simple RGB led library
Dependents: m3Dpi MQTT-Thermostat-example Final_project_Tran Final_project_Tran ... more
Color Class Reference
#include <Color.h>
Public Types | |
enum | Colors |
Enum with named colors for easy use. More... | |
Public Member Functions | |
Color (int red, int green, int blue) | |
Create Color instance, giving individual red, green and blue factors as integer values. | |
Color (float red, float green, float blue) | |
Create Color instance, giving individual red, green and blue factors as floating point values. | |
Color (int hexColor) | |
Create Color instance, giving red, green and blue factors as a single integer value. | |
int | getHex () |
Get the color value as an integer in hexadecimal notation. | |
int | getRed () |
Get the red factor of the color. | |
int | getGreen () |
Get the green factor of the color. | |
int | getBlue () |
Get the blue factor of the color. |
Detailed Description
Color class.
Used for easy management of colors. Colors can be set by giving individual values for the red, green and blue component, both in integer and floating point notation, or as a single integer formatted in hexadecimal format (hex triplet), used in web colors.
Definition at line 11 of file Color.h.
Member Enumeration Documentation
Constructor & Destructor Documentation
Color | ( | int | red, |
int | green, | ||
int | blue | ||
) |
Create Color instance, giving individual red, green and blue factors as integer values.
- Parameters:
-
red Amount of red to be in the color. This value can be an integer between 0 (0% red) and 255 (100% red) green Amount of green to be in the color. This value can be an integer between 0 (0% green) and 255 (100% green) blue Amount of blue to be in the color. This value can be an integer between 0 (0% blue) and 255 (100% blue)
Color | ( | float | red, |
float | green, | ||
float | blue | ||
) |
Create Color instance, giving individual red, green and blue factors as floating point values.
- Parameters:
-
red Amount of red to be in the color. This value can be a float between 0.0 (0% red) and 1.0 (100% red) green Amount of green to be in the color. This value can be a float between 0.0 (0% green) and 1.0 (100% green) blue Amount of blue to be in the color. This value can a float between 0.0 (0% blue) and 1.0 (100% blue)
Color | ( | int | hexColor ) |
Create Color instance, giving red, green and blue factors as a single integer value.
- Parameters:
-
hexColor Color in hexadecimal notation (hex triplet). 24-bit RGB color as used in web colors.
- Note:
- Each color is made up of 8 bits, 0xRRGGBB
Examples:
0xFF0000 = Red 0xFFFFE0 = Light yellow 0xFA8072 = Salmon
Member Function Documentation
int getBlue | ( | ) |
int getGreen | ( | ) |
int getHex | ( | ) |
Generated on Tue Jul 12 2022 13:58:34 by
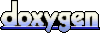