A simple Menu Library
Menu Class Reference
A simple Menu Library
A Menu is light and easy to use.
More...
#include <Menu.h>
Public Member Functions | |
Menu () | |
Construcor of the Menu. | |
void | addProgram (void *output_argument, int(*program)()) |
Add a program to the Menu. | |
void | clean () |
Free the alocated memory of the Bootstrap. | |
int | launch () |
Start the Menu. | |
Protected Member Functions | |
virtual void | startMenu ()=0 |
Start the Menu. | |
virtual bool | isSelectionChanging ()=0 |
Implementation must links an input to know if the user change the selection. | |
virtual bool | isValidating ()=0 |
Implementation must links an input to know if the user validate the selection. | |
virtual void | displaySelectedProgram (void *output_argument)=0 |
Implementation must links an output to show the selected program has changed. |
Detailed Description
A simple Menu Library
A Menu is light and easy to use.
It's composed of a :
- an output interface to show which program is selected,
- a way to change the selection,
- a way to validate the selection and launch the linked program.
If you want a example on how to use it, look at the MenuExample program (http://mbed.org/users/rominos2/code/MenuExample/)
Definition at line 31 of file Menu.h.
Constructor & Destructor Documentation
Member Function Documentation
void addProgram | ( | void * | output_argument, |
int(*)() | program | ||
) |
void clean | ( | ) |
virtual void displaySelectedProgram | ( | void * | output_argument ) | [protected, pure virtual] |
Implementation must links an output to show the selected program has changed.
For example, the color of a LED can be changed or a message printed.
- Parameters:
-
output_argument the argument of the selected program. If NULL, the interface must clear.
virtual bool isSelectionChanging | ( | ) | [protected, pure virtual] |
Implementation must links an input to know if the user change the selection.
For example, a switch can be checked.
- Returns:
- 1 if the selection changes, else false.
virtual bool isValidating | ( | ) | [protected, pure virtual] |
Implementation must links an input to know if the user validate the selection.
For example, a switch can be checked.
- Returns:
- 1 if the user validates, else false.
int launch | ( | ) |
Start the Menu.
First startMenu() is called.
Then the output interface show the chosen program (with the output argument) and the Bootstrap check isSelectionChanging() and isValidating().
Once validate, the function of the program is called.
- Returns:
- the result of the program's function.
virtual void startMenu | ( | ) | [protected, pure virtual] |
Start the Menu.
Implementation can make LED blinks or print a message on a screen for example
Generated on Thu Jul 14 2022 07:00:41 by
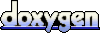