Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
Serial.h
00001 /* mbed Microcontroller Library - Serial 00002 * Copyright (c) 2007-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_SERIAL_H 00006 #define MBED_SERIAL_H 00007 00008 #include "device.h" 00009 00010 #if DEVICE_SERIAL 00011 00012 #include "platform.h" 00013 #include "PinNames.h" 00014 #include "PeripheralNames.h" 00015 #include "Stream.h" 00016 #include "FunctionPointer.h" 00017 00018 namespace mbed { 00019 00020 /** A serial port (UART) for communication with other serial devices 00021 * 00022 * Can be used for Full Duplex communication, or Simplex by specifying 00023 * one pin as NC (Not Connected) 00024 * 00025 * Example: 00026 * @code 00027 * // Print "Hello World" to the PC 00028 * 00029 * #include "mbed.h" 00030 * 00031 * Serial pc(USBTX, USBRX); 00032 * 00033 * int main() { 00034 * pc.printf("Hello World\n"); 00035 * } 00036 * @endcode 00037 */ 00038 class Serial : public Stream { 00039 00040 public: 00041 00042 /** Create a Serial port, connected to the specified transmit and receive pins 00043 * 00044 * @param tx Transmit pin 00045 * @param rx Receive pin 00046 * 00047 * @note 00048 * Either tx or rx may be specified as NC if unused 00049 */ 00050 Serial(PinName tx, PinName rx, const char *name = NULL); 00051 00052 /** Set the baud rate of the serial port 00053 * 00054 * @param baudrate The baudrate of the serial port (default = 9600). 00055 */ 00056 void baud(int baudrate); 00057 00058 enum Parity { 00059 None = 0 00060 , Odd 00061 , Even 00062 , Forced1 00063 , Forced0 00064 }; 00065 00066 enum IrqType { 00067 RxIrq = 0 00068 , TxIrq 00069 }; 00070 00071 /** Set the transmission format used by the Serial port 00072 * 00073 * @param bits The number of bits in a word (5-8; default = 8) 00074 * @param parity The parity used (Serial::None, Serial::Odd, Serial::Even, Serial::Forced1, Serial::Forced0; default = Serial::None) 00075 * @param stop The number of stop bits (1 or 2; default = 1) 00076 */ 00077 void format(int bits = 8, Parity parity = Serial::None, int stop_bits = 1); 00078 00079 #if 0 // Inhereted from Stream, for documentation only 00080 00081 /** Write a character 00082 * 00083 * @param c The character to write to the serial port 00084 */ 00085 int putc(int c); 00086 00087 /** Reads a character from the serial port. This will block until 00088 * a character is available. To see if a character is available, 00089 * see readable() 00090 * 00091 * @returns 00092 * The character read from the serial port 00093 */ 00094 int getc(); 00095 00096 /** Write a formated string 00097 * 00098 * @param format A printf-style format string, followed by the 00099 * variables to use in formating the string. 00100 */ 00101 int printf(const char* format, ...); 00102 00103 /** Read a formated string 00104 * 00105 * @param format A scanf-style format string, 00106 * followed by the pointers to variables to store the results. 00107 */ 00108 int scanf(const char* format, ...); 00109 00110 #endif 00111 00112 /** Determine if there is a character available to read 00113 * 00114 * @returns 00115 * 1 if there is a character available to read, 00116 * 0 otherwise 00117 */ 00118 int readable(); 00119 00120 /** Determine if there is space available to write a character 00121 * 00122 * @returns 00123 * 1 if there is space to write a character, 00124 * 0 otherwise 00125 */ 00126 int writeable(); 00127 00128 /** Attach a function to call whenever a serial interrupt is generated 00129 * 00130 * @param fptr A pointer to a void function, or 0 to set as none 00131 * @param type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00132 */ 00133 void attach(void (*fptr)(void), IrqType type = RxIrq); 00134 00135 /** Attach a member function to call whenever a serial interrupt is generated 00136 * 00137 * @param tptr pointer to the object to call the member function on 00138 * @param mptr pointer to the member function to be called 00139 * @param type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00140 */ 00141 template<typename T> 00142 void attach(T* tptr, void (T::*mptr)(void), IrqType type = RxIrq) { 00143 if((mptr != NULL) && (tptr != NULL)) { 00144 _irq[type].attach(tptr, mptr); 00145 setup_interrupt(type); 00146 } 00147 } 00148 00149 #ifdef MBED_RPC 00150 virtual const struct rpc_method *get_rpc_methods(); 00151 static struct rpc_class *get_rpc_class(); 00152 #endif 00153 00154 protected: 00155 00156 void setup_interrupt(IrqType type); 00157 void remove_interrupt(IrqType type); 00158 00159 virtual int _getc(); 00160 virtual int _putc(int c); 00161 00162 UARTName _uart; 00163 FunctionPointer _irq[2]; 00164 int _uidx; 00165 00166 }; 00167 00168 } // namespace mbed 00169 00170 #endif 00171 00172 #endif
Generated on Tue Jul 12 2022 11:27:27 by
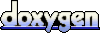