CMSIS DSP library
Dependents: performance_timer Surfboard_ gps2rtty Capstone ... more
arm_fir_lattice_q15.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010-2014 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 19. March 2015 00005 * $Revision: V.1.4.5 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_fir_lattice_q15.c 00009 * 00010 * Description: Q15 FIR lattice filter processing function. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * -------------------------------------------------------------------- */ 00040 00041 #include "arm_math.h" 00042 00043 /** 00044 * @ingroup groupFilters 00045 */ 00046 00047 /** 00048 * @addtogroup FIR_Lattice 00049 * @{ 00050 */ 00051 00052 00053 /** 00054 * @brief Processing function for the Q15 FIR lattice filter. 00055 * @param[in] *S points to an instance of the Q15 FIR lattice structure. 00056 * @param[in] *pSrc points to the block of input data. 00057 * @param[out] *pDst points to the block of output data 00058 * @param[in] blockSize number of samples to process. 00059 * @return none. 00060 */ 00061 00062 void arm_fir_lattice_q15( 00063 const arm_fir_lattice_instance_q15 * S, 00064 q15_t * pSrc, 00065 q15_t * pDst, 00066 uint32_t blockSize) 00067 { 00068 q15_t *pState; /* State pointer */ 00069 q15_t *pCoeffs = S->pCoeffs; /* Coefficient pointer */ 00070 q15_t *px; /* temporary state pointer */ 00071 q15_t *pk; /* temporary coefficient pointer */ 00072 00073 00074 #ifndef ARM_MATH_CM0_FAMILY 00075 00076 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00077 00078 q31_t fcurnt1, fnext1, gcurnt1 = 0, gnext1; /* temporary variables for first sample in loop unrolling */ 00079 q31_t fcurnt2, fnext2, gnext2; /* temporary variables for second sample in loop unrolling */ 00080 q31_t fcurnt3, fnext3, gnext3; /* temporary variables for third sample in loop unrolling */ 00081 q31_t fcurnt4, fnext4, gnext4; /* temporary variables for fourth sample in loop unrolling */ 00082 uint32_t numStages = S->numStages; /* Number of stages in the filter */ 00083 uint32_t blkCnt, stageCnt; /* temporary variables for counts */ 00084 00085 pState = &S->pState[0]; 00086 00087 blkCnt = blockSize >> 2u; 00088 00089 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00090 ** a second loop below computes the remaining 1 to 3 samples. */ 00091 while(blkCnt > 0u) 00092 { 00093 00094 /* Read two samples from input buffer */ 00095 /* f0(n) = x(n) */ 00096 fcurnt1 = *pSrc++; 00097 fcurnt2 = *pSrc++; 00098 00099 /* Initialize coeff pointer */ 00100 pk = (pCoeffs); 00101 00102 /* Initialize state pointer */ 00103 px = pState; 00104 00105 /* Read g0(n-1) from state */ 00106 