Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
SPI.h
00001 /* mbed Microcontroller Library - SPI 00002 * Copyright (c) 2010-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_SPI_H 00006 #define MBED_SPI_H 00007 00008 #include "device.h" 00009 00010 #if DEVICE_SPI 00011 00012 #include "platform.h" 00013 #include "PinNames.h" 00014 #include "PeripheralNames.h" 00015 #include "Base.h" 00016 00017 namespace mbed { 00018 00019 /** A SPI Master, used for communicating with SPI slave devices 00020 * 00021 * The default format is set to 8-bits, mode 0, and a clock frequency of 1MHz 00022 * 00023 * Most SPI devices will also require Chip Select and Reset signals. These 00024 * can be controlled using <DigitalOut> pins 00025 * 00026 * Example: 00027 * @code 00028 * // Send a byte to a SPI slave, and record the response 00029 * 00030 * #include "mbed.h" 00031 * 00032 * SPI device(p5, p6, p7); // mosi, miso, sclk 00033 * 00034 * int main() { 00035 * int response = device.write(0xFF); 00036 * } 00037 * @endcode 00038 */ 00039 class SPI : public Base { 00040 00041 public: 00042 00043 /** Create a SPI master connected to the specified pins 00044 * 00045 * Pin Options: 00046 * (5, 6, 7) or (11, 12, 13) 00047 * 00048 * mosi or miso can be specfied as NC if not used 00049 * 00050 * @param mosi SPI Master Out, Slave In pin 00051 * @param miso SPI Master In, Slave Out pin 00052 * @param sclk SPI Clock pin 00053 * @param name (optional) A string to identify the object 00054 */ 00055 SPI(PinName mosi, PinName miso, PinName sclk, const char *name = NULL); 00056 00057 /** Configure the data transmission format 00058 * 00059 * @param bits Number of bits per SPI frame (4 - 16) 00060 * @param mode Clock polarity and phase mode (0 - 3) 00061 * 00062 * @code 00063 * mode | POL PHA 00064 * -----+-------- 00065 * 0 | 0 0 00066 * 1 | 0 1 00067 * 2 | 1 0 00068 * 3 | 1 1 00069 * @endcode 00070 */ 00071 void format(int bits, int mode = 0); 00072 00073 /** Set the spi bus clock frequency 00074 * 00075 * @param hz SCLK frequency in hz (default = 1MHz) 00076 */ 00077 void frequency(int hz = 1000000); 00078 00079 /** Write to the SPI Slave and return the response 00080 * 00081 * @param value Data to be sent to the SPI slave 00082 * 00083 * @returns 00084 * Response from the SPI slave 00085 */ 00086 virtual int write(int value); 00087 00088 00089 #ifdef MBED_RPC 00090 virtual const struct rpc_method *get_rpc_methods(); 00091 static struct rpc_class *get_rpc_class(); 00092 #endif 00093 00094 protected: 00095 00096 SPIName _spi; 00097 00098 void aquire(void); 00099 static SPI *_owner; 00100 int _bits; 00101 int _mode; 00102 int _hz; 00103 00104 }; 00105 00106 } // namespace mbed 00107 00108 #endif 00109 00110 #endif
Generated on Tue Jul 12 2022 11:27:27 by
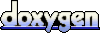