WebSocket client library
Embed:
(wiki syntax)
Show/hide line numbers
Websocket.h
00001 /** 00002 * @author Samuel Mokrani 00003 * 00004 * @section LICENSE 00005 * 00006 * Copyright (c) 2011 mbed 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * 00026 * @section DESCRIPTION 00027 * Simple websocket client 00028 * 00029 */ 00030 00031 #ifndef WEBSOCKET_H 00032 #define WEBSOCKET_H 00033 00034 #include "mbed.h" 00035 #include "Wifly.h" 00036 #include <string> 00037 00038 00039 #ifdef TARGET_LPC1768 00040 #include "EthernetNetIf.h" 00041 #include "TCPSocket.h" 00042 #include "dnsresolve.h" 00043 #endif //target 00044 00045 00046 /** Websocket client Class. 00047 * 00048 * Warning: you must use a wifi module (Wifly RN131-C) or an ethernet network to use this class 00049 * 00050 * Example (wifi network): 00051 * @code 00052 * #include "mbed.h" 00053 * #include "Wifly.h" 00054 * #include "Websocket.h" 00055 * 00056 * DigitalOut l1(LED1); 00057 * 00058 * //Here, we create an instance, with pins 9 and 10 connecting to the 00059 * //WiFly's TX and RX pins, and pin 21 to RESET. We are connecting to the 00060 * //"mbed" network, password "password", and we are using WPA. 00061 * Wifly wifly(p9, p10, p21, "mbed", "password", true); 00062 * 00063 * //Here, we create a Websocket instance in 'wo' (write-only) mode 00064 * //on the 'samux' channel 00065 * Websocket ws("ws://sockets.mbed.org/ws/samux/wo", &wifly); 00066 * 00067 * 00068 * int main() { 00069 * while (1) { 00070 * 00071 * //we connect the network 00072 * while (!wifly.join()) { 00073 * wifly.reset(); 00074 * } 00075 * 00076 * //we connect to the websocket server 00077 * while (!ws.connect()); 00078 * 00079 * while (1) { 00080 * wait(0.5); 00081 * 00082 * //Send Hello world 00083 * ws.send("Hello World! over Wifi"); 00084 * 00085 * // show that we are alive 00086 * l1 = !l1; 00087 * } 00088 * } 00089 * } 00090 * @endcode 00091 * 00092 * 00093 * 00094 * Example (ethernet network): 00095 * @code 00096 * #include "mbed.h" 00097 * #include "Websocket.h" 00098 * 00099 * Timer tmr; 00100 * 00101 * //Here, we create a Websocket instance in 'wo' (write-only) mode 00102 * //on the 'samux' channel 00103 * Websocket ws("ws://sockets.mbed.org/ws/samux/wo"); 00104 * 00105 * int main() { 00106 * while (1) { 00107 * 00108 * while (!ws.connect()); 00109 * 00110 * tmr.start(); 00111 * while (1) { 00112 * if (tmr.read() > 0.5) { 00113 * ws.send("Hello World! over Ethernet"); 00114 * tmr.start(); 00115 * } 00116 * Net::poll(); 00117 * } 00118 * } 00119 * } 00120 * @endcode 00121 */ 00122 class Websocket 00123 { 00124 public: 00125 /** 00126 * Constructor 00127 * 00128 * @param url The Websocket url in the form "ws://ip_domain[:port]/path" (by default: port = 80) 00129 * @param wifi pointer to a wifi module (the communication will be establish by this module) 00130 */ 00131 Websocket(char * url, Wifly * wifi); 00132 00133 #ifdef TARGET_LPC1768 00134 /** 00135 * Constructor for an ethernet communication 00136 * 00137 * @param url The Websocket url in the form "ws://ip_domain[:port]/path" (by default: port = 80) 00138 */ 00139 Websocket(char * url); 00140 #endif //target 00141 00142 /** 00143 * Connect to the websocket url 00144 * 00145 *@return true if the connection is established, false otherwise 00146 */ 00147 bool connect(); 00148 00149 /** 00150 * Send a string according to the websocket format 00151 * 00152 * @param str string to be sent 00153 */ 00154 void send(char * str); 00155 00156 /** 00157 * Read a websocket message 00158 * 00159 * @param message pointer to the string to be read (null if drop frame) 00160 * 00161 * @return true if a string has been read, false otherwise 00162 */ 00163 bool read(char * message); 00164 00165 /** 00166 * To see if there is a websocket connection active 00167 * 00168 * @return true if there is a connection active 00169 */ 00170 bool connected(); 00171 00172 /** 00173 * Close the websocket connection 00174 * 00175 * @return true if the connection has been closed, false otherwise 00176 */ 00177 bool close(); 00178 00179 /** 00180 * Accessor: get path from the websocket url 00181 * 00182 * @return path 00183 */ 00184 std::string getPath(); 00185 00186 enum Type { 00187 WIF, 00188 ETH 00189 }; 00190 00191 00192 private: 00193 00194 00195 void fillFields(char * url); 00196 void sendOpcode(uint8_t opcode); 00197 void sendLength(uint32_t len); 00198 void sendMask(); 00199 void sendChar(uint8_t c); 00200 00201 std::string ip_domain; 00202 std::string path; 00203 std::string port; 00204 00205 Wifly * wifi; 00206 00207 #ifdef TARGET_LPC1768 00208 void onTCPSocketEvent(TCPSocketEvent e); 00209 bool eth_connected; 00210 bool eth_readable; 00211 bool eth_writeable; 00212 char eth_rx[512]; 00213 bool response_server_eth; 00214 bool new_msg; 00215 00216 EthernetNetIf * eth; 00217 TCPSocket * sock; 00218 IpAddr * server_ip; 00219 #endif //target 00220 00221 Type netif; 00222 00223 }; 00224 00225 #endif
Generated on Fri Jul 15 2022 13:24:59 by
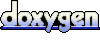