
Code for autonomous ground vehicle, Data Bus, 3rd place winner in 2012 Sparkfun AVC.
Dependencies: Watchdog mbed Schedule SimpleFilter LSM303DLM PinDetect DebounceIn Servo
SerialGraphicLCD.h
00001 /* Serial Graphics LCD Driver for Sparkfun Serial Graphics LCD, LCD-09351; and Graphic 00002 * LCD Serial Backpack, LCD-09352. 00003 * 00004 * @author Michael Shimniok http://www.bot-thoughts.com/ 00005 * 00006 */ 00007 #ifndef _SERIALGRAPHICLCD_H 00008 #define _SERIALGRAPHICLCD_H 00009 00010 #include "mbed.h" 00011 00012 /** Firmware modes */ 00013 #define SFE_FW 0 // Stock SFE firmware 00014 #define SD_FW 1 // summoningdark firmware http://sourceforge.net/projects/serialglcd/ 00015 00016 /** Modes for SD firmware */ 00017 #define FILL 0xFF 00018 #define CLEAR 0x00 00019 00020 /** LCD Baud Rates */ 00021 #define LCD_4800 1 00022 #define LCD_9600 2 00023 #define LCD_19200 3 00024 #define LCD_38400 4 00025 #define LCD_57600 5 00026 #define LCD_115200 6 00027 00028 /** LCD Types */ 00029 #define LCD_128x64 1 00030 #define LCD_160x128 2 00031 00032 /** Interface to the Sparkfun Serial Graphic LCD, LCD-09351; and Graphic 00033 * LCD Serial Backpack, LCD-09352. Derived class from Serial so that you 00034 * can conveniently printf(), putc(), etc to the display. 00035 * 00036 * Example: 00037 * @code 00038 * #include "mbed.h" 00039 * #include "SerialGraphicLCD.h" 00040 * 00041 * SerialGraphicLCD lcd(p26, p25); 00042 * 00043 * int main() { 00044 * lcd.baud(115200); // default baud rate 00045 * while (1) { 00046 * lcd.clear(); 00047 * lcd.rect(3, 3, 20, 20); 00048 * lcd.printf("Hello World!"); 00049 * lcd.pixel(14, 35, true); 00050 * lcd.pixel(16, 36, true); 00051 * lcd.pixel(18, 37, true); 00052 * lcd.pos(5, 30); 00053 * lcd.printf("Hi"); 00054 * lcd.circle(50, 20, 20, true); 00055 * lcd.pos(50, 20); 00056 * lcd.printf("Howdy"); 00057 * lcd.line(0, 0, 25, 25, true); 00058 * wait(2); 00059 * } 00060 * } 00061 * @endcode 00062 */ 00063 class SerialGraphicLCD: public Serial { 00064 public: 00065 /** Create a new interface to a Serial Graphic LCD 00066 * Note that the display lower left corner is coordinates 0, 0. 00067 * Rows start at the top at 0, columns start at the left at 0. 00068 * @param tx -- mbed transmit pin 00069 * @param rx -- mbed receive pin 00070 */ 00071 SerialGraphicLCD(PinName tx, PinName rx); 00072 00073 /** Create a new interface to a Serial Graphic LCD 00074 * Note that the display lower left corner is coordinates 0, 0. 00075 * Rows start at the top at 0, columns start at the left at 0. 00076 * @param tx -- mbed transmit pin 00077 * @param rx -- mbed receive pin 00078 * @param firmware -- SFE_FW, stock firmware or SD_FW, summoningdark firmware 00079 */ 00080 SerialGraphicLCD(PinName tx, PinName rx, int firmware); 00081 00082 /** clear the screen 00083 */ 00084 void clear(void); 00085 00086 /** set text position in rows, columns 00087 * 00088 * @param col is the col coordinate 00089 * @param row is the row coordinate 00090 */ 00091 void pos(int col, int row); 00092 00093 /** set text position in x, y coordinates 00094 * 00095 * @param x is the x coordinate 00096 * @param y is the y coordinate 00097 */ 00098 void posXY(int x, int y); 00099 00100 /** set or erase a pixel 00101 * 00102 * @param x is the x coordinate 00103 * @param y is the y coordinate 00104 * @param set if true sets the pixel, if false, erases it 00105 */ 00106 void pixel(int x, int y, bool set); 00107 00108 /** draw or erase a line 00109 * 00110 * @param x1 is the x coordinate of the start of the line 00111 * @param y1 is the y coordinate of the start of the line 00112 * @param x2 is the x coordinate of the end of