CMSIS DSP library
Dependents: performance_timer Surfboard_ gps2rtty Capstone ... more
arm_mat_sub_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010-2014 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 19. March 2015 00005 * $Revision: V.1.4.5 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_mat_sub_f32.c 00009 * 00010 * Description: Floating-point matrix subtraction. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * -------------------------------------------------------------------- */ 00040 00041 #include "arm_math.h" 00042 00043 /** 00044 * @ingroup groupMatrix 00045 */ 00046 00047 /** 00048 * @defgroup MatrixSub Matrix Subtraction 00049 * 00050 * Subtract two matrices. 00051 * \image html MatrixSubtraction.gif "Subraction of two 3 x 3 matrices" 00052 * 00053 * The functions check to make sure that 00054 * <code>pSrcA</code>, <code>pSrcB</code>, and <code>pDst</code> have the same 00055 * number of rows and columns. 00056 */ 00057 00058 /** 00059 * @addtogroup MatrixSub 00060 * @{ 00061 */ 00062 00063 /** 00064 * @brief Floating-point matrix subtraction 00065 * @param[in] *pSrcA points to the first input matrix structure 00066 * @param[in] *pSrcB points to the second input matrix structure 00067 * @param[out] *pDst points to output matrix structure 00068 * @return The function returns either 00069 * <code>ARM_MATH_SIZE_MISMATCH</code> or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 00070 */ 00071 00072 arm_status arm_mat_sub_f32( 00073 const arm_matrix_instance_f32 * pSrcA, 00074 const arm_matrix_instance_f32 * pSrcB, 00075 arm_matrix_instance_f32 * pDst) 00076 { 00077 float32_t *pIn1 = pSrcA->pData; /* input data matrix pointer A */ 00078 float32_t *pIn2 = pSrcB->pData; /* input data matrix pointer B */ 00079 float32_t *pOut = pDst->pData; /* output data matrix pointer */ 00080 00081 #ifndef ARM_MATH_CM0_FAMILY 00082 00083 float32_t inA1, inA2, inB1, inB2, out1, out2; /* temporary variables */ 00084 00085 #endif // #ifndef ARM_MATH_CM0_FAMILY 00086 00087 uint32_t numSamples; /* total number of elements in the matrix */ 00088 uint32_t blkCnt; /* loop counters */ 00089 arm_status status; /* status of matrix subtraction */ 00090 00091 #ifdef ARM_MATH_MATRIX_CHECK 00092 /* Check for matrix mismatch condition */ 00093 if((pSrcA->numRows != pSrcB->numRows) || 00094 (pSrcA->numCols != pSrcB->numCols) || 00095 (pSrcA->numRows != pDst->numRows) || (pSrcA->numCols != pDst->numCols)) 00096 { 00097 /* Set status as ARM_MATH_SIZE_MISMATCH */ 00098 status = ARM_MATH_SIZE_MISMATCH; 00099 } 00100 else 00101 #endif /* #ifdef ARM_MATH_MATRIX_CHECK */ 00102 { 00103 /* Total number of samples in the input matrix */ 00104 numSamples = (uint32_t) pSrcA->numRows * pSrcA->numCols; 00105 00106 #ifndef ARM_MATH_CM0_FAMILY 00107 00108 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00109 00110 /* Loop Unrolling */ 00111 blkCnt = numSamples >> 2u; 00112 00113 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00114 ** a second loop below computes the remaining 1 to 3 samples. */ 00115 while(blkCnt > 0u) 00116 { 00117 /* C(m,n) = A(m,n) - B(m,n) */ 00118 /* Subtract and then store the results in the destination buffer. */ 00119 /* Read values from source A */ 00120 inA1 = pIn1[0]; 00121 00122 /* Read values from source B */ 00123 inB1 = pIn2[0]; 00124 00125 /* Read values from source A */ 00126 inA2 = pIn1[1]; 00127 00128 /* out = sourceA - sourceB */ 00129 out1 = inA1 - inB1; 00130 00131 /* Read values from source B */ 00132 inB2 = pIn2[1]; 00133 00134 /* Read values from source A */ 00135 inA1 = pIn1[2]; 00136 00137 /* out = sourceA - sourceB */ 00138 out2 = inA2 - inB2; 00139 00140 /* Read values from source B */ 00141 inB1 = pIn2[2]; 00142 00143 /* Store result in destination */ 00144 pOut[0] = out1; 00145 pOut[1] = out2; 00146 00147 /* Read values from source A */ 00148 inA2 = pIn1[3]; 00149 00150 /* Read values from source B */ 00151 inB2 = pIn2[3]; 00152 00153 /* out = sourceA - sourceB */ 00154 out1 = inA1 - inB1; 00155 00156 00157 /* out = sourceA - sourceB */ 00158 out2 = inA2 - inB2; 00159 00160 /* Store result in destination */ 00161 pOut[2] = out1; 00162 00163 /* Store result in destination */ 00164 pOut[3] = out2; 00165 00166 00167 /* update pointers to process next sampels */ 00168 pIn1 += 4u; 00169 pIn2 += 4u; 00170 pOut += 4u; 00171 00172 /* Decrement the loop counter */ 00173 blkCnt--; 00174 } 00175 00176 /* If the numSamples is not a multiple of 4, compute any remaining output samples here. 00177 ** No loop unrolling is used. */ 00178 blkCnt = numSamples % 0x4u; 00179 00180 #else 00181 00182 /* Run the below code for Cortex-M0 */ 00183 00184 /* Initialize blkCnt with number of samples */ 00185 blkCnt = numSamples; 00186 00187 #endif /* #ifndef ARM_MATH_CM0_FAMILY */ 00188 00189 while(blkCnt > 0u) 00190 { 00191 /* C(m,n) = A(m,n) - B(m,n) */ 00192 /* Subtract and then store the results in the destination buffer. */ 00193 *pOut++ = (*pIn1++) - (*pIn2++); 00194 00195 /* Decrement the loop counter */ 00196 blkCnt--; 00197 } 00198 00199 /* Set status as ARM_MATH_SUCCESS */ 00200 status = ARM_MATH_SUCCESS; 00201 } 00202 00203 /* Return to application */ 00204 return (status); 00205 } 00206 00207 /** 00208 * @} end of MatrixSub group 00209 */
Generated on Tue Jul 12 2022 11:59:18 by
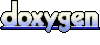