This is the final version of Mini Gateway for Automation and Security desgined for Renesas GR Peach Design Contest
Dependencies: GR-PEACH_video GraphicsFramework HTTPServer R_BSP mbed-rpc mbed-rtos Socket lwip-eth lwip-sys lwip FATFileSystem
Fork of mbed-os-example-mbed5-blinky by
FileSystemLike.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_FILESYSTEMLIKE_H 00017 #define MBED_FILESYSTEMLIKE_H 00018 00019 #include "platform/platform.h" 00020 00021 #include "drivers/FileBase.h" 00022 #include "drivers/FileHandle.h" 00023 #include "drivers/DirHandle.h" 00024 00025 namespace mbed { 00026 /** \addtogroup drivers */ 00027 /** @{*/ 00028 00029 /** A filesystem-like object is one that can be used to open files 00030 * though it by fopen("/name/filename", mode) 00031 * 00032 * Implementations must define at least open (the default definitions 00033 * of the rest of the functions just return error values). 00034 * 00035 * @Note Synchronization level: Set by subclass 00036 */ 00037 class FileSystemLike : public FileBase { 00038 00039 public: 00040 /** FileSystemLike constructor 00041 * 00042 * @param name The name to use for the filesystem. 00043 */ 00044 FileSystemLike(const char *name); 00045 00046 virtual ~FileSystemLike(); 00047 00048 static DirHandle *opendir(); 00049 friend class BaseDirHandle; 00050 00051 /** Opens a file from the filesystem 00052 * 00053 * @param filename The name of the file to open. 00054 * @param flags One of O_RDONLY, O_WRONLY, or O_RDWR, OR'd with 00055 * zero or more of O_CREAT, O_TRUNC, or O_APPEND. 00056 * 00057 * @returns 00058 * A pointer to a FileHandle object representing the 00059 * file on success, or NULL on failure. 00060 */ 00061 virtual FileHandle *open(const char *filename, int flags) = 0; 00062 00063 /** Remove a file from the filesystem. 00064 * 00065 * @param filename the name of the file to remove. 00066 * @param returns 0 on success, -1 on failure. 00067 */ 00068 virtual int remove(const char *filename) { (void) filename; return -1; }; 00069 00070 /** Rename a file in the filesystem. 00071 * 00072 * @param oldname the name of the file to rename. 00073 * @param newname the name to rename it to. 00074 * 00075 * @returns 00076 * 0 on success, 00077 * -1 on failure. 00078 */ 00079 virtual int rename(const char *oldname, const char *newname) { (void) oldname, (void) newname; return -1; }; 00080 00081 /** Opens a directory in the filesystem and returns a DirHandle 00082 * representing the directory stream. 00083 * 00084 * @param name The name of the directory to open. 00085 * 00086 * @returns 00087 * A DirHandle representing the directory stream, or 00088 * NULL on failure. 00089 */ 00090 virtual DirHandle *opendir(const char *name) { (void) name; return NULL; }; 00091 00092 /** Creates a directory in the filesystem. 00093 * 00094 * @param name The name of the directory to create. 00095 * @param mode The permissions to create the directory with. 00096 * 00097 * @returns 00098 * 0 on success, 00099 * -1 on failure. 00100 */ 00101 virtual int mkdir(const char *name, mode_t mode) { (void) name, (void) mode; return -1; } 00102 00103 // TODO other filesystem functions (mkdir, rm, rn, ls etc) 00104 }; 00105 00106 } // namespace mbed 00107 00108 #endif 00109 00110 /** @}*/
Generated on Tue Jul 12 2022 15:10:51 by
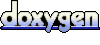