A TextLCD interface for driving 4-bit 16x2 KS0066U LCD
Dependents: KS0066U4_16x2 LAB05_Oppgave4 LAB05_Oppgave2 LAB05_Oppgave3 ... more
TextLCD Class Reference
A TextLCD interface for driving 4-bit 16x2 KS0066U LCD. More...
#include <TextLCD.h>
Public Member Functions | |
TextLCD (PinName rs, PinName rw, PinName e, PinName d4, PinName d5, PinName d6, PinName d7, const char *name=NULL) | |
Create a TextLCD interface and initiated 16x2 char mode. | |
void | lcdComand (unsigned char cmd) |
Writes a Command to the LCD-module. | |
void | lcdData (unsigned char data) |
Writes charecters to the LCD display. | |
void | gotoxy (int, int) |
moves text cursor to a screen column and row | |
int | putc (int c) |
Write a character to the LCD. | |
int | printf (const char *format,...) |
Write a formatted string to the LCD. | |
Protected Member Functions | |
void | writeLcdBitD4toD7 (char data) |
Writes the low nible of data to the LCD-module. | |
void | pulseEn () |
Causes the LCD-module to read the data on the data input pins EN = 1 for L-to-H / EN = 0 for H-to-L. | |
void | init_4BitMode2LinesDisplayOn () |
Enable 4 bit mode From KS0066U Documentation. |
Detailed Description
A TextLCD interface for driving 4-bit 16x2 KS0066U LCD.
Simple example:
#include "mbed.h" #include "TextLCD.h" TextLCD lcd(D11,D10,D9,D5,D4,D3,D2); int main() { lcd.gotoxy(1,1); lcd.printf("Hello"); lcd.gotoxy(1,2); lcd.printf(" World"); while(1) { wait_ms(300); } }
Definition at line 47 of file TextLCD.h.
Constructor & Destructor Documentation
TextLCD | ( | PinName | rs, |
PinName | rw, | ||
PinName | e, | ||
PinName | d4, | ||
PinName | d5, | ||
PinName | d6, | ||
PinName | d7, | ||
const char * | name = NULL |
||
) |
Create a TextLCD interface and initiated 16x2 char mode.
- Parameters:
-
rs Instruction/data control line rw Read/Write (is forced to '1') e Enable line (clock) d4-d7 Data lines for using as a 4-bit interface name I/O stream name (Optional) Stream example:
Definition at line 14 of file TextLCD.cpp.
Member Function Documentation
void gotoxy | ( | int | x, |
int | y | ||
) |
moves text cursor to a screen column and row
- Parameters:
-
column The horizontal position from the left, indexed from 0 row The vertical position from the top, indexed from 0
Definition at line 131 of file TextLCD.cpp.
void init_4BitMode2LinesDisplayOn | ( | ) | [protected] |
Enable 4 bit mode From KS0066U Documentation.
Definition at line 56 of file TextLCD.cpp.
void lcdComand | ( | unsigned char | cmd ) |
Writes a Command to the LCD-module.
- Parameters:
-
cmd command to be sendt to the LCD-Controller
Definition at line 99 of file TextLCD.cpp.
void lcdData | ( | unsigned char | data ) |
Writes charecters to the LCD display.
- Parameters:
-
data char to be sendt to the LCD-Controller
Definition at line 115 of file TextLCD.cpp.
int printf | ( | const char * | format, |
... | |||
) |
Write a formatted string to the LCD.
- Parameters:
-
format A printf-style format string, followed by the variables to use in formatting the string.
void pulseEn | ( | ) | [protected] |
Causes the LCD-module to read the data on the data input pins EN = 1 for L-to-H / EN = 0 for H-to-L.
Definition at line 42 of file TextLCD.cpp.
int putc | ( | int | c ) |
Write a character to the LCD.
- Parameters:
-
c The character to write to the display
void writeLcdBitD4toD7 | ( | char | data ) | [protected] |
Writes the low nible of data to the LCD-module.
- Parameters:
-
data Writes the low-nible to the LCD data pins D4 to D7
Definition at line 50 of file TextLCD.cpp.
Generated on Tue Jul 12 2022 12:54:47 by
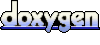