1
Embed:
(wiki syntax)
Show/hide line numbers
ESP8266.h
00001 /* ESP8266Interface Example 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef ESP8266_H 00018 #define ESP8266_H 00019 00020 #include "ATParser.h" 00021 00022 /** ESP8266Interface class. 00023 This is an interface to a ESP8266 radio. 00024 */ 00025 class ESP8266 00026 { 00027 public: 00028 ESP8266(PinName tx, PinName rx, int locOutPort, int locInPort, bool debug=false); 00029 00030 /** 00031 * Startup the ESP8266 00032 * 00033 * @param mode mode of WIFI 1-client, 2-host, 3-both 00034 * @return true only if ESP8266 was setup correctly 00035 */ 00036 bool startup(int mode); 00037 00038 /** 00039 * Reset ESP8266 00040 * 00041 * @return true only if ESP8266 resets successfully 00042 */ 00043 bool reset(void); 00044 00045 /** 00046 * Connect ESP8266 to AP 00047 * 00048 * @param ap the name of the AP 00049 * @param passPhrase the password of AP 00050 * @return true only if ESP8266 is connected successfully 00051 */ 00052 bool connect(const char *ap, const char *passPhrase); 00053 00054 /** 00055 * Disconnect ESP8266 from AP 00056 * 00057 * @return true only if ESP8266 is disconnected successfully 00058 */ 00059 bool disconnect(void); 00060 00061 /** 00062 * Get the IP address of ESP8266 00063 * 00064 * @return null-teriminated IP address or null if no IP address is assigned 00065 */ 00066 char *getIPAddress(void); 00067 00068 /** 00069 * Get the MAC address of ESP8266 00070 * 00071 * @return null-terminated MAC address or null if no MAC address is assigned 00072 */ 00073 const char *getMACAddress(void); 00074 00075 /** 00076 * Check if ESP8266 is conenected 00077 * 00078 * @return true only if the chip has an IP address 00079 */ 00080 bool isConnected(void); 00081 00082 /** 00083 * Open a socketed connection 00084 * 00085 * @param type the type of socket to open "UDP" or "TCP" 00086 * @param id id to give the new socket, valid 0-4 00087 * @param port port to open connection with 00088 * @param addr the IP address of the destination 00089 * @return true only if socket opened successfully 00090 */ 00091 bool open(int linkId, int localPort); 00092 00093 /** 00094 * Sends data to an open socket 00095 * 00096 * @param id id of socket to send to 00097 * @param data data to be sent 00098 * @param amount amount of data to be sent - max 1024 00099 * @return true only if data sent successfully 00100 */ 00101 bool send(const void *data, uint32_t amount, const char* addr, int port); 00102 00103 /** 00104 * Receives data from an open socket 00105 * 00106 * @param id id to receive from 00107 * @param data placeholder for returned information 00108 * @param amount number of bytes to be received 00109 * @return the number of bytes received 00110 */ 00111 int32_t recv(void *data, uint32_t amount, char* IP, int* port); 00112 00113 /** 00114 * Closes a socket 00115 * 00116 * @param id id of socket to close, valid only 0-4 00117 * @return true only if socket is closed successfully 00118 */ 00119 bool close(int id); 00120 00121 /** 00122 * Allows timeout to be changed between commands 00123 * 00124 * @param timeout_ms timeout of the connection 00125 */ 00126 void setTimeout(uint32_t timeout_ms); 00127 00128 /** 00129 * Checks if data is available 00130 */ 00131 bool readable(); 00132 00133 /** 00134 * Checks if data can be written 00135 */ 00136 bool writeable(); 00137 00138 //TODO section 00139 bool setupAP(); 00140 00141 private: 00142 BufferedSerial _serial; 00143 ATParser _parser; 00144 00145 char _ip_buffer[16]; 00146 char _mac_buffer[18]; 00147 00148 int localOutPort; 00149 int localInPort; 00150 }; 00151 00152 #endif
Generated on Wed Jul 13 2022 23:24:03 by
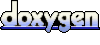