A simple Menu Library
Embed:
(wiki syntax)
Show/hide line numbers
Menu.cpp
00001 /* 00002 Copyright (c) 2014 Romain Berrada 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 furnished to do so, subject to the following conditions: 00009 00010 The above copyright notice and this permission notice shall be included in all copies or 00011 substantial portions of the Software. 00012 00013 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 00020 #include "mbed.h" 00021 #include "Menu.h" 00022 00023 Menu::Program::Program(void* output_argument, int(*program)()) : _output_interface_argument(output_argument), _program(program), _next(NULL) { 00024 } 00025 00026 Menu::Menu() : _first(NULL) { 00027 } 00028 00029 void Menu::addProgram(void* output_argument, int(*program)()) { 00030 Program* prog = new Program(output_argument, program); 00031 if (_first==NULL) { // if first program added 00032 _first = prog; 00033 return; 00034 } else { 00035 Program* temp = _first; 00036 while (temp->_next!=NULL) temp=temp->_next; 00037 temp->_next = prog; 00038 } 00039 } 00040 00041 void Menu::clean() { 00042 Program* prg = _first; 00043 Program* next; 00044 while (prg!=NULL) { 00045 next = prg->_next; 00046 delete prg; 00047 prg = next; 00048 } 00049 } 00050 00051 int Menu::launch() { 00052 Program* choice = _first; 00053 bool done = false; 00054 00055 if (choice==NULL) return -1; // if no program 00056 00057 startMenu(); 00058 displaySelectedProgram(choice->_output_interface_argument); 00059 00060 while(!done) { 00061 if (isSelectionChanging()) { 00062 // change the current choice 00063 choice = choice->_next; 00064 if (choice==NULL) choice = _first; 00065 displaySelectedProgram(choice->_output_interface_argument); 00066 } 00067 00068 if (isValidating()) { 00069 // validate the choice 00070 done = true; 00071 } 00072 00073 wait(0.1); 00074 } 00075 00076 displaySelectedProgram(NULL); 00077 return choice->_program(); // call the program function and return the result 00078 }
Generated on Thu Jul 14 2022 07:00:41 by
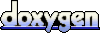