Simple library for scheduling events by polling time, that is, avoiding interrupts.
Dependents: AVC_20110423 AVC_2012
Schedule Class Reference
Simple library for scheduling events by polling time, that is, avoiding interrupts. More...
#include <Schedule.h>
Public Types | |
enum | |
Sets the behavior of getNext() after the initial schedule period has exceeded. More... | |
Public Member Functions | |
Schedule () | |
Creates an empty schedule. | |
Schedule (unsigned int scale, tick max, value start, value stop, flag m) | |
Creates a schedule based on a linear function. | |
void | scale (unsigned int scale) |
Sets a ratio of time to ticks. | |
void | max (tick max) |
Sets the total number of ticks to run the loop. | |
void | mode (flag m) |
Sets behavior of getNext() when called after tickCount exceeded. | |
void | set (tick t, value v) |
sets the value at the specified tick | |
void | set (unsigned int scale, tick max, value start, value stop, flag m) |
Set schedule based on a linear function. | |
value | get () |
get the next value for schedule's current time. | |
value | next () |
increment the clock and get the next value in the schedule | |
bool | ticked (unsigned int time) |
Pass in some unit of time and determine if the 'clock' has ticked. | |
bool | done () |
Are we done with the schedule? |
Detailed Description
Simple library for scheduling events by polling time, that is, avoiding interrupts.
Can schedule up to 64 events. The schedule is defined by the number of ticks making up the initial period of the schedule, and either a start and end value or values at specific tick values.
Definition at line 10 of file Schedule.h.
Member Enumeration Documentation
anonymous enum |
Sets the behavior of getNext() after the initial schedule period has exceeded.
- Parameters:
-
repeat means the schedule values are repeated hold means the last schedule value is provided after the schdule is done
Definition at line 17 of file Schedule.h.
Constructor & Destructor Documentation
Schedule | ( | ) |
Creates an empty schedule.
Definition at line 3 of file Schedule.cpp.
Schedule | ( | unsigned int | scale, |
tick | max, | ||
value | start, | ||
value | stop, | ||
flag | m | ||
) |
Creates a schedule based on a linear function.
See set()
- Parameters:
-
scale max the maximum tick value of the clock, sets the period start is the value returned at tick == 0 stop is the value returned at tick == ticks-1 m selects the mode / behavior of the schedule when getNext() called after period exceeded
Definition at line 10 of file Schedule.cpp.
Member Function Documentation
bool done | ( | ) |
Are we done with the schedule?
- Returns:
- true if schedule is done; see max()
Definition at line 91 of file Schedule.cpp.
value get | ( | ) |
get the next value for schedule's current time.
Use with ticked()
- Returns:
- the value at the current schedule's time
Definition at line 57 of file Schedule.cpp.
void max | ( | tick | max ) |
Sets the total number of ticks to run the loop.
Definition at line 21 of file Schedule.cpp.
void mode | ( | flag | m ) |
Sets behavior of getNext() when called after tickCount exceeded.
Definition at line 28 of file Schedule.cpp.
value next | ( | ) |
increment the clock and get the next value in the schedule
- Returns:
- the value at the schedule's next clock tick
Definition at line 72 of file Schedule.cpp.
void scale | ( | unsigned int | scale ) |
Sets a ratio of time to ticks.
See clockTicked()
- Parameters:
-
timePerTick specifies the number of time units per tick
Definition at line 15 of file Schedule.cpp.
void set | ( | tick | t, |
value | v | ||
) |
sets the value at the specified tick
- Parameters:
-
t specifies the scheduled tick v specifies the value to return when tick==whichTick
Definition at line 48 of file Schedule.cpp.
void set | ( | unsigned int | scale, |
tick | max, | ||
value | start, | ||
value | stop, | ||
flag | m | ||
) |
Set schedule based on a linear function.
- Parameters:
-
ticks total number of ticks over which the schedule is valid startValue is the value returned at tick == 0 stopValue is the value returned at tick == ticks-1
Definition at line 34 of file Schedule.cpp.
bool ticked | ( | unsigned int | time ) |
Pass in some unit of time and determine if the 'clock' has ticked.
Suppose timePerTick == 20 and you pass in the elapsed time in milliseconds then this function returns true every 20ms.
- Parameters:
-
time the integer corresponding to elapsed time
- Returns:
- true if the elapsed time % timePerTick == 0
Definition at line 79 of file Schedule.cpp.
Generated on Wed Jul 13 2022 10:41:54 by
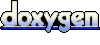