CMSIS DSP library
Dependents: performance_timer Surfboard_ gps2rtty Capstone ... more
arm_mult_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010-2014 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 19. March 2015 00005 * $Revision: V.1.4.5 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_mult_f32.c 00009 * 00010 * Description: Floating-point vector multiplication. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * -------------------------------------------------------------------- */ 00040 00041 #include "arm_math.h" 00042 00043 /** 00044 * @ingroup groupMath 00045 */ 00046 00047 /** 00048 * @defgroup BasicMult Vector Multiplication 00049 * 00050 * Element-by-element multiplication of two vectors. 00051 * 00052 * <pre> 00053 * pDst[n] = pSrcA[n] * pSrcB[n], 0 <= n < blockSize. 00054 * </pre> 00055 * 00056 * There are separate functions for floating-point, Q7, Q15, and Q31 data types. 00057 */ 00058 00059 /** 00060 * @addtogroup BasicMult 00061 * @{ 00062 */ 00063 00064 /** 00065 * @brief Floating-point vector multiplication. 00066 * @param[in] *pSrcA points to the first input vector 00067 * @param[in] *pSrcB points to the second input vector 00068 * @param[out] *pDst points to the output vector 00069 * @param[in] blockSize number of samples in each vector 00070 * @return none. 00071 */ 00072 00073 void arm_mult_f32( 00074 float32_t * pSrcA, 00075 float32_t * pSrcB, 00076 float32_t * pDst, 00077 uint32_t blockSize) 00078 { 00079 uint32_t blkCnt; /* loop counters */ 00080 #ifndef ARM_MATH_CM0_FAMILY 00081 00082 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00083 float32_t inA1, inA2, inA3, inA4; /* temporary input variables */ 00084 float32_t inB1, inB2, inB3, inB4; /* temporary input variables */ 00085 float32_t out1, out2, out3, out4; /* temporary output variables */ 00086 00087 /* loop Unrolling */ 00088 blkCnt = blockSize >> 2u; 00089 00090 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00091 ** a second loop below computes the remaining 1 to 3 samples. */ 00092 while(blkCnt > 0u) 00093 { 00094 /* C = A * B */ 00095 /* Multiply the inputs and store the results in output buffer */ 00096 /* read sample from sourceA */ 00097 inA1 = *pSrcA; 00098 /* read sample from sourceB */ 00099 inB1 = *pSrcB; 00100 /* read sample from sourceA */ 00101 inA2 = *(pSrcA + 1); 00102 /* read sample from sourceB */ 00103 inB2 = *(pSrcB + 1); 00104 00105 /* out = sourceA * sourceB */ 00106 out1 = inA1 * inB1; 00107 00108 /* read sample from sourceA */ 00109 inA3 = *(pSrcA + 2); 00110 /* read sample from sourceB */ 00111 inB3 = *(pSrcB + 2); 00112 00113 /* out = sourceA * sourceB */ 00114 out2 = inA2 * inB2; 00115 00116 /* read sample from sourceA */ 00117 inA4 = *(pSrcA + 3); 00118 00119 /* store result to destination buffer */ 00120 *pDst = out1; 00121 00122 /* read sample from sourceB */ 00123 inB4 = *(pSrcB + 3); 00124 00125 /* out = sourceA * sourceB */ 00126 out3 = inA3 * inB3; 00127 00128 /* store result to destination buffer */ 00129 *(pDst + 1) = out2; 00130 00131 /* out = sourceA * sourceB */ 00132 out4 = inA4 * inB4; 00133 /* store result to destination buffer */ 00134 *(pDst + 2) = out3; 00135 /* store result to destination buffer */ 00136 *(pDst + 3) = out4; 00137 00138 00139 /* update pointers to process next samples */ 00140 pSrcA += 4u; 00141 pSrcB += 4u; 00142 pDst += 4u; 00143 00144 /* Decrement the blockSize loop counter */ 00145 blkCnt--; 00146 } 00147 00148 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00149 ** No loop unrolling is used. */ 00150 blkCnt = blockSize % 0x4u; 00151 00152 #else 00153 00154 /* Run the below code for Cortex-M0 */ 00155 00156 /* Initialize blkCnt with number of samples */ 00157 blkCnt = blockSize; 00158 00159 #endif /* #ifndef ARM_MATH_CM0_FAMILY */ 00160 00161 while(blkCnt > 0u) 00162 { 00163 /* C = A * B */ 00164 /* Multiply the inputs and store the results in output buffer */ 00165 *pDst++ = (*pSrcA++) * (*pSrcB++); 00166 00167 /* Decrement the blockSize loop counter */ 00168 blkCnt--; 00169 } 00170 } 00171 00172 /** 00173 * @} end of BasicMult group 00174 */
Generated on Tue Jul 12 2022 11:59:18 by
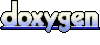