CMSIS DSP library
Dependents: performance_timer Surfboard_ gps2rtty Capstone ... more
arm_min_q7.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010-2014 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 19. March 2015 00005 * $Revision: V.1.4.5 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_min_q7.c 00009 * 00010 * Description: Minimum value of a Q7 vector. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * ---------------------------------------------------------------------------- */ 00040 00041 #include "arm_math.h" 00042 00043 /** 00044 * @ingroup groupStats 00045 */ 00046 00047 /** 00048 * @addtogroup Min 00049 * @{ 00050 */ 00051 00052 00053 /** 00054 * @brief Minimum value of a Q7 vector. 00055 * @param[in] *pSrc points to the input vector 00056 * @param[in] blockSize length of the input vector 00057 * @param[out] *pResult minimum value returned here 00058 * @param[out] *pIndex index of minimum value returned here 00059 * @return none. 00060 * 00061 */ 00062 00063 void arm_min_q7( 00064 q7_t * pSrc, 00065 uint32_t blockSize, 00066 q7_t * pResult, 00067 uint32_t * pIndex) 00068 { 00069 #ifndef ARM_MATH_CM0_FAMILY 00070 00071 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00072 00073 q7_t minVal1, minVal2, out; /* Temporary variables to store the output value. */ 00074 uint32_t blkCnt, outIndex, count; /* loop counter */ 00075 00076 /* Initialise the count value. */ 00077 count = 0u; 00078 /* Initialise the index value to zero. */ 00079 outIndex = 0u; 00080 /* Load first input value that act as reference value for comparision */ 00081 out = *pSrc++; 00082 00083 /* Loop unrolling */ 00084 blkCnt = (blockSize - 1u) >> 2u; 00085 00086 while(blkCnt > 0) 00087 { 00088 /* Initialize minVal to the next consecutive values one by one */ 00089 minVal1 = *pSrc++; 00090 minVal2 = *pSrc++; 00091 00092 /* compare for the minimum value */ 00093 if(out > minVal1) 00094 { 00095 /* Update the minimum value and its index */ 00096 out = minVal1; 00097 outIndex = count + 1u; 00098 } 00099 00100 minVal1 = *pSrc++; 00101 00102 /* compare for the minimum value */ 00103 if(out > minVal2) 00104 { 00105 /* Update the minimum value and its index */ 00106 out = minVal2; 00107 outIndex = count + 2u; 00108 } 00109 00110 minVal2 = *pSrc++; 00111 00112 /* compare for the minimum value */ 00113 if(out > minVal1) 00114 { 00115 /* Update the minimum value and its index */ 00116 out = minVal1; 00117 outIndex = count + 3u; 00118 } 00119 00120 /* compare for the minimum value */ 00121 if(out > minVal2) 00122 { 00123 /* Update the minimum value and its index */ 00124 out = minVal2; 00125 outIndex = count + 4u; 00126 } 00127 00128 count += 4u; 00129 00130 blkCnt--; 00131 } 00132 00133 /* if (blockSize - 1u ) is not multiple of 4 */ 00134 blkCnt = (blockSize - 1u) % 4u; 00135 00136 #else 00137 00138 /* Run the below code for Cortex-M0 */ 00139 00140 q7_t minVal1, out; /* Temporary variables to store the output value. */ 00141 uint32_t blkCnt, outIndex; /* loop counter */ 00142 00143 /* Initialise the index value to zero. */ 00144 outIndex = 0u; 00145 /* Load first input value that act as reference value for comparision */ 00146 out = *pSrc++; 00147 00148 blkCnt = (blockSize - 1u); 00149 00150 #endif // #ifndef ARM_MATH_CM0_FAMILY 00151 00152 while(blkCnt > 0) 00153 { 00154 /* Initialize minVal to the next consecutive values one by one */ 00155 minVal1 = *pSrc++; 00156 00157 /* compare for the minimum value */ 00158 if(out > minVal1) 00159 { 00160 /* Update the minimum value and it's index */ 00161 out = minVal1; 00162 outIndex = blockSize - blkCnt; 00163 } 00164 00165 blkCnt--; 00166 00167 } 00168 00169 /* Store the minimum value and its index into destination pointers */ 00170 *pResult = out; 00171 *pIndex = outIndex; 00172 00173 00174 } 00175 00176 /** 00177 * @} end of Min group 00178 */
Generated on Tue Jul 12 2022 11:59:18 by
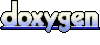