A library for STMicroelectronics STTS751 I2C temperature sensor
Embed:
(wiki syntax)
Show/hide line numbers
STTS751.h
00001 /* A library for STMicroelectronics STTS751 I2C temperature sensor 00002 * Copyright 2014, Takuo WATANABE (wtakuo) 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef STTS751_H 00018 #define STTS751_H 00019 00020 #include "mbed.h" 00021 00022 /** A library for STMicroelectronics STTS751 I2C temperature sensor 00023 * 00024 * http://www.st.com/web/catalog/sense_power/FM89/SC294/PF220116 00025 * 00026 * Example: 00027 * @code 00028 * #include "mbed.h" 00029 * #include "STTS751.h" 00030 * 00031 * // I2C pins: p9 = sda, p10 = scl 00032 * STTS751 sensor(p9, p10); 00033 * 00034 * // You can specify an I2C object instead. 00035 * // I2C i2c(p2, p10); 00036 * // STTS751 sensor(i2c); 00037 * 00038 * int main() { 00039 * // set the temperature resolution to 12bits 00040 * sensor.setResolution(STTS751::RES_12); 00041 * while (true) { 00042 * printf("tmp: %.4f\n", (float)sensor); 00043 * wait(1); 00044 * } 00045 * } 00046 * @endcode 00047 */ 00048 class STTS751 { 00049 public: 00050 /** Device models 00051 */ 00052 enum Model { 00053 MODEL_0 = 0x00, /**< Model 0 (default) */ 00054 MODEL_1 = 0x40 /**< Model 1 */ 00055 }; 00056 00057 /** I2C slave address selectors 00058 * The I2C slave address of the device is determined by the pull up register for Addr/~Therm pin. 00059 * 00060 */ 00061 enum Address { 00062 ADDRESS_0 = (0x48 << 1), /**< pull up resistor = 7.5K */ 00063 ADDRESS_1 = (0x49 << 1), /**< pull up resistor = 12K */ 00064 ADDRESS_2 = (0x38 << 1), /**< pull up resistor = 20K */ 00065 ADDRESS_3 = (0x39 << 1) /**< pull up resistor = 33K or GND (default) */ 00066 }; 00067 00068 /** Temperature resolutions 00069 */ 00070 enum Resolution { 00071 RES_9 = 0x08, /**< 9 bits */ 00072 RES_10 = 0x00, /**< 10 bits (default) */ 00073 RES_11 = 0x04, /**< 11 bits */ 00074 RES_12 = 0x0c /**< 12 bits */ 00075 }; 00076 00077 /** Create an STTS751 object connected to the specified I2C pins. 00078 * 00079 * @param sda I2C data pin 00080 * @param scl I2C clock pin 00081 * @param standby Standby mode (defaults to false) 00082 * @param addr I2C slave address (defaults to ADDRESS_3) 00083 * @param model Device model (defaults to MODEL_0) 00084 */ 00085 STTS751(PinName sda, PinName scl, 00086 bool standby = false, Address addr = ADDRESS_3, Model model = MODEL_0); 00087 00088 00089 /** Create an STTS751 object connected to the specified I2C port. 00090 * 00091 * @param i2c I2C port 00092 * @param standby Standby mode (defaults to false) 00093 * @param addr I2C slave address (defaults to ADDRESS_3) 00094 * @param model Device model (defaults to MODEL_0) 00095 */ 00096 STTS751(I2C &_i2c, 00097 bool standby = false, Address addr = ADDRESS_3, Model model = MODEL_0); 00098 00099 /** Initialize the device 00100 */ 00101 void init(); 00102 00103 /** The I2C slave address (8bit) of the device 00104 * 00105 * @returns The I2C slave address (8bit) of the device 00106 */ 00107 int addr(); 00108 00109 /** Get the current temperature resolution 00110 * 00111 * @returns The current temperature resolution 00112 */ 00113 Resolution resolution(); 00114 00115 /** Set the temperature resolution 00116 * 00117 * @param res Resolution (defaults to RES_10) 00118 */ 00119 void setResolution(Resolution res = RES_10); 00120 00121 /** Get the current conversion rate 00122 * 00123 * @returns The current conversion rate 00124 */ 00125 int conversionRate(); 00126 00127 /** Set the conversion rate 00128 * Set the number of times the temperature value is updated each second. 