A TextLCD interface for driving 4-bit 16x2 KS0066U LCD
Dependents: KS0066U4_16x2 LAB05_Oppgave4 LAB05_Oppgave2 LAB05_Oppgave3 ... more
TextLCD.h
00001 /* mbed TextLCD Library,4-bit 16x2 LCD for KS0066U 00002 * Copyright (c) 2015 Rune Langøy 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 #ifndef LCD_H_ 00023 #define LCD_H_ 00024 00025 /** A TextLCD interface for driving 4-bit 16x2 KS0066U LCD 00026 * 00027 * Simple example: 00028 * @code 00029 * #include "mbed.h" 00030 * #include "TextLCD.h" 00031 * 00032 * TextLCD lcd(D11,D10,D9,D5,D4,D3,D2); 00033 * int main() 00034 * { 00035 * lcd.gotoxy(1,1); 00036 * lcd.printf("Hello"); 00037 * 00038 * lcd.gotoxy(1,2); 00039 * lcd.printf(" World"); 00040 * 00041 * while(1) { 00042 * wait_ms(300); 00043 * } 00044 * } 00045 * @endcode 00046 */ 00047 class TextLCD : public Stream 00048 { 00049 public: 00050 00051 00052 /** Create a TextLCD interface and initiated 16x2 char mode 00053 * 00054 * @param rs Instruction/data control line 00055 * @param rw Read/Write (is forced to '1') 00056 * @param e Enable line (clock) 00057 * @param d4-d7 Data lines for using as a 4-bit interface 00058 * @param name I/O stream name (Optional) 00059 * Stream example: 00060 * @code 00061 * #include "mbed.h" 00062 * #include "TextLCD.h" 00063 * 00064 * TextLCD lcd(D11,D10,D9,D5,D4,D3,D2,"lcdOut"); 00065 * int main() 00066 * { 00067 * freopen("/lcdOut", "w", stdout); 00068 * printf("Hello World"); 00069 * while(1) { 00070 * wait_ms(300); 00071 * } 00072 * } 00073 * @endcode 00074 */ 00075 TextLCD(PinName rs,PinName rw, PinName e, PinName d4, PinName d5, 00076 PinName d6, PinName d7,const char* name=NULL) ; 00077 00078 /** Writes a Command to the LCD-module 00079 * 00080 * @param cmd command to be sendt to the LCD-Controller 00081 */ 00082 void lcdComand(unsigned char cmd); 00083 00084 00085 /** Writes charecters to the LCD display 00086 * 00087 * @param data char to be sendt to the LCD-Controller 00088 */ 00089 void lcdData(unsigned char data); 00090 00091 /** moves text cursor to a screen column and row 00092 * 00093 * @param column The horizontal position from the left, indexed from 0 00094 * @param row The vertical position from the top, indexed from 0 00095 */ 00096 void gotoxy(int , int ); 00097 #if DOXYGEN_ONLY 00098 /** Write a character to the LCD 00099 * 00100 * @param c The character to write to the display 00101 */ 00102 int putc(int c); 00103 00104 /** Write a formatted string to the LCD 00105 * 00106 * @param format A printf-style format string, followed by the 00107 * variables to use in formatting the string. 00108 */ 00109 int printf(const char* format, ...); 00110 #endif 00111 protected: 00112 /** Writes the low nible of data to the LCD-module 00113 * 00114 * @param data Writes the low-nible to the LCD data pins D4 to D7 00115 */ 00116 void writeLcdBitD4toD7(char data); 00117 00118 /** Causes the LCD-module to read the data on the data input pins 00119 * EN = 1 for L-to-H / 00120 * EN = 0 for H-to-L 00121 */ 00122 void pulseEn(); 00123 00124 /** Enable 4 bit mode From KS0066U Documentation 00125 */ 00126 void init_4BitMode2LinesDisplayOn(); 00127 // Stream implementation functions 00128 virtual int _putc(int value); 00129 virtual int _getc(); 00130 00131 DigitalOut LCD_RS,LCD_RW,LCD_EN; 00132 BusOut LCD_D4to7; 00133 }; 00134 00135 #endif /* LCD_H_ */
Generated on Tue Jul 12 2022 12:54:47 by
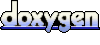