
Code for autonomous ground vehicle, Data Bus, 3rd place winner in 2012 Sparkfun AVC.
Dependencies: Watchdog mbed Schedule SimpleFilter LSM303DLM PinDetect DebounceIn Servo
CartPosition.h
00001 #ifndef __CARTPOSITION_H 00002 #define __CARTPOSITION_H 00003 00004 /** Geographical position and calculation based on cartesian coordinates 00005 */ 00006 class CartPosition { 00007 public: 00008 /** Create a new cartesian coordinate object 00009 */ 00010 CartPosition(void); 00011 /** Create a new cartesian coordinate object 00012 * @param x sets x coordinate 00013 * @param y sets y coordinate 00014 */ 00015 CartPosition(float x, float y); 00016 /** Sets coordinates for object 00017 * @param x sets x coordinate 00018 * @param y sets y coordinate 00019 */ 00020 void set(float x, float y); 00021 /** Sets coordinates for object 00022 * @param p sets coordinates of this object to that of p 00023 */ 00024 void set(CartPosition p); 00025 /** Computes bearing to a position from this position 00026 * @param to is the coordinate to which we're calculating bearing 00027 */ 00028 float bearingTo(CartPosition to); 00029 /** Computes distance to a position from this position 00030 * @param to is the coordinate to which we're calculating distance 00031 */ 00032 float distanceTo(CartPosition to); 00033 /** Computes the new coordinates for this object given a bearing and distance 00034 * @param bearing is the direction traveled 00035 * @distance is the distance traveled 00036 */ 00037 void move(float bearing, float distance); 00038 /** x coordinate of this object */ 00039 float _x; 00040 /** y coordinate of this object */ 00041 float _y; 00042 }; 00043 #endif
Generated on Tue Jul 12 2022 14:09:25 by
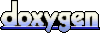