1
Embed:
(wiki syntax)
Show/hide line numbers
ESP8266.cpp
00001 /* ESP8266 Example 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "ESP8266.h" 00018 00019 ESP8266::ESP8266(PinName tx, PinName rx, int locOutPort, int locInPort, bool debug) 00020 : _serial(tx, rx, 1024), _parser(_serial), 00021 localOutPort(locOutPort), localInPort(locInPort) 00022 { 00023 _serial.baud(115200); 00024 _parser.debugOn(debug); 00025 } 00026 00027 bool ESP8266::startup(int mode) 00028 { 00029 if(mode < 1 || mode > 3) { 00030 return false; 00031 } 00032 00033 return reset() 00034 && _parser.send("AT+CWMODE_CUR=%d", mode) 00035 && _parser.recv("OK") 00036 && _parser.send("AT+CIPMUX=1") 00037 && _parser.recv("OK") 00038 && _parser.send("AT+CIPDINFO=1") 00039 && _parser.recv("OK"); 00040 } 00041 00042 bool ESP8266::reset(void) 00043 { 00044 for (int i = 0; i < 2; i++) { 00045 if (_parser.send("AT+RST") 00046 && _parser.recv("OK\r\nready")) { 00047 return true; 00048 } 00049 } 00050 00051 return false; 00052 } 00053 00054 bool ESP8266::connect(const char *ap, const char *passPhrase) 00055 { 00056 setTimeout(20000); 00057 bool b = _parser.send("AT+CWJAP_CUR=\"%s\",\"%s\"", ap, passPhrase) && _parser.recv("OK"); 00058 setTimeout(8000); 00059 return b; 00060 } 00061 00062 bool ESP8266::disconnect(void) 00063 { 00064 return _parser.send("AT+CWQAP") && _parser.recv("OK"); 00065 } 00066 00067 char* ESP8266::getIPAddress(void) 00068 { 00069 if (!(_parser.send("AT+CIFSR") 00070 && _parser.recv("+CIFSR:STAIP,\"%[^\"]\"", _ip_buffer) 00071 && _parser.recv("OK"))) { 00072 return 0; 00073 } 00074 00075 return _ip_buffer; 00076 } 00077 00078 const char* ESP8266::getMACAddress(void) 00079 { 00080 if (!(_parser.send("AT+CIFSR") 00081 && _parser.recv("+CIFSR:STAMAC,\"%[^\"]\"", _mac_buffer) 00082 && _parser.recv("OK"))) { 00083 return 0; 00084 } 00085 00086 return _mac_buffer; 00087 } 00088 00089 bool ESP8266::isConnected(void) 00090 { 00091 return getIPAddress() != 0; 00092 } 00093 00094 bool ESP8266::open(int linkId, int localPort) 00095 { 00096 return _parser.send("AT+CIPSTART=%d,\"%s\",\"%s\",%d,%d,%d", linkId, "UDP","192.168.0.0",49000, localPort, 2) 00097 && _parser.recv("OK"); 00098 } 00099 00100 bool ESP8266::send(const void* data, uint32_t amount, const char* addr, int port) 00101 { 00102 //May take a second try if device is busy 00103 for (unsigned i = 0; i < 4; i++) { 00104 if (_parser.send("AT+CIPSEND=%d,%d,\"%s\",%d", 1, amount, addr, port) 00105 && _parser.recv(">") 00106 && (_parser.write((char*)data, (int)amount) >= 0) 00107 && _parser.recv("SEND OK")) { 00108 return true; 00109 } 00110 } 00111 00112 return false; 00113 } 00114 00115 int32_t ESP8266::recv(void *data, uint32_t amount, char* IP, int* port) 00116 { 00117 uint32_t linkId; 00118 uint32_t recv_amount; 00119 00120 if (!(_parser.recv("+IPD,%d,%d,%[^\,],%d:", &linkId, &recv_amount, IP, port) 00121 && linkId == 2 00122 && recv_amount <= amount 00123 && _parser.read((char*)data, recv_amount))) { 00124 return -1; 00125 } 00126 00127 return recv_amount; 00128 } 00129 00130 bool ESP8266::close(int id) 00131 { 00132 //May take a second try if device is busy 00133 for (unsigned i = 0; i < 2; i++) { 00134 if (_parser.send("AT+CIPCLOSE=%d", id) 00135 && _parser.recv("OK")) { 00136 return true; 00137 } 00138 } 00139 00140 return false; 00141 } 00142 00143 void ESP8266::setTimeout(uint32_t timeout_ms) 00144 { 00145 _parser.setTimeout(timeout_ms); 00146 } 00147 00148 bool ESP8266::readable() 00149 { 00150 return _serial.readable(); 00151 } 00152 00153 bool ESP8266::writeable() 00154 { 00155 return _serial.writeable(); 00156 } 00157 00158 bool ESP8266::setupAP() 00159 { 00160 return _parser.send("AT+CWSAP_CUR=\"RobotClapeyronAP\",\"passpass123\",5,3") 00161 && _parser.recv("OK") 00162 && open(1,localOutPort) 00163 && open(2,localInPort); 00164 } 00165
Generated on Wed Jul 13 2022 23:24:03 by
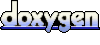