removed unnecessary message by printf (in 32bit register read function)
Fork of PCA9629A by
PCA9629A Class Reference
#include <PCA9629A.h>
Public Types | |
enum | RegisterName { MODE, WDTOI, WDCNTL, IO_CFG, INTMODE, MSK, INTSTAT, IP, INT_MTR_ACT, EXTRASTEPS0, EXTRASTEPS1, OP_CFG_PHS, OP_STAT_TO, RUCNTL, RDCNTL, PMA, LOOPDLY_CW, LOOPDLY_CCW, CWSCOUNTL, CWSCOUNTH, CCWSCOUNTL, CCWSCOUNTH, CWPWL, CWPWH, CCWPWL, CCWPWH, MCNTL, SUBADR1, SUBADR2, SUBADR3, ALLCALLADR, STEPCOUNT0, STEPCOUNT1, STEPCOUNT2, STEPCOUNT3 } |
name of the PCA9629A registers More... | |
enum | RegisterNameFor16bitAccess |
register names for 2 bytes accessing More... | |
enum | RegisterNameFor32bitAccess |
register names for 4 bytes accessing More... | |
enum | Direction { CW = 0, CCW } |
keyword to select direction of rotation More... | |
Public Member Functions | |
PCA9629A (PinName I2C_sda, PinName I2C_scl, char I2C_address=DEFAULT_PCA9629A_ADDR) | |
Create a PCA9629A instance connected to specified I2C pins with specified address. | |
PCA9629A (I2C &i2c_, char I2C_address=DEFAULT_PCA9629A_ADDR) | |
Create a PCA9629A instance connected to specified I2C pins with specified address. | |
~PCA9629A () | |
Destractor. | |
void | software_reset (void) |
Software reset. | |
void | init_registers (void) |
Initialize all registers. | |
void | set_all_registers (char *a, char size) |
Initialize all registers. | |
void | write (RegisterName register_name, char value) |
Write 1 byte data into a register. | |
void | write (RegisterNameFor16bitAccess register_name, short value) |
Write 2 bytes data into a register. | |
char | read (RegisterName register_name) |
Read 1 byte data from a register. | |
short | read (RegisterNameFor16bitAccess register_name) |
Read 2 byte data from registers. | |
long | read (RegisterNameFor32bitAccess register_name) |
Read 4 byte data from registers. | |
void | start (Direction dir) |
Motor start. | |
void | stop (void) |
Motor stop. | |
short | pps (Direction dir, float pulse_per_second) |
Set PPS. | |
void | steps (Direction dir, int step_count) |
Set steps count. | |
void | ramp_up (char rate) |
Ramp-up rate. | |
void | ramp_down (char rate) |
Ramp-down rate. | |
void | register_dump (void) |
Register dump. | |
void | speed_change (unsigned short pw) |
Register dump. | |
void | calibration (float coefficient) |
Register dump. |
Detailed Description
PCA9629A class.
This is a driver code for the PCA9629A stepper motor controller. This class provides interface for PCA9629A operation and accessing its registers. Detail information is available on next URL. http://www.nxp.com/products/interface_and_connectivity/i2c/i2c_bus_controller_and_bridge_ics/PCA9629APW.html
Example:
#include "mbed.h" #include "PCA9629A.h" PCA9629A motor( p28, p27, 0x42 ); // SDA, SCL, I2C_address (option) int main() { // Speed setting 200pps for clockwise (CW) rotation motor.pps( PCA9629A::CW, 200 ); // Set 24 steps for CW motor.steps( PCA9629A::CW, 24 ); // Speed setting 100pps for counterclockwise (CCW) rotation motor.pps( PCA9629A::CCW, 100 ); // Set 48 steps for CCW motor.steps( PCA9629A::CCW, 24 ); while ( 1 ) { motor.start( PCA9629A::CW ); // Motor start for CW wait( 1.0 ); motor.start( PCA9629A::CCW ); // Motor start for CCW wait( 1.0 ); } }
Definition at line 70 of file PCA9629A.h.
Member Enumeration Documentation
enum Direction |
keyword to select direction of rotation
Definition at line 137 of file PCA9629A.h.
enum RegisterName |
name of the PCA9629A registers
- Enumerator:
Definition at line 74 of file PCA9629A.h.
register names for 2 bytes accessing
Definition at line 124 of file PCA9629A.h.
register names for 4 bytes accessing
Definition at line 132 of file PCA9629A.h.
Constructor & Destructor Documentation
PCA9629A | ( | PinName | I2C_sda, |
PinName | I2C_scl, | ||
char | I2C_address = DEFAULT_PCA9629A_ADDR |
||
) |
Create a PCA9629A instance connected to specified I2C pins with specified address.
- Parameters:
-
I2C_sda I2C-bus SDA pin I2C_scl I2C-bus SCL pin I2C_address I2C-bus address (default: 0x42)
Definition at line 40 of file PCA9629A.cpp.
