of the serial port
- Note:
- Either tx or rx may be specified as NC if unused
Definition at line 26 of file Serial.cpp.
Serial | ( | PinName | tx, |
PinName | rx, | ||
const char * | name = NULL , |
||
int | baud = MBED_CONF_PLATFORM_DEFAULT_SERIAL_BAUD_RATE |
||
) | [inherited] |
Create a Serial port, connected to the specified transmit and receive pins.
- Parameters:
-
tx Transmit pin rx Receive pin name The name of the stream associated with this serial port (optional) baud The baud rate of the serial port (optional, defaults to MBED_CONF_PLATFORM_DEFAULT_SERIAL_BAUD_RATE)
- Note:
- Either tx or rx may be specified as NC if unused
Definition at line 23 of file Serial.cpp.
int set_dma_usage | ( | DMAUsage | usage ) | [inherited] |
int set_dma_usage_rx | ( | DMAUsage | usage ) | [inherited] |
Configure DMA usage suggestion for non-blocking RX transfers.
- Parameters:
-
usage The usage DMA hint for peripheral
- Returns:
- Zero if the usage was set, -1 if a transaction is on-going
Definition at line 198 of file SerialBase.cpp.
int set_dma_usage_tx | ( | DMAUsage | usage ) | [inherited] |
Configure DMA usage suggestion for non-blocking TX transfers.
- Parameters:
-
usage The usage DMA hint for peripheral
- Returns:
- Zero if the usage was set, -1 if a transaction is on-going
Definition at line 189 of file SerialBase.cpp.
void set_flow_control | ( | Flow | type, |
PinName | flow1 = NC , |
||
PinName | flow2 = NC |
||
) | [inherited] |
Set the flow control type on the serial port.
- Parameters:
-
type the flow control type (Disabled, RTS, CTS, RTSCTS) flow1 the first flow control pin (RTS for RTS or RTSCTS, CTS for CTS) flow2 the second flow control pin (CTS for RTSCTS)
Definition at line 127 of file SerialBase.cpp.
void set_link | ( | Mode | mode ) | [inherited] |
Sets the speed and duplex parameters of an ethernet link.
- AutoNegotiate Auto negotiate speed and duplex
- HalfDuplex10 10 Mbit, half duplex
- FullDuplex10 10 Mbit, full duplex
- HalfDuplex100 100 Mbit, half duplex
- FullDuplex100 100 Mbit, full duplex
- Parameters:
-
mode the speed and duplex mode to set the link to:
Definition at line 56 of file Ethernet.cpp.
void start | ( | void | ) | [inherited] |
void start_transaction | ( | transaction_t * | data ) | [protected, inherited] |
void start_transfer | ( | const void * | tx_buffer, |
int | tx_length, | ||
void * | rx_buffer, | ||
int | rx_length, | ||
unsigned char | bit_width, | ||
const event_callback_t & | callback, | ||
int | event | ||
) | [protected, inherited] |
Configures a callback, spi peripheral and initiate a new transfer.
- Parameters:
-
tx_buffer The TX buffer with data to be transfered. If NULL is passed, the default SPI value is sent tx_length The length of TX buffer in bytes rx_buffer The RX buffer which is used for received data. If NULL is passed, received data are ignored rx_length The length of RX buffer in bytes bit_width The buffers element width callback The event callback function event The logical OR of events to modify
void stop | ( | void | ) | [inherited] |
void stop | ( | void | ) | [inherited] |
Reset the I2C slave back into the known ready receiving state.
Definition at line 57 of file I2CSlave.cpp.
unsigned char tderror | ( | ) | [inherited] |
virtual off_t telldir | ( | ) | [virtual, inherited] |
Returns the current position of the DirHandle.
- Returns:
- the current position, -1 on error.
Definition at line 83 of file DirHandle.h.
int transfer | ( | const Type * | tx_buffer, |
int | tx_length, | ||
Type * | rx_buffer, | ||
int | rx_length, | ||
const event_callback_t & | callback, | ||
int | event = SPI_EVENT_COMPLETE |
||
) | [inherited] |
Start non-blocking SPI transfer using 8bit buffers.
- Parameters:
-
tx_buffer The TX buffer with data to be transfered. If NULL is passed, the default SPI value is sent tx_length The length of TX buffer in bytes rx_buffer The RX buffer which is used for received data. If NULL is passed, received data are ignored rx_length The length of RX buffer in bytes callback The event callback function event The logical OR of events to modify. Look at spi hal header file for SPI events.
