A TextLCD interface for driving 4-bit 16x2 KS0066U LCD
Dependents: KS0066U4_16x2 LAB05_Oppgave4 LAB05_Oppgave2 LAB05_Oppgave3 ... more
TextLCD.cpp
00001 00002 #include "mbed.h" 00003 #include "TextLCD.h" 00004 00005 #define SET_EN() (LCD_EN=1) 00006 #define SET_RS() (LCD_RS=1) 00007 #define SET_RW() (LCD_RW=1) 00008 00009 #define CLEAR_EN() (LCD_EN=0) 00010 #define CLEAR_RS() (LCD_RS=0) 00011 #define CLEAR_RW() (LCD_RW=0) 00012 00013 00014 TextLCD::TextLCD(PinName rs,PinName rw, PinName e, PinName d4, PinName d5, 00015 PinName d6, PinName d7, const char* name) : Stream(name), LCD_RS(rs),LCD_RW(rw), LCD_EN(e), 00016 LCD_D4to7(d4,d5,d6,d7) 00017 { 00018 CLEAR_RW(); 00019 wait_ms(50); // Wait for disp to turn on 00020 00021 CLEAR_EN(); //EN =0 00022 CLEAR_RS(); 00023 00024 init_4BitMode2LinesDisplayOn(); //enabler 4 bit mode 00025 wait_ms(1); 00026 00027 lcdComand(0x00); 00028 wait_us(100); 00029 00030 lcdComand(0x28);//(0b0010 1000); // 4- bit mode LCD 2 line 16x2 Matrix 00031 wait_us(100); 00032 00033 lcdComand(0x01); //(0b00000001); 00034 wait_us(100); 00035 lcdComand(0x06);//(0b00000110); 00036 wait_us(100); 00037 lcdComand(0xC);//(0b0000 1100); 00038 wait_us(100); 00039 } 00040 00041 // Causes the LCD-module to read the data on the data input pins 00042 void TextLCD::pulseEn() 00043 { 00044 SET_EN(); // EN = 1 for L-to-H / 00045 wait_us(200); 00046 CLEAR_EN(); // EN = 0 for H-to-L 00047 } 00048 00049 //Writes the low lible of data to the LCD-module data pins D4 to D7 00050 void TextLCD::writeLcdBitD4toD7(char data) 00051 { 00052 LCD_D4to7=data; 00053 } 00054 00055 //Enable 4 bit mode From KS0066U Documentation 00056 void TextLCD::init_4BitMode2LinesDisplayOn() 00057 { 00058 00059 //Start by selecting configuration mode 00060 CLEAR_RS(); 00061 CLEAR_RW(); 00062 00063 wait(.015); // Wait 150ms to ensure powered up 00064 00065 // send "Display Settings" 3 times (Only top nibble of 0x30 as we've got 4-bit bus) 00066 writeLcdBitD4toD7(0x3); 00067 pulseEn(); 00068 wait_ms(2); 00069 writeLcdBitD4toD7(0x3); 00070 pulseEn(); 00071 wait_ms(2); 00072 writeLcdBitD4toD7(0x3); 00073 pulseEn(); 00074 wait_ms(2); 00075 00076 //Enable 4 bit mode From KS0066U Documentation 00077 writeLcdBitD4toD7(0x2);// (0b0010) 4- bit start / 4-bit mode 00078 wait_us(100); 00079 writeLcdBitD4toD7(0x2); 00080 pulseEn(); 00081 wait_us(100); 00082 writeLcdBitD4toD7(0x2);////(0b0010); // Select 4- bit start (Already on the out port no need to write once more ) 00083 pulseEn(); //LCD exec function 00084 wait_us(50); 00085 00086 writeLcdBitD4toD7(0xC);//(0b1100); // 2 Lines + Disp On 00087 pulseEn(); //LCD exec function 00088 00089 lcdComand(0x01); // Clear Display 00090 wait_ms(3); 00091 lcdComand(0x28); // Function set 001 BW N F - - 00092 lcdComand(0x06); // Cursor Direction and Display Shift : 0000 01 CD S (CD 0-left, 1-right S(hift) 0-no, 1-yes 00093 lcdComand(0x0C); // Dispon + Hide cursor 00094 wait_us(100); 00095 } 00096 00097 00098 //Writes the byte comand to the LCD-module using 4 bits mode 00099 void TextLCD::lcdComand(unsigned char cmd) 00100 { 00101 writeLcdBitD4toD7(cmd>>4); //Write the first high cmd nibble 00102 00103 CLEAR_RS(); // RS = 0 for command 00104 CLEAR_RW(); // RW = 0 for write 00105 00106 pulseEn(); //EN Hi-Lo 00107 00108 writeLcdBitD4toD7(cmd); //Write the second low cmd nibble 00109 00110 pulseEn(); //EN H to Lo 00111 00112 wait_us(100); //wait 00113 } 00114 00115 void TextLCD::lcdData(unsigned char data) 00116 { 00117 writeLcdBitD4toD7(data>>4); //Write the first high data nibble 00118 00119 SET_RS(); // RS = 1 for data 00120 CLEAR_RW(); // RW = 0 for write 00121 pulseEn(); // EN H to Lo 00122 wait_us(100); //wait 00123 00124 writeLcdBitD4toD7(data); 00125 00126 pulseEn(); //EN H to Lo 00127 wait_us(100); //wait 00128 } 00129 00130 //Moves cursor to the X,Y position 00131 void TextLCD::gotoxy(int x, int y) 00132 { 00133 unsigned char firstCharAdr[]= { 0x80,0xc0,0x94,0xD4}; 00134 lcdComand(firstCharAdr[y-1]+ x -1); 00135 00136 wait_us(100); 00137 } 00138 00139 int TextLCD::_putc(int value) 00140 { 00141 lcdData(value); 00142 return value; 00143 } 00144 00145 int TextLCD::_getc() 00146 { 00147 return -1; 00148 }
Generated on Tue Jul 12 2022 12:54:47 by
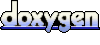