gcurnt1 = *px; 00107 00108 /* Process first sample for first tap */ 00109 /* f1(n) = f0(n) + K1 * g0(n-1) */ 00110 fnext1 = (q31_t) ((gcurnt1 * (*pk)) >> 15u) + fcurnt1; 00111 fnext1 = __SSAT(fnext1, 16); 00112 00113 /* g1(n) = f0(n) * K1 + g0(n-1) */ 00114 gnext1 = (q31_t) ((fcurnt1 * (*pk)) >> 15u) + gcurnt1; 00115 gnext1 = __SSAT(gnext1, 16); 00116 00117 /* Process second sample for first tap */ 00118 /* for sample 2 processing */ 00119 fnext2 = (q31_t) ((fcurnt1 * (*pk)) >> 15u) + fcurnt2; 00120 fnext2 = __SSAT(fnext2, 16); 00121 00122 gnext2 = (q31_t) ((fcurnt2 * (*pk)) >> 15u) + fcurnt1; 00123 gnext2 = __SSAT(gnext2, 16); 00124 00125 00126 /* Read next two samples from input buffer */ 00127 /* f0(n+2) = x(n+2) */ 00128 fcurnt3 = *pSrc++; 00129 fcurnt4 = *pSrc++; 00130 00131 /* Copy only last input samples into the state buffer 00132 which is used for next four samples processing */ 00133 *px++ = (q15_t) fcurnt4; 00134 00135 /* Process third sample for first tap */ 00136 fnext3 = (q31_t) ((fcurnt2 * (*pk)) >> 15u) + fcurnt3; 00137 fnext3 = __SSAT(fnext3, 16); 00138 gnext3 = (q31_t) ((fcurnt3 * (*pk)) >> 15u) + fcurnt2; 00139 gnext3 = __SSAT(gnext3, 16); 00140 00141 /* Process fourth sample for first tap */ 00142 fnext4 = (q31_t) ((fcurnt3 * (*pk)) >> 15u) + fcurnt4; 00143 fnext4 = __SSAT(fnext4, 16); 00144 gnext4 = (q31_t) ((fcurnt4 * (*pk++)) >> 15u) + fcurnt3; 00145 gnext4 = __SSAT(gnext4, 16); 00146 00147 /* Update of f values for next coefficient set processing */ 00148 fcurnt1 = fnext1; 00149 fcurnt2 = fnext2; 00150 fcurnt3 = fnext3; 00151 fcurnt4 = fnext4; 00152 00153 00154 /* Loop unrolling. Process 4 taps at a time . */ 00155 stageCnt = (numStages - 1u) >> 2; 00156 00157 00158 /* Loop over the number of taps. Unroll by a factor of 4. 00159 ** Repeat until we've computed numStages-3 coefficients. */ 00160 00161 /* Process 2nd, 3rd, 4th and 5th taps ... here */ 00162 while(stageCnt > 0u) 00163 { 00164 /* Read g1(n-1), g3(n-1) .... from state */ 00165 gcurnt1 = *px; 00166 00167 /* save g1(n) in state buffer */ 00168 *px++ = (q15_t) gnext4; 00169 00170 /* Process first sample for 2nd, 6th .. tap */ 00171 /* Sample processing for K2, K6.... */ 00172 /* f1(n) = f0(n) + K1 * g0(n-1) */ 00173 fnext1 = (q31_t) ((gcurnt1 * (*pk)) >> 15u) + fcurnt1; 00174 fnext1 = __SSAT(fnext1, 16); 00175 00176 00177 /* Process second sample for 2nd, 6th .. tap */ 00178 /* for sample 2 processing */ 00179 fnext2 = (q31_t) ((gnext1 * (*pk)) >> 15u) + fcurnt2; 00180 fnext2 = __SSAT(fnext2, 16); 00181 /* Process third sample for 2nd, 6th .. tap */ 00182 fnext3 = (q31_t) ((gnext2 * (*pk)) >> 15u) + fcurnt3; 00183 fnext3 = __SSAT(fnext3, 16); 00184 /* Process fourth sample for 2nd, 6th .. tap */ 00185 /* fnext4 = fcurnt4 + (*pk) * gnext3; */ 00186 fnext4 = (q31_t) ((gnext3 * (*pk)) >> 15u) + fcurnt4; 00187 fnext4 = __SSAT(fnext4, 16); 00188 00189 /* g1(n) = f0(n) * K1 + g0(n-1) */ 00190 /* Calculation of state values for next stage */ 00191 gnext4 = (q31_t) ((fcurnt4 * (*pk)) >> 15u) + gnext3; 00192 gnext4 = __SSAT(gnext4, 16); 00193 gnext3 = (q31_t) ((fcurnt3 * (*pk)) >> 15u) + gnext2; 00194 gnext3 = __SSAT(gnext3, 16); 00195 00196 gnext2 = (q31_t) ((fcurnt2 * (*pk)) >> 15u) + gnext1; 00197 gnext2 = __SSAT(gnext2, 16); 00198 00199 gnext1 = (q31_t) ((fcurnt1 * (*pk++)) >> 15u) + gcurnt1; 00200 gnext1 = __SSAT(gnext1, 16); 00201 00202 00203 /* Read g2(n-1), g4(n-1) .... from state */ 00204 gcurnt1 = *px; 00205 00206 /* save g1(n) in state buffer */ 00207 *px++ = (q15_t) gnext4; 00208 00209 /* Sample processing for K3, K7.... */ 00210 /* Process first sample for 3rd, 7th .. tap */ 00211 /* f3(n) = f2(n) + K3 * g2(n-1) */ 00212 fcurnt1 = (q31_t) ((gcurnt1 * (*pk)) >> 15u) + fnext1; 00213 fcurnt1 = __SSAT(fcurnt1, 16); 00214 00215 /* Process second sample for 3rd, 7th .. tap */ 00216 fcurnt2 = (q31_t) ((gnext1 * (*pk)) >> 15u) + fnext2; 00217 fcurnt2 = __SSAT(fcurnt2, 16); 00218 00219 /* Process third sample for 3rd, 7th .. tap */ 00220 fcurnt3 = (q31_t) ((gnext2 * (*pk)) >> 15u) + fnext3; 00221 fcurnt3 = __SSAT(fcurnt3, 16); 00222 00223 /* Process fourth sample for 3rd, 7th .. tap */ 00224 fcurnt4 = (q31_t) ((gnext3 * (*pk)) >> 15u) + fnext4; 00225 fcurnt4 = __SSAT(fcurnt4, 16); 00226 00227 /* Calculation of state values for next stage */ 00228 /* g3(n) = f2(n) * K3 + g2(n-1) */ 00229 gnext4 = (q31_t) ((fnext4 * (*pk)) >> 15u) + gnext3; 00230 gnext4 = __SSAT(gnext4, 16); 00231 00232 gnext3 = (q31_t) ((fnext3 * (*pk)) >> 15u) + gnext2; 00233 gnext3 = __SSAT(gnext3, 16); 00234 00235 gnext2 = (q31_t) ((fnext2 * (*pk)) >> 15u) + gnext1; 00236 gnext2 = __SSAT(gnext2, 16); 00237 00238 gnext1 = (q31_t) ((fnext1 * (*pk++)) >> 15u) + gcurnt1; 00239 gnext1 = __SSAT(gnext1, 16); 00240 00241 /* Read g1(n-1), g3(n-1) .... from state */ 00242 gcurnt1 = *px; 00243 00244 /* save g1(n) in state buffer */ 00245 *px++ = (q15_t) gnext4; 00246 00247 /* Sample processing for K4, K8.... */ 00248 /* Process first sample for 4th, 8th .. tap */ 00249 /* f4(n) = f3(n) + K4 * g3(n-1) */ 00250 fnext1 = (q31_t) ((gcurnt1 * (*pk)) >> 15u) + fcurnt1; 00251 fnext1 = __SSAT(fnext1, 16); 00252 00253 /* Process second sample for 4th, 8th .. tap */ 00254 /* for sample 2 processing */ 00255 fnext2 = (q31_t) ((gnext1 * (*pk)) >> 15u) + fcurnt2; 00256 fnext2 = __SSAT(fnext2, 16); 00257 00258 /* Process third sample for 4th, 8th .. tap */ 00259 fnext3 = (q31_t) ((gnext2 * (*pk)) >> 15u) + fcurnt3; 00260 fnext3 = __SSAT(fnext3, 16); 00261 00262 /* Process fourth sample for 4th, 8th .. tap */ 00263 fnext4 = (q31_t) ((gnext3 * (*pk)) >> 15u) + fcurnt4; 00264 fnext4 = __SSAT(fnext4, 16); 00265 00266 /* g4(n) = f3(n) * K4 + g3(n-1) */ 00267 /* Calculation of state values for next stage */ 00268 gnext4 = (q31_t) ((fcurnt4 * (*pk)) >> 15u) + gnext3; 00269 gnext4 = __SSAT(gnext4, 16); 00270 00271 gnext3 = (q31_t) ((fcurnt3 * (*pk)) >> 15u) + gnext2; 00272 gnext3 = __SSAT(gnext3, 16); 00273 00274 gnext2 = (q31_t) ((fcurnt2 * (*pk)) >> 15u) + gnext1; 00275 gnext2 = __SSAT(gnext2, 16); 00276 gnext1 = (q31_t) ((fcurnt1 * (*pk++)) >> 15u) + gcurnt1; 00277 gnext1 = __SSAT(gnext1, 16); 00278 00279 00280 /* Read g2(n-1), g4(n-1) .... from state */ 00281 gcurnt1 = *px; 00282 00283 /* save g4(n) in state buffer */ 00284 *px++ = (q15_t) gnext4; 00285 00286 /* Sample processing for K5, K9.... */ 00287 /* Process first sample for 5th, 9th .. tap */ 00288 /* f5(n) = f4(n) + K5 * g4(n-1) */ 00289 fcurnt1 = (q31_t) ((gcurnt1 * (*pk)) >> 15u) + fnext1; 00290 fcurnt1 = __SSAT(fcurnt1, 16); 00291 00292 /* Process second sample for 5th, 9th .. tap */ 00293 fcurnt2 = (q31_t) ((gnext1 * (*pk)) >> 15u) + fnext2; 00294 fcurnt2 = __SSAT(fcurnt2, 16); 00295 00296 /* Process third sample for 5th, 9th .. tap */ 00297 fcurnt3 = (q31_t) ((gnext2 * (*pk)) >> 15u) + fnext3; 00298 fcurnt3 = __SSAT(fcurnt3, 16); 00299 00300 /* Process fourth sample for 5th, 9th .. tap */ 00301 fcurnt4 = (q31_t) ((gnext3 * (*pk)) >> 15u) + fnext4; 00302 fcurnt4 = __SSAT(fcurnt4, 16); 00303 00304 /* Calculation of state values for next stage */ 00305 /* g5(n) = f4(n) * K5 + g4(n-1) */ 00306 gnext4 = (q31_t) ((fnext4 * (*pk)) >> 15u) + gnext3; 00307 gnext4 = __SSAT(gnext4, 16); 00308 gnext3 = (q31_t) ((fnext3 * (*pk)) >> 15u) + gnext2; 00309 gnext3 = __SSAT(gnext3, 16); 00310 gnext2 = (q31_t) ((fnext2 * (*pk)) >> 15u) + gnext1; 00311 gnext2 = __SSAT(gnext2, 16); 00312 gnext1 = (q31_t) ((fnext1 * (*pk++)) >> 15u) + gcurnt1; 00313 gnext1 = __SSAT(gnext1, 16); 00314 00315 stageCnt--; 00316 } 00317 00318 /* If the (filter length -1) is not a multiple of 4, compute the remaining filter taps */ 00319 stageCnt = (numStages - 1u) % 0x4u; 00320 00321 while(stageCnt > 0u) 00322 { 00323 gcurnt1 = *px; 00324 00325 /* save g value in state buffer */ 00326 *px++ = (q15_t) gnext4; 00327 00328 /* Process four samples for last three taps here */ 00329 fnext1 = (q31_t) ((gcurnt1 * (*pk)) >> 15u) + fcurnt1; 00330 fnext1 = __SSAT(fnext1, 16); 