00129 * The conversion rate (times/second) can be calculated by 2^(rate - 4). 00130 * Note that rate should be less than 8 if the temperature resolution is 12-bit and 00131 * should be less than 9 if the temperature resolution is greater than 10-bit 00132 * 00133 * @param rate Conversion rate (0 .. 9) 00134 */ 00135 void setConversionRate(int rate); 00136 00137 /** Set the device mode 00138 * 00139 * @param standby true : standby mode, false : continuous conversion mode 00140 */ 00141 void setStandbyMode(bool standby); 00142 00143 /** Start a temperature conversion (in standby mode) 00144 */ 00145 void start(); 00146 00147 /** Checks that a temperature conversion has finished and the temperature value is available 00148 * 00149 * @returns true if a temperature conversion has finished and the temperature value is available 00150 */ 00151 bool ready(); 00152 00153 /** Obtain the current temperature measurement 00154 * 00155 * @param nowait If true, read the temperature value without waiting for finishing a conversion (in standby mode) 00156 * @returns The current temperature measurement in Celsius. 00157 */ 00158 float temp(bool nowait = false); 00159 00160 #ifdef MBED_OPERATORS 00161 /** A shorthand for temp() 00162 * 00163 * @returns The current temperature measurement in Celsius. 00164 */ 00165 operator float(); 00166 #endif 00167 00168 protected: 00169 /** I2C registers 00170 */ 00171 enum { 00172 REG_TEMPERATURE_H = 0x00, 00173 REG_STATUS = 0x01, 00174 REG_TEMPERATURE_L = 0x02, 00175 REG_CONFIGURATION = 0x03, 00176 REG_CONV_RATE = 0x04, 00177 REG_HIGH_LIMIT_H = 0x05, 00178 REG_HIGH_LIMIT_L = 0x06, 00179 REG_LOW_LIMIT_H = 0x07, 00180 REG_LOW_LIMIT_L = 0x08, 00181 REG_ONESHOT = 0x0f, 00182 REG_THERM = 0x20, 00183 REG_THERM_HYSTERESIS = 0x21, 00184 REG_SMBUS_TIMEOUT = 0x22, 00185 REG_PRODUCT_ID = 0xfd, 00186 REG_MFG_ID = 0xfe, 00187 REG_REVISION_ID = 0xff, 00188 }; 00189 00190 /** Configuration register (default 0x00) 00191 * - b7 : MASK1 00192 * - b6 : ~RUN/STOP (0 : continuous conversion mode, 1 : standby mode) 00193 * - b5 : 0 00194 * - b4 : RFU 00195 * - b3 : Tres1 00196 * - b2 : Tres0 00197 * - b1-b0 : RFU 00198 */ 00199 enum { 00200 CONF_MASK1 = 0x80, 00201 CONF_RUNSTOP = 0x40, 00202 CONF_RES_MASK = 0x0c, 00203 }; 00204 00205 /** Status Register (default undefined) 00206 * - b7 : Busy 00207 * - b6 : T_HIGH 00208 * - b5 : T_LOW 00209 * - b4-b1 : RFU 00210 * - b0 : THRM 00211 */ 00212 enum { 00213 STATUS_BUSY = 0x80, 00214 STATUS_THIGH = 0x40, 00215 STATUS_LOW = 0x20, 00216 STATUS_THURM = 0x01 00217 }; 00218 00219 /** Conversion Rate Register (default 4) 00220 * - b7-b4: 0 00221 * - b3-b0: Conversion rate (0..9) 00222 */ 00223 enum { 00224 CONV_RATE_MASK = 0x0f 00225 }; 00226 00227 I2C _i2c; 00228 const int _addr; 00229 bool _standby; 00230 00231 char read8(char reg); 00232 void write8(char reg, char data); 00233 }; 00234 00235 #endif
Generated on Fri Jul 15 2022 05:08:55 by
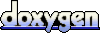