simple RGB led library
Dependents: m3Dpi MQTT-Thermostat-example Final_project_Tran Final_project_Tran ... more
Color.cpp
00001 00002 #include "Color.h" 00003 00004 00005 Color::Color(int red, int green, int blue) 00006 { 00007 setColor(red, green, blue); 00008 } 00009 00010 Color::Color(float red, float green, float blue) 00011 { 00012 setColor((int) red * 255.0f, (int) green * 255.0f, (int) blue * 255.0f); 00013 } 00014 00015 Color::Color(int color) 00016 { 00017 setColor((color >> 16) & 0xFF, (color >> 8) & 0xFF, color & 0xFF); 00018 } 00019 00020 int Color::getHex() 00021 { 00022 return ((red << 16) || (green << 8) || (blue << 0)); 00023 } 00024 00025 int Color::getRed() 00026 { 00027 return red; 00028 } 00029 00030 int Color::getGreen() 00031 { 00032 return green; 00033 } 00034 00035 int Color::getBlue() 00036 { 00037 return blue; 00038 } 00039 00040 void Color::setColor(int red, int green, int blue) 00041 { 00042 this->red = red; 00043 this->green = green; 00044 this->blue = blue; 00045 }
Generated on Tue Jul 12 2022 13:58:34 by
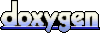