PCA9629A | ( | I2C & | i2c_, |
char | I2C_address = DEFAULT_PCA9629A_ADDR |
||
) |
Create a PCA9629A instance connected to specified I2C pins with specified address.
- Parameters:
-
I2C object (instance) I2C_address I2C-bus address (default: 0x42)
Definition at line 50 of file PCA9629A.cpp.
~PCA9629A | ( | ) |
Destractor.
Definition at line 58 of file PCA9629A.cpp.
Member Function Documentation
void calibration | ( | float | coefficient ) |
Register dump.
Dumping all register data to serial console
void init_registers | ( | void | ) |
Initialize all registers.
The initializing values are defined in the function
Definition at line 72 of file PCA9629A.cpp.
short pps | ( | Direction | dir, |
float | pulse_per_second | ||
) |
Set PPS.
Setting PulsePerSecond This interface can be used to set CWPWx or CCWPWx registers
- Parameters:
-
dir rotate direction ("CW" or "CCW") pulse_per_second pps defineds pulse width for the motor. The pulse width will be 1/pps
- Returns:
- 16 bit data that what set to the CWPWx or CCWPWx registers
Definition at line 222 of file PCA9629A.cpp.
void ramp_down | ( | char | rate ) |
Ramp-down rate.
Setting ramp-down rate
- Parameters:
-
rate set deceleration ratio. From 0 to 13. 0 for disable. Bigger valure decelerate faster.
Definition at line 250 of file PCA9629A.cpp.
void ramp_up | ( | char | rate ) |
Ramp-up rate.
Setting ramp-up rate
- Parameters:
-
rate set acceleration ratio. From 0 to 13. 0 for disable. Bigger valure accelerate faster.
Definition at line 245 of file PCA9629A.cpp.
long read | ( | RegisterNameFor32bitAccess | register_name ) |
Read 4 byte data from registers.
Setting data into a register
- Parameters:
-
register_name the register name: data writing into (it can be "STEPCOUNT")
- Returns:
- read 32 bits data from the registers
Definition at line 168 of file PCA9629A.cpp.
char read | ( | RegisterName | register_name ) |
Read 1 byte data from a register.
Setting data into a register
- Parameters:
-
register_name the register name: data reading from
- Returns:
- read 8 bits data from the register
Definition at line 126 of file PCA9629A.cpp.
short read | ( | RegisterNameFor16bitAccess | register_name ) |
Read 2 byte data from registers.
Setting data into a register
- Parameters:
-
register_name the register name: data writing into (it can be "SROTN_", "CWPW_", "CCWPW_", "CWRCOUNT_", "CCWSCOUNT_", "CWRCOUNT_" or "CCWRCOUNT_" )
- Returns:
- read 16 bits data from the registers
Definition at line 147 of file PCA9629A.cpp.
void register_dump | ( | void | ) |
Register dump.
Dumping all register data to serial console
Definition at line 255 of file PCA9629A.cpp.
void set_all_registers | ( | char * | a, |
char | size | ||
) |
Initialize all registers.
The initializing values are defined in the function
Definition at line 87 of file PCA9629A.cpp.
void software_reset | ( | void | ) |
void speed_change | ( | unsigned short | pw ) |
Register dump.
Dumping all register data to serial console
Definition at line 274 of file PCA9629A.cpp.
void start | ( | Direction | dir ) |
Motor start.
Start command This function starts motor operation with hard-stop flag and rotation+step enabled, no repeat will be performed If custom start is required, use "write( PCA9629A::MCNTL, 0xXX )" to control each bits.
- Parameters:
-
dir rotate direction ("CW" or "CCW")
Definition at line 189 of file PCA9629A.cpp.
void steps | ( | Direction | dir, |
int | step_count | ||
) |
Set steps count.
Setting step count This interfaces CWSCOUNTx and CCWSCOUNTx registers
- Parameters:
-
dir rotate direction ("CW" or "CCW") step_count sets number of steps with 16 bit value
Definition at line 240 of file PCA9629A.cpp.
void stop | ( | void | ) |
void write | ( | RegisterName | register_name, |
char | value | ||
) |
Write 1 byte data into a register.
Setting 8 bits data into a register
- Parameters:
-
register_name the register name: data writing into value 8 bits writing data
Definition at line 97 of file PCA9629A.cpp.
void write | ( | RegisterNameFor16bitAccess | register_name, |
short | value | ||
) |
Write 2 bytes data into a register.
Setting 16 bits data into registers
- Parameters:
-
register_name the register name: data writing into (it can be "SROTN_", "CWPW_", "CCWPW_", "CWRCOUNT_", "CCWSCOUNT_", "CWRCOUNT_" or "CCWRCOUNT_" ) value 16 bits writing data
Definition at line 111 of file PCA9629A.cpp.
Generated on Sun Aug 7 2022 23:26:17 by
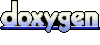