
Code for autonomous ground vehicle, Data Bus, 3rd place winner in 2012 Sparkfun AVC.
Dependencies: Watchdog mbed Schedule SimpleFilter LSM303DLM PinDetect DebounceIn Servo
Bargraph.cpp
00001 #include "Bargraph.h" 00002 00003 #define WIDTH 8 00004 #define HEIGHT 9 00005 00006 SerialGraphicLCD *Bargraph::lcd = 0; 00007 00008 Bargraph::Bargraph(int x, int y, int size, char name): 00009 _x(x), _y(y), _x2(x+WIDTH), _y2(y+size-1), _s(size), _n(name), _last(0) 00010 { 00011 } 00012 00013 Bargraph::Bargraph(int x, int y, int size, int width, char name): 00014 _x(x), _y(y), _x2(x+width-1), _y2(y+size-1), _s(size), _w(width), _n(name), _last(0) 00015 { 00016 } 00017 00018 void Bargraph::init() 00019 { 00020 if (lcd) { 00021 if (_n != ' ') { 00022 lcd->posXY(_x, _y2+2); // horizontal center 00023 lcd->printf("%c", _n); 00024 } 00025 lcd->rect(_x, _y, _x2, _y2, true); 00026 int value = _last; 00027 _last = 0; 00028 update(value); 00029 } 00030 } 00031 00032 void Bargraph::calibrate(float min, float max) 00033 { 00034 _min = min; 00035 _max = max; 00036 } 00037 00038 void Bargraph::update(float value) 00039 { 00040 int ivalue; 00041 00042 ivalue = (int) ((value - _min) * (_s-1)/(_max - _min)); 00043 00044 update(ivalue); 00045 00046 return; 00047 } 00048 00049 void Bargraph::update(int value) 00050 { 00051 if (lcd) { 00052 if (value >= 0 && value < _s) { 00053 int newY = _y2-value; 00054 00055 for (int y=_y+1; y < _y2; y++) { 00056 lcd->line(_x+1, y, _x2-1, y, (y > newY)); 00057 wait_ms(5); 00058 } 00059 } 00060 _last = value; 00061 } 00062 } 00063
Generated on Tue Jul 12 2022 14:09:25 by
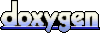