
Simple example to demonstrate the USB MIDI library
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // Hello World example for the USBMIDI library 00002 00003 #include "mbed.h" 00004 #include "USBMIDI.h" 00005 00006 void show_message(MIDIMessage msg) { 00007 switch (msg.type()) { 00008 case MIDIMessage::NoteOnType: 00009 printf("NoteOn key:%d, velocity: %d, channel: %d\n", msg.key(), msg.velocity(), msg.channel()); 00010 break; 00011 case MIDIMessage::NoteOffType: 00012 printf("NoteOff key:%d, velocity: %d, channel: %d\n", msg.key(), msg.velocity(), msg.channel()); 00013 break; 00014 case MIDIMessage::ControlChangeType: 00015 printf("ControlChange controller: %d, data: %d\n", msg.controller(), msg.value()); 00016 break; 00017 case MIDIMessage::PitchWheelType: 00018 printf("PitchWheel channel: %d, pitch: %d\n", msg.channel(), msg.pitch()); 00019 break; 00020 default: 00021 printf("Another message\n"); 00022 } 00023 } 00024 00025 USBMIDI midi; 00026 00027 int main() { 00028 midi.attach(show_message); // call back for messages received 00029 while (1) { 00030 for(int i=48; i<83; i++) { // send some messages! 00031 midi.write(MIDIMessage::NoteOn(i)); 00032 wait(0.25); 00033 midi.write(MIDIMessage::NoteOff(i)); 00034 wait(0.5); 00035 } 00036 } 00037 }
Generated on Wed Jul 13 2022 02:24:57 by
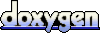