A library for STMicroelectronics STTS751 I2C temperature sensor
Embed:
(wiki syntax)
Show/hide line numbers
STTS751.cpp
00001 /* A library for STMicroelectronics STTS751 I2C temperature sensor 00002 * Copyright 2014, Takuo WATANABE (wtakuo) 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "STTS751.h" 00018 00019 #define SET_BITS(x,m,b) (((x) & ~(m)) | (b)) 00020 00021 STTS751::STTS751(PinName sda, PinName scl, bool standby, Address addr, Model model) : 00022 _i2c(sda, scl), _addr(addr | model), _standby(standby) { 00023 init(); 00024 } 00025 00026 STTS751::STTS751(I2C &i2c, bool standby, Address addr, Model model) : 00027 _i2c(i2c), _addr(addr | model), _standby(standby) { 00028 init(); 00029 } 00030 00031 void STTS751::init() { 00032 char conf = 0x00; 00033 if (_standby) 00034 conf |= CONF_RUNSTOP; 00035 write8(REG_CONFIGURATION, conf); 00036 // conversion rate = 1/sec 00037 write8(REG_CONV_RATE, 0x04); 00038 } 00039 00040 int STTS751::addr() { 00041 return _addr; 00042 } 00043 00044 STTS751::Resolution STTS751::resolution() { 00045 char conf = read8(REG_CONFIGURATION); 00046 return (STTS751::Resolution) (conf & CONF_RES_MASK); 00047 } 00048 00049 void STTS751::setResolution(STTS751::Resolution res) { 00050 char conf = read8(REG_CONFIGURATION); 00051 conf = SET_BITS(conf, CONF_RES_MASK, res); 00052 write8(REG_CONFIGURATION, conf); 00053 } 00054 00055 int STTS751::conversionRate() { 00056 char conv = read8(REG_CONV_RATE); 00057 return conv & CONV_RATE_MASK; 00058 } 00059 00060 void STTS751::setConversionRate(int rate) { 00061 write8(REG_CONV_RATE, (char)(rate & CONV_RATE_MASK)); 00062 } 00063 00064 void STTS751::setStandbyMode(bool standby) { 00065 _standby = standby; 00066 char conf = read8(REG_CONFIGURATION); 00067 if (_standby) 00068 conf |= CONF_RUNSTOP; 00069 else 00070 conf &= ~CONF_RUNSTOP; 00071 write8(REG_CONFIGURATION, conf); 00072 } 00073 00074 void STTS751::start() { 00075 if (ready()) 00076 write8(REG_ONESHOT, 1); 00077 } 00078 00079 bool STTS751::ready() { 00080 char status = read8(REG_STATUS); 00081 return (status & STATUS_BUSY) == 0; 00082 } 00083 00084 float STTS751::temp(bool nowait) { 00085 if (_standby && !nowait) { 00086 start(); 00087 while (!ready()) 00088 wait(0.01); 00089 } 00090 signed char h = read8(REG_TEMPERATURE_H); 00091 unsigned char l = read8(REG_TEMPERATURE_L); 00092 return ((h << 8) | l) / 256.0; 00093 } 00094 00095 #ifdef MBED_OPERATORS 00096 STTS751::operator float() { 00097 return temp(); 00098 } 00099 #endif 00100 00101 char STTS751::read8(char reg) { 00102 _i2c.write(_addr, ®, 1, true); 00103 _i2c.read(_addr, ®, 1); 00104 return reg; 00105 } 00106 00107 void STTS751::write8(char reg, char data) { 00108 char buff[2] = { reg, data }; 00109 _i2c.write(_addr, buff, 2); 00110 }
Generated on Fri Jul 15 2022 05:08:55 by
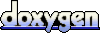