Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
Ticker Class Reference
A Ticker is used to call a function at a recurring interval. More...
#include <Ticker.h>
Inherits mbed::TimerEvent.
Inherited by Timeout.
Public Member Functions | |
void | attach (void(*fptr)(void), float t) |
Attach a function to be called by the Ticker, specifiying the interval in seconds. | |
template<typename T > | |
void | attach (T *tptr, void(T::*mptr)(void), float t) |
Attach a member function to be called by the Ticker, specifiying the interval in seconds. | |
void | attach_us (void(*fptr)(void), unsigned int t) |
Attach a function to be called by the Ticker, specifiying the interval in micro-seconds. | |
template<typename T > | |
void | attach_us (T *tptr, void(T::*mptr)(void), unsigned int t) |
Attach a member function to be called by the Ticker, specifiying the interval in micro-seconds. | |
void | detach () |
Detach the function. | |
Static Public Member Functions | |
static void | irq () |
The handler registered with the underlying timer interrupt. | |
Protected Member Functions | |
virtual void | handler () |
The handler called to service the timer event of the derived class. | |
void | insert (unsigned int timestamp) |
Insert in to linked list. | |
void | remove () |
Remove from linked list, if in it. | |
Static Protected Member Functions | |
static unsigned int | timestamp () |
Get the current usec timestamp. | |
Protected Attributes | |
TimerEvent * | _next |
Pointer to the next in the list, NULL if last. | |
unsigned int | _timestamp |
The timestamp at which the even should be triggered. | |
Static Protected Attributes | |
static TimerEvent * | _head |
The head of the list of the events, NULL if none. |
Detailed Description
A Ticker is used to call a function at a recurring interval.
You can use as many seperate Ticker objects as you require.
Example:
// Toggle the blinking led after 5 seconds #include "mbed.h" Ticker timer; DigitalOut led1(LED1); DigitalOut led2(LED2); int flip = 0; void attime() { flip = !flip; } int main() { timer.attach(&attime, 5); while(1) { if(flip == 0) { led1 = !led1; } else { led2 = !led2; } wait(0.2); } }
Definition at line 46 of file Ticker.h.
Member Function Documentation
void attach | ( | void(*)(void) | fptr, |
float | t | ||
) |
void attach | ( | T * | tptr, |
void(T::*)(void) | mptr, | ||
float | t | ||
) |
void attach_us | ( | T * | tptr, |
void(T::*)(void) | mptr, | ||
unsigned int | t | ||
) |
void attach_us | ( | void(*)(void) | fptr, |
unsigned int | t | ||
) |
void detach | ( | ) |
Detach the function.
virtual void handler | ( | ) | [protected, virtual] |
The handler called to service the timer event of the derived class.
Implements TimerEvent.
Reimplemented in Timeout.
void insert | ( | unsigned int | timestamp ) | [protected, inherited] |
Insert in to linked list.
static void irq | ( | ) | [static, inherited] |
The handler registered with the underlying timer interrupt.
void remove | ( | ) | [protected, inherited] |
Remove from linked list, if in it.
static unsigned int timestamp | ( | ) | [static, protected, inherited] |
Get the current usec timestamp.
Field Documentation
TimerEvent* _head [static, protected, inherited] |
The head of the list of the events, NULL if none.
Definition at line 46 of file TimerEvent.h.
TimerEvent* _next [protected, inherited] |
Pointer to the next in the list, NULL if last.
Definition at line 50 of file TimerEvent.h.
unsigned int _timestamp [protected, inherited] |
The timestamp at which the even should be triggered.
Definition at line 54 of file TimerEvent.h.
Generated on Tue Jul 12 2022 11:27:31 by
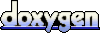