CMSIS DSP library
Dependents: performance_timer Surfboard_ gps2rtty Capstone ... more
arm_max_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010-2014 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 19. March 2015 00005 * $Revision: V.1.4.5 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_max_f32.c 00009 * 00010 * Description: Maximum value of a floating-point vector. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * ---------------------------------------------------------------------------- */ 00040 00041 #include "arm_math.h" 00042 00043 /** 00044 * @ingroup groupStats 00045 */ 00046 00047 /** 00048 * @defgroup Max Maximum 00049 * 00050 * Computes the maximum value of an array of data. 00051 * The function returns both the maximum value and its position within the array. 00052 * There are separate functions for floating-point, Q31, Q15, and Q7 data types. 00053 */ 00054 00055 /** 00056 * @addtogroup Max 00057 * @{ 00058 */ 00059 00060 00061 /** 00062 * @brief Maximum value of a floating-point vector. 00063 * @param[in] *pSrc points to the input vector 00064 * @param[in] blockSize length of the input vector 00065 * @param[out] *pResult maximum value returned here 00066 * @param[out] *pIndex index of maximum value returned here 00067 * @return none. 00068 */ 00069 00070 void arm_max_f32( 00071 float32_t * pSrc, 00072 uint32_t blockSize, 00073 float32_t * pResult, 00074 uint32_t * pIndex) 00075 { 00076 #ifndef ARM_MATH_CM0_FAMILY 00077 00078 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00079 float32_t maxVal1, maxVal2, out; /* Temporary variables to store the output value. */ 00080 uint32_t blkCnt, outIndex, count; /* loop counter */ 00081 00082 /* Initialise the count value. */ 00083 count = 0u; 00084 /* Initialise the index value to zero. */ 00085 outIndex = 0u; 00086 /* Load first input value that act as reference value for comparision */ 00087 out = *pSrc++; 00088 00089 /* Loop unrolling */ 00090 blkCnt = (blockSize - 1u) >> 2u; 00091 00092 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00093 while(blkCnt > 0u) 00094 { 00095 /* Initialize maxVal to the next consecutive values one by one */ 00096 maxVal1 = *pSrc++; 00097 00098 maxVal2 = *pSrc++; 00099 00100 /* compare for the maximum value */ 00101 if(out < maxVal1) 00102 { 00103 /* Update the maximum value and its index */ 00104 out = maxVal1; 00105 outIndex = count + 1u; 00106 } 00107 00108 maxVal1 = *pSrc++; 00109 00110 /* compare for the maximum value */ 00111 if(out < maxVal2) 00112 { 00113 /* Update the maximum value and its index */ 00114 out = maxVal2; 00115 outIndex = count + 2u; 00116 } 00117 00118 maxVal2 = *pSrc++; 00119 00120 /* compare for the maximum value */ 00121 if(out < maxVal1) 00122 { 00123 /* Update the maximum value and its index */ 00124 out = maxVal1; 00125 outIndex = count + 3u; 00126 } 00127 00128 /* compare for the maximum value */ 00129 if(out < maxVal2) 00130 { 00131 /* Update the maximum value and its index */ 00132 out = maxVal2; 00133 outIndex = count + 4u; 00134 } 00135 00136 count += 4u; 00137 00138 /* Decrement the loop counter */ 00139 blkCnt--; 00140 } 00141 00142 /* if (blockSize - 1u) is not multiple of 4 */ 00143 blkCnt = (blockSize - 1u) % 4u; 00144 00145 #else 00146 00147 /* Run the below code for Cortex-M0 */ 00148 float32_t maxVal1, out; /* Temporary variables to store the output value. */ 00149 uint32_t blkCnt, outIndex; /* loop counter */ 00150 00151 /* Initialise the index value to zero. */ 00152 outIndex = 0u; 00153 /* Load first input value that act as reference value for comparision */ 00154 out = *pSrc++; 00155 00156 blkCnt = (blockSize - 1u); 00157 00158 #endif /* #ifndef ARM_MATH_CM0_FAMILY */ 00159 00160 while(blkCnt > 0u) 00161 { 00162 /* Initialize maxVal to the next consecutive values one by one */ 00163 maxVal1 = *pSrc++; 00164 00165 /* compare for the maximum value */ 00166 if(out < maxVal1) 00167 { 00168 /* Update the maximum value and it's index */ 00169 out = maxVal1; 00170 outIndex = blockSize - blkCnt; 00171 } 00172 00173 00174 /* Decrement the loop counter */ 00175 blkCnt--; 00176 00177 } 00178 00179 /* Store the maximum value and it's index into destination pointers */ 00180 *pResult = out; 00181 *pIndex = outIndex; 00182 } 00183 00184 /** 00185 * @} end of Max group 00186 */
Generated on Tue Jul 12 2022 11:59:18 by
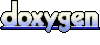