WebSocket client library
Websocket Class Reference
Websocket client Class. More...
#include <Websocket.h>
Public Member Functions | |
Websocket (char *url, Wifly *wifi) | |
Constructor. | |
Websocket (char *url) | |
Constructor for an ethernet communication. | |
bool | connect () |
Connect to the websocket url. | |
void | send (char *str) |
Send a string according to the websocket format. | |
bool | read (char *message) |
Read a websocket message. | |
bool | connected () |
To see if there is a websocket connection active. | |
bool | close () |
Close the websocket connection. | |
std::string | getPath () |
Accessor: get path from the websocket url. |
Detailed Description
Websocket client Class.
Warning: you must use a wifi module (Wifly RN131-C) or an ethernet network to use this class
Example (wifi network):
#include "mbed.h" #include "Wifly.h" #include "Websocket.h" DigitalOut l1(LED1); //Here, we create an instance, with pins 9 and 10 connecting to the //WiFly's TX and RX pins, and pin 21 to RESET. We are connecting to the //"mbed" network, password "password", and we are using WPA. Wifly wifly(p9, p10, p21, "mbed", "password", true); //Here, we create a Websocket instance in 'wo' (write-only) mode //on the 'samux' channel Websocket ws("ws://sockets.mbed.org/ws/samux/wo", &wifly); int main() { while (1) { //we connect the network while (!wifly.join()) { wifly.reset(); } //we connect to the websocket server while (!ws.connect()); while (1) { wait(0.5); //Send Hello world ws.send("Hello World! over Wifi"); // show that we are alive l1 = !l1; } } }
Example (ethernet network):
#include "mbed.h" #include "Websocket.h" Timer tmr; //Here, we create a Websocket instance in 'wo' (write-only) mode //on the 'samux' channel Websocket ws("ws://sockets.mbed.org/ws/samux/wo"); int main() { while (1) { while (!ws.connect()); tmr.start(); while (1) { if (tmr.read() > 0.5) { ws.send("Hello World! over Ethernet"); tmr.start(); } Net::poll(); } } }
Definition at line 122 of file Websocket.h.
Constructor & Destructor Documentation
Websocket | ( | char * | url, |
Wifly * | wifi | ||
) |
Constructor.
- Parameters:
-
url The Websocket url in the form "ws://ip_domain[:port]/path" (by default: port = 80) wifi pointer to a wifi module (the communication will be establish by this module)
Definition at line 6 of file Websocket.cpp.
Websocket | ( | char * | url ) |
Constructor for an ethernet communication.
- Parameters:
-
url The Websocket url in the form "ws://ip_domain[:port]/path" (by default: port = 80)
Definition at line 14 of file Websocket.cpp.
Member Function Documentation
bool close | ( | ) |
Close the websocket connection.
- Returns:
- true if the connection has been closed, false otherwise
Definition at line 445 of file Websocket.cpp.
bool connect | ( | ) |
Connect to the websocket url.
- Returns:
- true if the connection is established, false otherwise
Definition at line 119 of file Websocket.cpp.
bool connected | ( | ) |
To see if there is a websocket connection active.
- Returns:
- true if there is a connection active
Definition at line 482 of file Websocket.cpp.
std::string getPath | ( | ) |
Accessor: get path from the websocket url.
- Returns:
- path
Definition at line 527 of file Websocket.cpp.
bool read | ( | char * | message ) |
Read a websocket message.
- Parameters:
-
message pointer to the string to be read (null if drop frame)
- Returns:
- true if a string has been read, false otherwise
Definition at line 316 of file Websocket.cpp.
void send | ( | char * | str ) |
Send a string according to the websocket format.
- Parameters:
-
str string to be sent
Definition at line 299 of file Websocket.cpp.
Generated on Fri Jul 15 2022 13:24:59 by
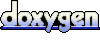