Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
TimerEvent.h
00001 /* mbed Microcontroller Library - TimerEvent 00002 * Copyright (c) 2007-2009 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_TIMEREVENT_H 00006 #define MBED_TIMEREVENT_H 00007 00008 namespace mbed { 00009 00010 /** Base abstraction for timer interrupts 00011 */ 00012 class TimerEvent { 00013 00014 public: 00015 00016 TimerEvent(); 00017 00018 /** The handler registered with the underlying timer interrupt 00019 */ 00020 static void irq(); 00021 00022 /** Destruction removes it... 00023 */ 00024 virtual ~TimerEvent(); 00025 00026 protected: 00027 00028 /** The handler called to service the timer event of the derived class 00029 */ 00030 virtual void handler() = 0; 00031 00032 /** Insert in to linked list 00033 */ 00034 void insert(unsigned int timestamp); 00035 00036 /** Remove from linked list, if in it 00037 */ 00038 void remove(); 00039 00040 /** Get the current usec timestamp 00041 */ 00042 static unsigned int timestamp(); 00043 00044 /** The head of the list of the events, NULL if none 00045 */ 00046 static TimerEvent *_head; 00047 00048 /** Pointer to the next in the list, NULL if last 00049 */ 00050 TimerEvent *_next; 00051 00052 /** The timestamp at which the even should be triggered 00053 */ 00054 unsigned int _timestamp; 00055 00056 }; 00057 00058 } // namespace mbed 00059 00060 #endif
Generated on Tue Jul 12 2022 11:27:27 by
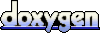