A library for STMicroelectronics STTS751 I2C temperature sensor
STTS751 Class Reference
A library for STMicroelectronics STTS751 I2C temperature sensor. More...
#include <STTS751.h>
Public Types | |
enum | Model { MODEL_0 = 0x00, MODEL_1 = 0x40 } |
Device models. More... | |
enum | Address { ADDRESS_0 = (0x48 << 1), ADDRESS_1 = (0x49 << 1), ADDRESS_2 = (0x38 << 1), ADDRESS_3 = (0x39 << 1) } |
I2C slave address selectors The I2C slave address of the device is determined by the pull up register for Addr/~Therm pin. More... | |
enum | Resolution { RES_9 = 0x08, RES_10 = 0x00, RES_11 = 0x04, RES_12 = 0x0c } |
Temperature resolutions. More... | |
Public Member Functions | |
STTS751 (PinName sda, PinName scl, bool standby=false, Address addr=ADDRESS_3, Model model=MODEL_0) | |
Create an STTS751 object connected to the specified I2C pins. | |
STTS751 (I2C &_i2c, bool standby=false, Address addr=ADDRESS_3, Model model=MODEL_0) | |
Create an STTS751 object connected to the specified I2C port. | |
void | init () |
Initialize the device. | |
int | addr () |
The I2C slave address (8bit) of the device. | |
Resolution | resolution () |
Get the current temperature resolution. | |
void | setResolution (Resolution res=RES_10) |
Set the temperature resolution. | |
int | conversionRate () |
Get the current conversion rate. | |
void | setConversionRate (int rate) |
Set the conversion rate Set the number of times the temperature value is updated each second. | |
void | setStandbyMode (bool standby) |
Set the device mode. | |
void | start () |
Start a temperature conversion (in standby mode) | |
bool | ready () |
Checks that a temperature conversion has finished and the temperature value is available. | |
float | temp (bool nowait=false) |
Obtain the current temperature measurement. | |
operator float () | |
A shorthand for temp() | |
Protected Types | |
enum | |
I2C registers. More... | |
enum | |
Configuration register (default 0x00)
| |
enum | |
Status Register (default undefined)
| |
enum | |
Conversion Rate Register (default 4)
|
Detailed Description
A library for STMicroelectronics STTS751 I2C temperature sensor.
http://www.st.com/web/catalog/sense_power/FM89/SC294/PF220116
Example:
#include "mbed.h" #include "STTS751.h" // I2C pins: p9 = sda, p10 = scl STTS751 sensor(p9, p10); // You can specify an I2C object instead. // I2C i2c(p2, p10); // STTS751 sensor(i2c); int main() { // set the temperature resolution to 12bits sensor.setResolution(STTS751::RES_12); while (true) { printf("tmp: %.4f\n", (float)sensor); wait(1); } }
Definition at line 48 of file STTS751.h.
Member Enumeration Documentation
anonymous enum [protected] |
anonymous enum [protected] |
anonymous enum [protected] |
enum Address |
enum Model |
enum Resolution |
Constructor & Destructor Documentation
STTS751 | ( | PinName | sda, |
PinName | scl, | ||
bool | standby = false , |
||
Address | addr = ADDRESS_3 , |
||
Model | model = MODEL_0 |
||
) |
Create an STTS751 object connected to the specified I2C pins.
- Parameters:
-
sda I2C data pin scl I2C clock pin standby Standby mode (defaults to false) addr I2C slave address (defaults to ADDRESS_3) model Device model (defaults to MODEL_0)
Definition at line 21 of file STTS751.cpp.
Create an STTS751 object connected to the specified I2C port.
- Parameters:
-
i2c I2C port standby Standby mode (defaults to false) addr I2C slave address (defaults to ADDRESS_3) model Device model (defaults to MODEL_0)
Definition at line 26 of file STTS751.cpp.
Member Function Documentation
int addr | ( | ) |
The I2C slave address (8bit) of the device.
- Returns:
- The I2C slave address (8bit) of the device
Definition at line 40 of file STTS751.cpp.
int conversionRate | ( | ) |
Get the current conversion rate.
- Returns:
- The current conversion rate
Definition at line 55 of file STTS751.cpp.
void init | ( | ) |
Initialize the device.
Definition at line 31 of file STTS751.cpp.
operator float | ( | ) |
A shorthand for temp()
- Returns:
- The current temperature measurement in Celsius.
Definition at line 96 of file STTS751.cpp.
bool ready | ( | ) |
Checks that a temperature conversion has finished and the temperature value is available.
- Returns:
- true if a temperature conversion has finished and the temperature value is available
Definition at line 79 of file STTS751.cpp.
STTS751::Resolution resolution | ( | ) |
Get the current temperature resolution.
- Returns:
- The current temperature resolution
Definition at line 44 of file STTS751.cpp.
void setConversionRate | ( | int | rate ) |
Set the conversion rate Set the number of times the temperature value is updated each second.
The conversion rate (times/second) can be calculated by 2^(rate - 4). Note that rate should be less than 8 if the temperature resolution is 12-bit and should be less than 9 if the temperature resolution is greater than 10-bit
- Parameters:
-
rate Conversion rate (0 .. 9)
Definition at line 60 of file STTS751.cpp.
void setResolution | ( | STTS751::Resolution | res = RES_10 ) |
Set the temperature resolution.
- Parameters:
-
res Resolution (defaults to RES_10)
Definition at line 49 of file STTS751.cpp.
void setStandbyMode | ( | bool | standby ) |
Set the device mode.
- Parameters:
-
standby true : standby mode, false : continuous conversion mode
Definition at line 64 of file STTS751.cpp.
void start | ( | ) |
Start a temperature conversion (in standby mode)
Definition at line 74 of file STTS751.cpp.
float temp | ( | bool | nowait = false ) |
Obtain the current temperature measurement.
- Parameters:
-
nowait If true, read the temperature value without waiting for finishing a conversion (in standby mode)
- Returns:
- The current temperature measurement in Celsius.
Definition at line 84 of file STTS751.cpp.
Generated on Fri Jul 15 2022 05:08:55 by
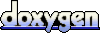