CMSIS DSP library
Dependents: performance_timer Surfboard_ gps2rtty Capstone ... more
arm_q31_to_float.c
00001 /* ---------------------------------------------------------------------------- 00002 * Copyright (C) 2010-2014 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 19. March 2015 00005 * $Revision: V.1.4.5 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_q31_to_float.c 00009 * 00010 * Description: Converts the elements of the Q31 vector to floating-point vector. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * ---------------------------------------------------------------------------- */ 00040 00041 #include "arm_math.h" 00042 00043 /** 00044 * @ingroup groupSupport 00045 */ 00046 00047 /** 00048 * @defgroup q31_to_x Convert 32-bit Integer value 00049 */ 00050 00051 /** 00052 * @addtogroup q31_to_x 00053 * @{ 00054 */ 00055 00056 /** 00057 * @brief Converts the elements of the Q31 vector to floating-point vector. 00058 * @param[in] *pSrc points to the Q31 input vector 00059 * @param[out] *pDst points to the floating-point output vector 00060 * @param[in] blockSize length of the input vector 00061 * @return none. 00062 * 00063 * \par Description: 00064 * 00065 * The equation used for the conversion process is: 00066 * 00067 * <pre> 00068 * pDst[n] = (float32_t) pSrc[n] / 2147483648; 0 <= n < blockSize. 00069 * </pre> 00070 * 00071 */ 00072 00073 00074 void arm_q31_to_float( 00075 q31_t * pSrc, 00076 float32_t * pDst, 00077 uint32_t blockSize) 00078 { 00079 q31_t *pIn = pSrc; /* Src pointer */ 00080 uint32_t blkCnt; /* loop counter */ 00081 00082 00083 #ifndef ARM_MATH_CM0_FAMILY 00084 00085 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00086 00087 /*loop Unrolling */ 00088 blkCnt = blockSize >> 2u; 00089 00090 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00091 ** a second loop below computes the remaining 1 to 3 samples. */ 00092 while(blkCnt > 0u) 00093 { 00094 /* C = (float32_t) A / 2147483648 */ 00095 /* convert from q31 to float and then store the results in the destination buffer */ 00096 *pDst++ = ((float32_t) * pIn++ / 2147483648.0f); 00097 *pDst++ = ((float32_t) * pIn++ / 2147483648.0f); 00098 *pDst++ = ((float32_t) * pIn++ / 2147483648.0f); 00099 *pDst++ = ((float32_t) * pIn++ / 2147483648.0f); 00100 00101 /* Decrement the loop counter */ 00102 blkCnt--; 00103 } 00104 00105 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00106 ** No loop unrolling is used. */ 00107 blkCnt = blockSize % 0x4u; 00108 00109 #else 00110 00111 /* Run the below code for Cortex-M0 */ 00112 00113 /* Loop over blockSize number of values */ 00114 blkCnt = blockSize; 00115 00116 #endif /* #ifndef ARM_MATH_CM0_FAMILY */ 00117 00118 while(blkCnt > 0u) 00119 { 00120 /* C = (float32_t) A / 2147483648 */ 00121 /* convert from q31 to float and then store the results in the destination buffer */ 00122 *pDst++ = ((float32_t) * pIn++ / 2147483648.0f); 00123 00124 /* Decrement the loop counter */ 00125 blkCnt--; 00126 } 00127 } 00128 00129 /** 00130 * @} end of q31_to_x group 00131 */
Generated on Tue Jul 12 2022 11:59:18 by
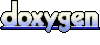