
Code for autonomous ground vehicle, Data Bus, 3rd place winner in 2012 Sparkfun AVC.
Dependencies: Watchdog mbed Schedule SimpleFilter LSM303DLM PinDetect DebounceIn Servo
SerialGraphicLCD.cpp
00001 #include "SerialGraphicLCD.h" 00002 00003 #define XSIZE 6 00004 #define YSIZE 9 00005 00006 SerialGraphicLCD::SerialGraphicLCD(PinName tx, PinName rx): 00007 Serial(tx, rx), _firmware(SFE_FW) 00008 { 00009 baud(115200); // default baud rate 00010 resolution(LCD_128x64); // default resolution 00011 } 00012 00013 SerialGraphicLCD::SerialGraphicLCD(PinName tx, PinName rx, int firmware): 00014 Serial(tx, rx), _firmware(firmware) 00015 { 00016 baud(115200); // default baud rate 00017 resolution(LCD_128x64); // default resolution 00018 } 00019 00020 void SerialGraphicLCD::clear() { 00021 putc(0x7c); 00022 putc(0x00); 00023 } 00024 00025 void SerialGraphicLCD::pos(int col, int row) { 00026 if (_firmware == SD_FW) 00027 posXY(XSIZE*col, (YSIZE*row)); 00028 else if (_firmware == SFE_FW) 00029 posXY(XSIZE*col, _yMax-(YSIZE*row)-1); 00030 } 00031 00032 void SerialGraphicLCD::posXY(int x, int y) { 00033 putc(0x7c); 00034 putc(0x18); 00035 putc(x); 00036 putc(0x7c); 00037 putc(0x19); 00038 putc(y); 00039 } 00040 00041 void SerialGraphicLCD::pixel(int x, int y, bool set) { 00042 putc(0x7c); 00043 putc(0x10); 00044 putc(x); 00045 putc(y); 00046 putc((set) ? 0x01 : 0x00); 00047 } 00048 00049 void SerialGraphicLCD::line(int x1, int y1, int x2, int y2, bool set) { 00050 putc(0x7c); 00051 putc(0x0c); 00052 putc(x1); 00053 putc(y1); 00054 putc(x2); 00055 putc(y2); 00056 putc((set) ? 0x01 : 0x00); 00057 } 00058 00059 void SerialGraphicLCD::circle(int x, int y, int r, bool set) { 00060 putc(0x7c); 00061 putc(0x03); 00062 putc(x); 00063 putc(y); 00064 putc(r); 00065 putc((set) ? 0x01 : 0x00); 00066 } 00067 00068 // Unfortunately, the datasheet for the stock firmware is incorrect; 00069 // the box command does not take a 5th parameter for draw/erase like the others 00070 // However, it does in the sd firmware 00071 void SerialGraphicLCD::rect(int x1, int y1, int x2, int y2) { 00072 putc(0x7c); 00073 putc(0x0f); 00074 putc(x1); 00075 putc(y1); 00076 putc(x2); 00077 putc(y2); 00078 if (_firmware == SD_FW) 00079 putc(0x01); 00080 } 00081 00082 void SerialGraphicLCD::rect(int x1, int y1, int x2, int y2, bool set) { 00083 putc(0x7c); 00084 putc(0x0f); 00085 putc(x1); 00086 putc(y1); 00087 putc(x2); 00088 putc(y2); 00089 if (_firmware == SD_FW) 00090 putc((set) ? 0x01 : 0x00); 00091 } 00092 00093 void SerialGraphicLCD::rectFill(int x1, int y1, int x2, int y2, char fillByte) { 00094 if (_firmware == SD_FW) { 00095 00096 // Bugs in firmware; if y2-y1 == 2, nothing drawn; if y2-y1 == 3, fill is 4 tall 00097 // if ((y2 - y1) > 3) { 00098 putc(0x7c); 00099 putc(0x12); 00100 putc(x1); 00101 putc(y1); 00102 putc(x2+1); // bug in firmware, off-by-one on x2 00103 putc(y2); 00104 putc(fillByte); 00105 // } else { 00106 // for (int y=y1; y <= y2; y++) 00107 // line(x1, y, x2, y, fillByte == FILL); 00108 // } 00109 } 00110 } 00111 00112 void SerialGraphicLCD::erase(int x1, int y1, int x2, int y2) { 00113 putc(0x7c); 00114 putc(0x05); 00115 putc(x1); 00116 putc(y1); 00117 putc(x2); 00118 putc(y2); 00119 } 00120 00121 void SerialGraphicLCD::backlight(int i) { 00122 if (i >= 0 && i <= 100) { 00123 putc(0x7c); 00124 putc(0x02); 00125 putc(i); 00126 } 00127 } 00128 00129 void SerialGraphicLCD::reverseMode() { 00130 putc(0x7c); 00131 putc(0x12); 00132 } 00133 00134 void SerialGraphicLCD::resolution(int type) { 00135 switch (type) { 00136 case LCD_128x64 : 00137 resolution(128, 64); 00138 break; 00139 case LCD_160x128 : 00140 resolution(160, 128); 00141 break; 00142 } 00143 } 00144 00145 void SerialGraphicLCD::resolution(int x, int y) { 00146 _xMax = x; 00147 _yMax = y; 00148 } 00149 00150 00151 void SerialGraphicLCD::lcdbaud(int b) { 00152 if (b > 0 && b < 7) { 00153 putc(0x7c); 00154 putc(0x07); 00155 putc(b+'0'); 00156 } 00157 }
Generated on Tue Jul 12 2022 14:09:28 by
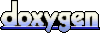