- Returns:
- Zero if the transfer has started, or -1 if SPI peripheral is busy
int transfer | ( | const void * | tx_buffer, |
int | tx_length, | ||
void * | rx_buffer, | ||
int | rx_length, | ||
unsigned char | bit_width, | ||
const event_callback_t & | callback, | ||
int | event | ||
) | [protected, inherited] |
Common transfer method.
- Parameters:
-
tx_buffer The TX buffer with data to be transfered. If NULL is passed, the default SPI value is sent tx_length The length of TX buffer in bytes rx_buffer The RX buffer which is used for received data. If NULL is passed, received data are ignored rx_length The length of RX buffer in bytes bit_width The buffers element width callback The event callback function event The logical OR of events to modify
- Returns:
- Zero if the transfer has started or was added to the queue, or -1 if SPI peripheral is busy/buffer is full
int transfer | ( | int | address, |
const char * | tx_buffer, | ||
int | tx_length, | ||
char * | rx_buffer, | ||
int | rx_length, | ||
const event_callback_t & | callback, | ||
int | event = I2C_EVENT_TRANSFER_COMPLETE , |
||
bool | repeated = false |
||
) | [inherited] |
Start non-blocking I2C transfer.
- Parameters:
-
address 8/10 bit I2c slave address tx_buffer The TX buffer with data to be transfered tx_length The length of TX buffer in bytes rx_buffer The RX buffer which is used for received data rx_length The length of RX buffer in bytes event The logical OR of events to modify callback The event callback function repeated Repeated start, true - do not send stop at end
- Returns:
- Zero if the transfer has started, or -1 if I2C peripheral is busy
void unlock | ( | void | ) | [virtual, inherited] |
virtual void unlock | ( | void | ) | [protected, virtual, inherited] |
Release exclusive access to this object.
Definition at line 103 of file DirHandle.h.
void unlock | ( | void | ) | [protected, virtual, inherited] |
Release exclusive access to this serial port.
Reimplemented from SerialBase.
Definition at line 43 of file Serial.cpp.
void unlock | ( | void | ) | [virtual, inherited] |
virtual void unlock | ( | void | ) | [protected, virtual, inherited] |
Release exclusive access to this object.
Reimplemented in Serial.
Definition at line 135 of file FileHandle.h.
void unlock | ( | void | ) | [protected, virtual, inherited] |
Release exclusive access to this serial port.
Reimplemented from SerialBase.
Definition at line 87 of file RawSerial.cpp.
void unlock | ( | void | ) | [protected, virtual, inherited] |
Release exclusive access to this serial port.
Reimplemented in RawSerial, and Serial.
Definition at line 122 of file SerialBase.cpp.
int write | ( | int | data ) | [inherited] |
int write | ( | const uint8_t * | buffer, |
int | length, | ||
const event_callback_t & | callback, | ||
int | event = SERIAL_EVENT_TX_COMPLETE |
||
) | [inherited] |
Begin asynchronous write using 8bit buffer.
The completition invokes registered TX event callback
- Parameters:
-
buffer The buffer where received data will be stored length The buffer length in bytes callback The event callback function event The logical OR of TX events
Definition at line 153 of file SerialBase.cpp.
int write | ( | int | address, |
const char * | data, | ||
int | length, | ||
bool | repeated = false |
||
) | [inherited] |
Write to an I2C slave.
Performs a complete write transaction. The bottom bit of the address is forced to 0 to indicate a write.
- Parameters:
-
address 8-bit I2C slave address [ addr | 0 ] data Pointer to the byte-array data to send length Number of bytes to send repeated Repeated start, true - do not send stop at end
- Returns:
- 0 or non-zero - written number of bytes, negative - I2C_ERROR_XXX status
void write | ( | int | value ) | [inherited] |
Write the value to the output bus.
- Parameters:
-
value An integer specifying a bit to write for every corresponding DigitalInOut pin
Definition at line 54 of file BusInOut.cpp.
int write | ( | const char * | data, |
int | length | ||
) | [inherited] |
Write to an I2C master.
