CMSIS DSP library
Dependents: performance_timer Surfboard_ gps2rtty Capstone ... more
arm_scale_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010-2014 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 19. March 2015 00005 * $Revision: V.1.4.5 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_scale_f32.c 00009 * 00010 * Description: Multiplies a floating-point vector by a scalar. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * ---------------------------------------------------------------------------- */ 00040 00041 #include "arm_math.h" 00042 00043 /** 00044 * @ingroup groupMath 00045 */ 00046 00047 /** 00048 * @defgroup scale Vector Scale 00049 * 00050 * Multiply a vector by a scalar value. For floating-point data, the algorithm used is: 00051 * 00052 * <pre> 00053 * pDst[n] = pSrc[n] * scale, 0 <= n < blockSize. 00054 * </pre> 00055 * 00056 * In the fixed-point Q7, Q15, and Q31 functions, <code>scale</code> is represented by 00057 * a fractional multiplication <code>scaleFract</code> and an arithmetic shift <code>shift</code>. 00058 * The shift allows the gain of the scaling operation to exceed 1.0. 00059 * The algorithm used with fixed-point data is: 00060 * 00061 * <pre> 00062 * pDst[n] = (pSrc[n] * scaleFract) << shift, 0 <= n < blockSize. 00063 * </pre> 00064 * 00065 * The overall scale factor applied to the fixed-point data is 00066 * <pre> 00067 * scale = scaleFract * 2^shift. 00068 * </pre> 00069 * 00070 * The functions support in-place computation allowing the source and destination 00071 * pointers to reference the same memory buffer. 00072 */ 00073 00074 /** 00075 * @addtogroup scale 00076 * @{ 00077 */ 00078 00079 /** 00080 * @brief Multiplies a floating-point vector by a scalar. 00081 * @param[in] *pSrc points to the input vector 00082 * @param[in] scale scale factor to be applied 00083 * @param[out] *pDst points to the output vector 00084 * @param[in] blockSize number of samples in the vector 00085 * @return none. 00086 */ 00087 00088 00089 void arm_scale_f32( 00090 float32_t * pSrc, 00091 float32_t scale, 00092 float32_t * pDst, 00093 uint32_t blockSize) 00094 { 00095 uint32_t blkCnt; /* loop counter */ 00096 #ifndef ARM_MATH_CM0_FAMILY 00097 00098 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00099 float32_t in1, in2, in3, in4; /* temporary variabels */ 00100 00101 /*loop Unrolling */ 00102 blkCnt = blockSize >> 2u; 00103 00104 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00105 ** a second loop below computes the remaining 1 to 3 samples. */ 00106 while(blkCnt > 0u) 00107 { 00108 /* C = A * scale */ 00109 /* Scale the input and then store the results in the destination buffer. */ 00110 /* read input samples from source */ 00111 in1 = *pSrc; 00112 in2 = *(pSrc + 1); 00113 00114 /* multiply with scaling factor */ 00115 in1 = in1 * scale; 00116 00117 /* read input sample from source */ 00118 in3 = *(pSrc + 2); 00119 00120 /* multiply with scaling factor */ 00121 in2 = in2 * scale; 00122 00123 /* read input sample from source */ 00124 in4 = *(pSrc + 3); 00125 00126 /* multiply with scaling factor */ 00127 in3 = in3 * scale; 00128 in4 = in4 * scale; 00129 /* store the result to destination */ 00130 *pDst = in1; 00131 *(pDst + 1) = in2; 00132 *(pDst + 2) = in3; 00133 *(pDst + 3) = in4; 00134 00135 /* update pointers to process next samples */ 00136 pSrc += 4u; 00137 pDst += 4u; 00138 00139 /* Decrement the loop counter */ 00140 blkCnt--; 00141 } 00142 00143 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00144 ** No loop unrolling is used. */ 00145 blkCnt = blockSize % 0x4u; 00146 00147 #else 00148 00149 /* Run the below code for Cortex-M0 */ 00150 00151 /* Initialize blkCnt with number of samples */ 00152 blkCnt = blockSize; 00153 00154 #endif /* #ifndef ARM_MATH_CM0_FAMILY */ 00155 00156 while(blkCnt > 0u) 00157 { 00158 /* C = A * scale */ 00159 /* Scale the input and then store the result in the destination buffer. */ 00160 *pDst++ = (*pSrc++) * scale; 00161 00162 /* Decrement the loop counter */ 00163 blkCnt--; 00164 } 00165 } 00166 00167 /** 00168 * @} end of scale group 00169 */
Generated on Tue Jul 12 2022 11:59:19 by
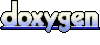