CMSIS DSP library
Dependents: performance_timer Surfboard_ gps2rtty Capstone ... more
arm_fir_interpolate_init_q15.c
00001 /*----------------------------------------------------------------------------- 00002 * Copyright (C) 2010-2014 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 19. March 2015 00005 * $Revision: V.1.4.5 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_fir_interpolate_init_q15.c 00009 * 00010 * Description: Q15 FIR interpolator initialization function 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * ---------------------------------------------------------------------------*/ 00040 00041 #include "arm_math.h" 00042 00043 /** 00044 * @ingroup groupFilters 00045 */ 00046 00047 /** 00048 * @addtogroup FIR_Interpolate 00049 * @{ 00050 */ 00051 00052 /** 00053 * @brief Initialization function for the Q15 FIR interpolator. 00054 * @param[in,out] *S points to an instance of the Q15 FIR interpolator structure. 00055 * @param[in] L upsample factor. 00056 * @param[in] numTaps number of filter coefficients in the filter. 00057 * @param[in] *pCoeffs points to the filter coefficient buffer. 00058 * @param[in] *pState points to the state buffer. 00059 * @param[in] blockSize number of input samples to process per call. 00060 * @return The function returns ARM_MATH_SUCCESS if initialization was successful or ARM_MATH_LENGTH_ERROR if 00061 * the filter length <code>numTaps</code> is not a multiple of the interpolation factor <code>L</code>. 00062 * 00063 * <b>Description:</b> 00064 * \par 00065 * <code>pCoeffs</code> points to the array of filter coefficients stored in time reversed order: 00066 * <pre> 00067 * {b[numTaps-1], b[numTaps-2], b[numTaps-2], ..., b[1], b[0]} 00068 * </pre> 00069 * The length of the filter <code>numTaps</code> must be a multiple of the interpolation factor <code>L</code>. 00070 * \par 00071 * <code>pState</code> points to the array of state variables. 00072 * <code>pState</code> is of length <code>(numTaps/L)+blockSize-1</code> words 00073 * where <code>blockSize</code> is the number of input samples processed by each call to <code>arm_fir_interpolate_q15()</code>. 00074 */ 00075 00076 arm_status arm_fir_interpolate_init_q15( 00077 arm_fir_interpolate_instance_q15 * S, 00078 uint8_t L, 00079 uint16_t numTaps, 00080 q15_t * pCoeffs, 00081 q15_t * pState, 00082 uint32_t blockSize) 00083 { 00084 arm_status status; 00085 00086 /* The filter length must be a multiple of the interpolation factor */ 00087 if((numTaps % L) != 0u) 00088 { 00089 /* Set status as ARM_MATH_LENGTH_ERROR */ 00090 status = ARM_MATH_LENGTH_ERROR; 00091 } 00092 else 00093 { 00094 00095 /* Assign coefficient pointer */ 00096 S->pCoeffs = pCoeffs; 00097 00098 /* Assign Interpolation factor */ 00099 S->L = L; 00100 00101 /* Assign polyPhaseLength */ 00102 S->phaseLength = numTaps / L; 00103 00104 /* Clear state buffer and size of buffer is always phaseLength + blockSize - 1 */ 00105 memset(pState, 0, 00106 (blockSize + ((uint32_t) S->phaseLength - 1u)) * sizeof(q15_t)); 00107 00108 /* Assign state pointer */ 00109 S->pState = pState; 00110 00111 status = ARM_MATH_SUCCESS; 00112 } 00113 00114 return (status); 00115 00116 } 00117 00118 /** 00119 * @} end of FIR_Interpolate group 00120 */
Generated on Tue Jul 12 2022 11:59:17 by
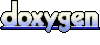