- Parameters:
-
data pointer to the byte array to be transmitted length the number of bytes to transmite
- Returns:
- 0 on success, non-0 otherwise
Definition at line 49 of file I2CSlave.cpp.
void write | ( | int | value ) | [inherited] |
Set the output, specified as 0 or 1 (int)
- Parameters:
-
value An integer specifying the pin output value, 0 for logical 0, 1 (or any other non-zero value) for logical 1
Definition at line 61 of file DigitalInOut.h.
void write | ( | int | value ) | [inherited] |
Set the output, specified as 0 or 1 (int)
- Parameters:
-
value An integer specifying the pin output value, 0 for logical 0, 1 (or any other non-zero value) for logical 1
Definition at line 73 of file DigitalOut.h.
int write | ( | int | value ) | [virtual, inherited] |
void write | ( | float | value ) | [inherited] |
Set the ouput duty-cycle, specified as a percentage (float)
- Parameters:
-
value A floating-point value representing the output duty-cycle, specified as a percentage. The value should lie between 0.0f (representing on 0%) and 1.0f (representing on 100%). Values outside this range will be saturated to 0.0f or 1.0f.
void write | ( | int | value ) | [inherited] |
Write the value to the output port.
- Parameters:
-
value An integer specifying a bit to write for every corresponding port pin
Definition at line 52 of file PortInOut.h.
void write | ( | float | value ) | [inherited] |
Set the output voltage, specified as a percentage (float)
- Parameters:
-
value A floating-point value representing the output voltage, specified as a percentage. The value should lie between 0.0f (representing 0v / 0%) and 1.0f (representing 3.3v / 100%). Values outside this range will be saturated to 0.0f or 1.0f.
Definition at line 71 of file AnalogOut.h.
int write | ( | CANMessage | msg ) | [inherited] |
Write a CANMessage to the bus.
- Parameters:
-
msg The CANMessage to write.
- Returns:
- 0 if write failed, 1 if write was successful
void write | ( | int | value ) | [inherited] |
void write | ( | int | value ) | [inherited] |
Write the value to the output bus.
- Parameters:
-
value An integer specifying a bit to write for every corresponding DigitalOut pin
Definition at line 54 of file BusOut.cpp.
int write | ( | const uint16_t * | buffer, |
int | length, | ||
const event_callback_t & | callback, | ||
int | event = SERIAL_EVENT_TX_COMPLETE |
||
) | [inherited] |
Begin asynchronous write using 16bit buffer.
The completition invokes registered TX event callback
- Parameters:
-
buffer The buffer where received data will be stored length The buffer length in bytes callback The event callback function event The logical OR of TX events
Definition at line 162 of file SerialBase.cpp.
ssize_t write | ( | const void * | buffer, |
size_t | length | ||
) | [protected, virtual, inherited] |
Write the contents of a buffer to the file.
- Parameters:
-
buffer the buffer to write from length the number of characters to write
- Returns:
- The number of characters written (possibly 0) on success, -1 on error.
Implements FileHandle.
Definition at line 67 of file Stream.cpp.
int write | ( | int | data ) | [inherited] |
Write a single byte to an I2C master.
the byte to write
- Returns:
- '1' if an ACK was received, '0' otherwise
Definition at line 53 of file I2CSlave.cpp.
int write | ( | const char * | data, |
int | size | ||
) | [inherited] |
Writes into an outgoing ethernet packet.
It will append size bytes of data to the previously written bytes.
- Parameters:
-
data An array to write. size The size of data.
- Returns:
- The number of written bytes.
Definition at line 32 of file Ethernet.cpp.
void write_u16 | ( | unsigned short | value ) | [inherited] |
Set the output voltage, represented as an unsigned short in the range [0x0, 0xFFFF].
- Parameters:
-
value 16-bit unsigned short representing the output voltage, normalised to a 16-bit value (0x0000 = 0v, 0xFFFF = 3.3v)
Definition at line 82 of file AnalogOut.h.
int writeable | ( | ) | [inherited] |
Determine if there is space available to write a character.
- Returns:
- 1 if there is space to write a character, 0 otherwise
Definition at line 64 of file SerialBase.cpp.
~Ethernet | ( | ) | [virtual, inherited] |
Powers the hardware down.
Definition at line 28 of file Ethernet.cpp.
~TimerEvent | ( | ) | [virtual, inherited] |
Destruction removes it...
Definition at line 38 of file TimerEvent.cpp.
Variable Documentation
timestamp_t _delay [protected, inherited] |
Callback<void()> _function [protected, inherited] |
int _nc_mask [protected, inherited] |
Mask of bus's NC pins If bit[n] is set to 1 - pin is connected if bit[n] is cleared - pin is not connected (NC)
Definition at line 113 of file BusInOut.h.
int _nc_mask [protected, inherited] |
Generated on Tue Jul 12 2022 15:10:59 by
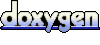