simple RGB led library
Dependents: m3Dpi MQTT-Thermostat-example Final_project_Tran Final_project_Tran ... more
Color.h
00001 00002 #ifndef COLOR_H 00003 #define COLOR_H 00004 00005 00006 /** Color class. 00007 * Used for easy management of colors. Colors can be set by giving individual values for the 00008 * red, green and blue component, both in integer and floating point notation, or as a single 00009 * integer formatted in hexadecimal format (hex triplet), used in web colors. 00010 */ 00011 class Color{ 00012 00013 int red; 00014 int green; 00015 int blue; 00016 00017 void setColor(int red, int green, int blue); 00018 00019 public: 00020 00021 /// Enum with named colors for easy use 00022 enum Colors {RED = 0xFF0000, GREEN = 0x00FF00, BLUE = 0x0000FF, CYAN = 0x00FFFF, MAGENTA = 0xFF00FF, YELLOW = 0xFFFF00, WHITE = 0xFFFFFF, BLACK = 0x000000}; 00023 00024 /** Create Color instance, giving individual red, green and blue factors as integer values 00025 * @param red Amount of red to be in the color. This value can be an integer between 0 (0% red) and 255 (100% red) 00026 * @param green Amount of green to be in the color. This value can be an integer between 0 (0% green) and 255 (100% green) 00027 * @param blue Amount of blue to be in the color. This value can be an integer between 0 (0% blue) and 255 (100% blue) 00028 */ 00029 Color(int red, int green, int blue); 00030 00031 /** Create Color instance, giving individual red, green and blue factors as floating point values 00032 * @param red Amount of red to be in the color. This value can be a float between 0.0 (0% red) and 1.0 (100% red) 00033 * @param green Amount of green to be in the color. This value can be a float between 0.0 (0% green) and 1.0 (100% green) 00034 * @param blue Amount of blue to be in the color. This value can a float between 0.0 (0% blue) and 1.0 (100% blue) 00035 */ 00036 Color(float red, float green, float blue); 00037 00038 /** Create Color instance, giving red, green and blue factors as a single integer value 00039 * @param hexColor Color in hexadecimal notation (hex triplet). 24-bit RGB color as used in web colors. 00040 * @note Each color is made up of 8 bits, 0xRRGGBB 00041 * 00042 * Examples: 00043 * @code 00044 * 0xFF0000 = Red 00045 * 0xFFFFE0 = Light yellow 00046 * 0xFA8072 = Salmon 00047 * @endcode 00048 */ 00049 Color(int hexColor); 00050 00051 /** Get the color value as an integer in hexadecimal notation 00052 * @return color as integer in hexadecimal notation (between 0x000000 and 0xFFFFFF) 00053 */ 00054 int getHex(); 00055 00056 /** Get the red factor of the color 00057 * @return red factor as integer between 0 (0% red) and 255 (100% red) 00058 */ 00059 int getRed(); 00060 00061 /** Get the green factor of the color 00062 * @return green factor as integer between 0 (0% green) and 255 (100% green) 00063 */ 00064 int getGreen(); 00065 00066 /** Get the blue factor of the color 00067 * @return blue factor as integer between 0 (0% blue) and 255 (100% blue) 00068 */ 00069 int getBlue(); 00070 }; 00071 00072 #endif
Generated on Tue Jul 12 2022 13:58:34 by
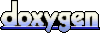