the line 00113 * @param y2 is the y coordinate of the end of the line 00114 * @param set if true sets the line, if false, erases it 00115 */ 00116 void line(int x1, int y1, int x2, int y2, bool set); 00117 00118 /** set or reset a circle 00119 * 00120 * @param x is the x coordinate of the circle center 00121 * @param y is the y coordinate of the circle center 00122 * @param r is the radius of the circle 00123 * @param set if true sets the pixel, if false, clears it 00124 */ 00125 void circle(int x, int y, int r, bool set); 00126 00127 /** draw a rectangle 00128 * 00129 * @param x1 is the x coordinate of the upper left of the rectangle 00130 * @param y1 is the y coordinate of the upper left of the rectangle 00131 * @param x2 is the x coordinate of the lower right of the rectangle 00132 * @param y2 is the y coordinate of the lower right of the rectangle 00133 */ 00134 void rect(int x1, int y1, int x2, int y2); 00135 00136 /** draw or erase a rectangle (SD firmware only) 00137 * 00138 * @param x1 is the x coordinate of the upper left of the rectangle 00139 * @param y1 is the y coordinate of the upper left of the rectangle 00140 * @param x2 is the x coordinate of the lower right of the rectangle 00141 * @param y2 is the y coordinate of the lower right of the rectangle 00142 */ 00143 void rect(int x1, int y1, int x2, int y2, bool set); 00144 00145 /** Draw a filled box. 00146 * 00147 * @param x1 is the x coordinate of the upper left of the rectangle 00148 * @param y1 is the y coordinate of the upper left of the rectangle 00149 * @param x2 is the x coordinate of the lower right of the rectangle 00150 * @param y2 is the y coordinate of the lower right of the rectangle 00151 * @param fillByte describes 1 8-pixel high stripe that is repeated every x 00152 * pixels and every 8 y pixels. The most useful are CLEAR (0x00) to clear the box, and FILL (0xFF) to fill it. 00153 */ 00154 void rectFill(int x1, int y1, int x2, int y2, char fillByte); 00155 00156 /** erase a rectangular area 00157 * 00158 * @param x1 is the x coordinate of the upper left of the area 00159 * @param y1 is the y coordinate of the upper left of the area 00160 * @param x2 is the x coordinate of the lower right of the area 00161 * @param y2 is the y coordinate of the lower right of the area 00162 */ 00163 void erase(int x1, int y1, int x2, int y2); 00164 00165 /** set backlight duty cycle 00166 * 00167 * @param i is the duty cycle from 0 to 100; 0 is off, 100 is full power 00168 */ 00169 void backlight(int i); 00170 00171 /** clear screen and put in reverse mode 00172 */ 00173 void reverseMode(void); 00174 00175 /** configure the lcd baud rate so you have to call this along with baud() to change 00176 * communication speeds 00177 * 00178 * @param b is the baud rate, LCD_4800, LCD_9600, LCD_19200, LCD_38400, LCD_57600 or LCD_115200 00179 */ 00180 void lcdbaud(int b); 00181 00182 00183 /** sets the resolution of the LCD so that the pos() call works properly 00184 * defaults to LCD_128x64. 00185 * 00186 * @param type is the type of LCD, either LCD_128x64 or LCD_160x128 00187 */ 00188 void resolution(int type); 00189 00190 /** sets the resolution of the LCD in x and y coordinates which determines 00191 * how rows and columns are calculated in the pos() call. Defaults to 00192 * x=128, y=64 00193 * 00194 * @param x is the number of horizontal pixels 00195 * @param y is the number of vertical pixels 00196 */ 00197 void resolution(int x, int y); 00198 00199 private: 00200 int _xMax; 00201 int _yMax; 00202 int _firmware; 00203 }; 00204 00205 00206 #endif
Generated on Tue Jul 12 2022 14:09:28 by
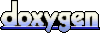