A simple Menu Library
Embed:
(wiki syntax)
Show/hide line numbers
Menu.h
00001 /* 00002 Copyright (c) 2014 Romain Berrada 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 furnished to do so, subject to the following conditions: 00009 00010 The above copyright notice and this permission notice shall be included in all copies or 00011 substantial portions of the Software. 00012 00013 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 00020 #ifndef INCLUDE_MENU_H 00021 #define INCLUDE_MENU_H 00022 00023 /** A simple Menu Library \n 00024 A Menu is light and easy to use. It's composed of a : \n 00025 - an output interface to show which program is selected, \n 00026 - a way to change the selection, \n 00027 - a way to validate the selection and launch the linked program. \n 00028 00029 If you want a example on how to use it, look at the MenuExample program (http://mbed.org/users/rominos2/code/MenuExample/) 00030 */ 00031 class Menu { 00032 private: 00033 /** A Program is reprensented with this nested class. 00034 */ 00035 class Program { 00036 private: 00037 void* _output_interface_argument; /**< the output displayed when this program is seletected. For example, a LED Color or a message. */ 00038 int(*_program)(); /**< the function to call when the program is validated. */ 00039 Program* _next; /**< the next program in the chained list */ 00040 00041 Program(void* output_argument, int(*program)()); /**< Constructor of a Program */ 00042 00043 friend class Menu; 00044 }; 00045 00046 Program* _first; /**< First program of the chained list. */ 00047 00048 protected: 00049 /** Start the Menu. 00050 Implementation can make LED blinks or print a message on a screen for example 00051 */ 00052 virtual void startMenu() = 0; 00053 /** Implementation must links an input to know if the user change the selection. 00054 For example, a switch can be checked. 00055 @return 1 if the selection changes, else false. 00056 */ 00057 virtual bool isSelectionChanging() = 0; 00058 /** Implementation must links an input to know if the user validate the selection. 00059 For example, a switch can be checked. 00060 @return 1 if the user validates, else false. 00061 */ 00062 virtual bool isValidating() = 0; 00063 00064 /** Implementation must links an output to show the selected program has changed. 00065 For example, the color of a LED can be changed or a message printed. 00066 @param output_argument the argument of the selected program. If NULL, the interface must clear. 00067 */ 00068 virtual void displaySelectedProgram(void* output_argument) = 0; 00069 00070 public: 00071 /** Construcor of the Menu. 00072 */ 00073 Menu(); 00074 00075 /** Add a program to the Menu. 00076 @param output_argument the output displayed when this program is seletected. For example, a LED Color or a message. 00077 @param the function to call when the program is validated. 00078 */ 00079 void addProgram(void* output_argument, int(*program)()); 00080 /** Free the alocated memory of the Bootstrap. 00081 All program will be removed from the list. 00082 */ 00083 void clean(); 00084 /** Start the Menu. \n 00085 First startMenu() is called. \n 00086 Then the output interface show the chosen program (with the output argument) and the Bootstrap check isSelectionChanging() and isValidating(). \n 00087 Once validate, the function of the program is called. \n 00088 @return the result of the program's function. 00089 */ 00090 int launch(); 00091 }; 00092 00093 #endif
Generated on Thu Jul 14 2022 07:00:41 by
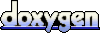