00331 fnext2 = (q31_t) ((gnext1 * (*pk)) >> 15u) + fcurnt2; 00332 fnext2 = __SSAT(fnext2, 16); 00333 00334 fnext3 = (q31_t) ((gnext2 * (*pk)) >> 15u) + fcurnt3; 00335 fnext3 = __SSAT(fnext3, 16); 00336 00337 fnext4 = (q31_t) ((gnext3 * (*pk)) >> 15u) + fcurnt4; 00338 fnext4 = __SSAT(fnext4, 16); 00339 00340 /* g1(n) = f0(n) * K1 + g0(n-1) */ 00341 gnext4 = (q31_t) ((fcurnt4 * (*pk)) >> 15u) + gnext3; 00342 gnext4 = __SSAT(gnext4, 16); 00343 gnext3 = (q31_t) ((fcurnt3 * (*pk)) >> 15u) + gnext2; 00344 gnext3 = __SSAT(gnext3, 16); 00345 gnext2 = (q31_t) ((fcurnt2 * (*pk)) >> 15u) + gnext1; 00346 gnext2 = __SSAT(gnext2, 16); 00347 gnext1 = (q31_t) ((fcurnt1 * (*pk++)) >> 15u) + gcurnt1; 00348 gnext1 = __SSAT(gnext1, 16); 00349 00350 /* Update of f values for next coefficient set processing */ 00351 fcurnt1 = fnext1; 00352 fcurnt2 = fnext2; 00353 fcurnt3 = fnext3; 00354 fcurnt4 = fnext4; 00355 00356 stageCnt--; 00357 00358 } 00359 00360 /* The results in the 4 accumulators, store in the destination buffer. */ 00361 /* y(n) = fN(n) */ 00362 00363 #ifndef ARM_MATH_BIG_ENDIAN 00364 00365 *__SIMD32(pDst)++ = __PKHBT(fcurnt1, fcurnt2, 16); 00366 *__SIMD32(pDst)++ = __PKHBT(fcurnt3, fcurnt4, 16); 00367 00368 #else 00369 00370 *__SIMD32(pDst)++ = __PKHBT(fcurnt2, fcurnt1, 16); 00371 *__SIMD32(pDst)++ = __PKHBT(fcurnt4, fcurnt3, 16); 00372 00373 #endif /* #ifndef ARM_MATH_BIG_ENDIAN */ 00374 00375 blkCnt--; 00376 } 00377 00378 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00379 ** No loop unrolling is used. */ 00380 blkCnt = blockSize % 0x4u; 00381 00382 while(blkCnt > 0u) 00383 { 00384 /* f0(n) = x(n) */ 00385 fcurnt1 = *pSrc++; 00386 00387 /* Initialize coeff pointer */ 00388 pk = (pCoeffs); 00389 00390 /* Initialize state pointer */ 00391 px = pState; 00392 00393 /* read g2(n) from state buffer */ 00394 gcurnt1 = *px; 00395 00396 /* for sample 1 processing */ 00397 /* f1(n) = f0(n) + K1 * g0(n-1) */ 00398 fnext1 = (((q31_t) gcurnt1 * (*pk)) >> 15u) + fcurnt1; 00399 fnext1 = __SSAT(fnext1, 16); 00400 00401 00402 /* g1(n) = f0(n) * K1 + g0(n-1) */ 00403 gnext1 = (((q31_t) fcurnt1 * (*pk++)) >> 15u) + gcurnt1; 00404 gnext1 = __SSAT(gnext1, 16); 00405 00406 /* save g1(n) in state buffer */ 00407 *px++ = (q15_t) fcurnt1; 00408 00409 /* f1(n) is saved in fcurnt1 00410 for next stage processing */ 00411 fcurnt1 = fnext1; 00412 00413 stageCnt = (numStages - 1u); 00414 00415 /* stage loop */ 00416 while(stageCnt > 0u) 00417 { 00418 /* read g2(n) from state buffer */ 00419 gcurnt1 = *px; 00420 00421 /* save g1(n) in state buffer */ 00422 *px++ = (q15_t) gnext1; 00423 00424 /* Sample processing for K2, K3.... */ 00425 /* f2(n) = f1(n) + K2 * g1(n-1) */ 00426 fnext1 = (((q31_t) gcurnt1 * (*pk)) >> 15u) + fcurnt1; 00427 fnext1 = __SSAT(fnext1, 16); 00428 00429 /* g2(n) = f1(n) * K2 + g1(n-1) */ 00430 gnext1 = (((q31_t) fcurnt1 * (*pk++)) >> 15u) + gcurnt1; 00431 gnext1 = __SSAT(gnext1, 16); 00432 00433 00434 /* f1(n) is saved in fcurnt1 00435 for next stage processing */ 00436 fcurnt1 = fnext1; 00437 00438 stageCnt--; 00439 00440 } 00441 00442 /* y(n) = fN(n) */ 00443 *pDst++ = __SSAT(fcurnt1, 16); 00444 00445 00446 blkCnt--; 00447 00448 } 00449 00450 #else 00451 00452 /* Run the below code for Cortex-M0 */ 00453 00454 q31_t fcurnt, fnext, gcurnt, gnext; /* temporary variables */ 00455 uint32_t numStages = S->numStages; /* Length of the filter */ 00456 uint32_t blkCnt, stageCnt; /* temporary variables for counts */ 00457 00458 pState = &S->pState[0]; 00459 00460 blkCnt = blockSize; 00461 00462 while(blkCnt > 0u) 00463 { 00464 /* f0(n) = x(n) */ 00465 fcurnt = *pSrc++; 00466 00467 /* Initialize coeff pointer */ 00468 pk = (pCoeffs); 00469 00470 /* Initialize state pointer */ 00471 px = pState; 00472 00473 /* read g0(n-1) from state buffer */ 00474 gcurnt = *px; 00475 00476 /* for sample 1 processing */ 00477 /* f1(n) = f0(n) + K1 * g0(n-1) */ 00478 fnext = ((gcurnt * (*pk)) >> 15u) + fcurnt; 00479 fnext = __SSAT(fnext, 16); 00480 00481 00482 /* g1(n) = f0(n) * K1 + g0(n-1) */ 00483 gnext = ((fcurnt * (*pk++)) >> 15u) + gcurnt; 00484 gnext = __SSAT(gnext, 16); 00485 00486 /* save f0(n) in state buffer */ 00487 *px++ = (q15_t) fcurnt; 00488 00489 /* f1(n) is saved in fcurnt 00490 for next stage processing */ 00491 fcurnt = fnext; 00492 00493 stageCnt = (numStages - 1u); 00494 00495 /* stage loop */ 00496 while(stageCnt > 0u) 00497 { 00498 /* read g1(n-1) from state buffer */ 00499 gcurnt = *px; 00500 00501 /* save g0(n-1) in state buffer */ 00502 *px++ = (q15_t) gnext; 00503 00504 /* Sample processing for K2, K3.... */ 00505 /* f2(n) = f1(n) + K2 * g1(n-1) */ 00506 fnext = ((gcurnt * (*pk)) >> 15u) + fcurnt; 00507 fnext = __SSAT(fnext, 16); 00508 00509 /* g2(n) = f1(n) * K2 + g1(n-1) */ 00510 gnext = ((fcurnt * (*pk++)) >> 15u) + gcurnt; 00511 gnext = __SSAT(gnext, 16); 00512 00513 00514 /* f1(n) is saved in fcurnt 00515 for next stage processing */ 00516 fcurnt = fnext; 00517 00518 stageCnt--; 00519 00520 } 00521 00522 /* y(n) = fN(n) */ 00523 *pDst++ = __SSAT(fcurnt, 16); 00524 00525 00526 blkCnt--; 00527 00528 } 00529 00530 #endif /* #ifndef ARM_MATH_CM0_FAMILY */ 00531 00532 } 00533 00534 /** 00535 * @} end of FIR_Lattice group 00536 */
Generated on Tue Jul 12 2022 11:59:17 by
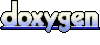