1
ESP8266 Class Reference
ESP8266Interface class. More...
#include <ESP8266.h>
Public Member Functions | |
bool | startup (int mode) |
Startup the ESP8266. | |
bool | reset (void) |
Reset ESP8266. | |
bool | connect (const char *ap, const char *passPhrase) |
Connect ESP8266 to AP. | |
bool | disconnect (void) |
Disconnect ESP8266 from AP. | |
char * | getIPAddress (void) |
Get the IP address of ESP8266. | |
const char * | getMACAddress (void) |
Get the MAC address of ESP8266. | |
bool | isConnected (void) |
Check if ESP8266 is conenected. | |
bool | open (int linkId, int localPort) |
Open a socketed connection. | |
bool | send (const void *data, uint32_t amount, const char *addr, int port) |
Sends data to an open socket. | |
int32_t | recv (void *data, uint32_t amount, char *IP, int *port) |
Receives data from an open socket. | |
bool | close (int id) |
Closes a socket. | |
void | setTimeout (uint32_t timeout_ms) |
Allows timeout to be changed between commands. | |
bool | readable () |
Checks if data is available. | |
bool | writeable () |
Checks if data can be written. |
Detailed Description
ESP8266Interface class.
This is an interface to a ESP8266 radio.
Definition at line 25 of file ESP8266.h.
Member Function Documentation
bool close | ( | int | id ) |
Closes a socket.
- Parameters:
-
id id of socket to close, valid only 0-4
- Returns:
- true only if socket is closed successfully
Definition at line 130 of file ESP8266.cpp.
bool connect | ( | const char * | ap, |
const char * | passPhrase | ||
) |
Connect ESP8266 to AP.
- Parameters:
-
ap the name of the AP passPhrase the password of AP
- Returns:
- true only if ESP8266 is connected successfully
Definition at line 54 of file ESP8266.cpp.
bool disconnect | ( | void | ) |
Disconnect ESP8266 from AP.
- Returns:
- true only if ESP8266 is disconnected successfully
Definition at line 62 of file ESP8266.cpp.
char * getIPAddress | ( | void | ) |
Get the IP address of ESP8266.
- Returns:
- null-teriminated IP address or null if no IP address is assigned
Definition at line 67 of file ESP8266.cpp.
const char * getMACAddress | ( | void | ) |
Get the MAC address of ESP8266.
- Returns:
- null-terminated MAC address or null if no MAC address is assigned
Definition at line 78 of file ESP8266.cpp.
bool isConnected | ( | void | ) |
Check if ESP8266 is conenected.
- Returns:
- true only if the chip has an IP address
Definition at line 89 of file ESP8266.cpp.
bool open | ( | int | linkId, |
int | localPort | ||
) |
Open a socketed connection.
- Parameters:
-
type the type of socket to open "UDP" or "TCP" id id to give the new socket, valid 0-4 port port to open connection with addr the IP address of the destination
- Returns:
- true only if socket opened successfully
Definition at line 94 of file ESP8266.cpp.
bool readable | ( | ) |
Checks if data is available.
Definition at line 148 of file ESP8266.cpp.
int32_t recv | ( | void * | data, |
uint32_t | amount, | ||
char * | IP, | ||
int * | port | ||
) |
Receives data from an open socket.
- Parameters:
-
id id to receive from data placeholder for returned information amount number of bytes to be received
- Returns:
- the number of bytes received
Definition at line 115 of file ESP8266.cpp.
bool reset | ( | void | ) |
Reset ESP8266.
- Returns:
- true only if ESP8266 resets successfully
Definition at line 42 of file ESP8266.cpp.
bool send | ( | const void * | data, |
uint32_t | amount, | ||
const char * | addr, | ||
int | port | ||
) |
Sends data to an open socket.
- Parameters:
-
id id of socket to send to data data to be sent amount amount of data to be sent - max 1024
- Returns:
- true only if data sent successfully
Definition at line 100 of file ESP8266.cpp.
void setTimeout | ( | uint32_t | timeout_ms ) |
Allows timeout to be changed between commands.
- Parameters:
-
timeout_ms timeout of the connection
Definition at line 143 of file ESP8266.cpp.
bool startup | ( | int | mode ) |
Startup the ESP8266.
- Parameters:
-
mode mode of WIFI 1-client, 2-host, 3-both
- Returns:
- true only if ESP8266 was setup correctly
Definition at line 27 of file ESP8266.cpp.
bool writeable | ( | ) |
Checks if data can be written.
Definition at line 153 of file ESP8266.cpp.
Generated on Wed Jul 13 2022 23:24:03 by
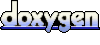