Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
Ticker.h
00001 /* mbed Microcontroller Library - Ticker 00002 * Copyright (c) 2007-2009 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_TICKER_H 00006 #define MBED_TICKER_H 00007 00008 #include "TimerEvent.h" 00009 #include "FunctionPointer.h" 00010 00011 namespace mbed { 00012 00013 /** A Ticker is used to call a function at a recurring interval 00014 * 00015 * You can use as many seperate Ticker objects as you require. 00016 * 00017 * Example: 00018 * @code 00019 * // Toggle the blinking led after 5 seconds 00020 * 00021 * #include "mbed.h" 00022 * 00023 * Ticker timer; 00024 * DigitalOut led1(LED1); 00025 * DigitalOut led2(LED2); 00026 * 00027 * int flip = 0; 00028 * 00029 * void attime() { 00030 * flip = !flip; 00031 * } 00032 * 00033 * int main() { 00034 * timer.attach(&attime, 5); 00035 * while(1) { 00036 * if(flip == 0) { 00037 * led1 = !led1; 00038 * } else { 00039 * led2 = !led2; 00040 * } 00041 * wait(0.2); 00042 * } 00043 * } 00044 * @endcode 00045 */ 00046 class Ticker : public TimerEvent { 00047 00048 public: 00049 00050 /** Attach a function to be called by the Ticker, specifiying the interval in seconds 00051 * 00052 * @param fptr pointer to the function to be called 00053 * @param t the time between calls in seconds 00054 */ 00055 void attach(void (*fptr)(void), float t) { 00056 attach_us(fptr, t * 1000000.0f); 00057 } 00058 00059 /** Attach a member function to be called by the Ticker, specifiying the interval in seconds 00060 * 00061 * @param tptr pointer to the object to call the member function on 00062 * @param mptr pointer to the member function to be called 00063 * @param t the time between calls in seconds 00064 */ 00065 template<typename T> 00066 void attach(T* tptr, void (T::*mptr)(void), float t) { 00067 attach_us(tptr, mptr, t * 1000000.0f); 00068 } 00069 00070 /** Attach a function to be called by the Ticker, specifiying the interval in micro-seconds 00071 * 00072 * @param fptr pointer to the function to be called 00073 * @param t the time between calls in micro-seconds 00074 */ 00075 void attach_us(void (*fptr)(void), unsigned int t) { 00076 _function.attach(fptr); 00077 setup(t); 00078 } 00079 00080 /** Attach a member function to be called by the Ticker, specifiying the interval in micro-seconds 00081 * 00082 * @param tptr pointer to the object to call the member function on 00083 * @param mptr pointer to the member function to be called 00084 * @param t the time between calls in micro-seconds 00085 */ 00086 template<typename T> 00087 void attach_us(T* tptr, void (T::*mptr)(void), unsigned int t) { 00088 _function.attach(tptr, mptr); 00089 setup(t); 00090 } 00091 00092 /** Detach the function 00093 */ 00094 void detach(); 00095 00096 protected: 00097 00098 void setup(unsigned int t); 00099 virtual void handler(); 00100 00101 unsigned int _delay; 00102 FunctionPointer _function; 00103 00104 }; 00105 00106 } // namespace mbed 00107 00108 #endif
Generated on Tue Jul 12 2022 11:27:27 by
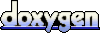