CMSIS DSP library
Dependents: performance_timer Surfboard_ gps2rtty Capstone ... more
arm_cmplx_mag_q15.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010-2014 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 19. March 2015 00005 * $Revision: V.1.4.5 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_cmplx_mag_q15.c 00009 * 00010 * Description: Q15 complex magnitude. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * ---------------------------------------------------------------------------- */ 00040 00041 #include "arm_math.h" 00042 00043 /** 00044 * @ingroup groupCmplxMath 00045 */ 00046 00047 /** 00048 * @addtogroup cmplx_mag 00049 * @{ 00050 */ 00051 00052 00053 /** 00054 * @brief Q15 complex magnitude 00055 * @param *pSrc points to the complex input vector 00056 * @param *pDst points to the real output vector 00057 * @param numSamples number of complex samples in the input vector 00058 * @return none. 00059 * 00060 * <b>Scaling and Overflow Behavior:</b> 00061 * \par 00062 * The function implements 1.15 by 1.15 multiplications and finally output is converted into 2.14 format. 00063 */ 00064 00065 void arm_cmplx_mag_q15( 00066 q15_t * pSrc, 00067 q15_t * pDst, 00068 uint32_t numSamples) 00069 { 00070 q31_t acc0, acc1; /* Accumulators */ 00071 00072 #ifndef ARM_MATH_CM0_FAMILY 00073 00074 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00075 uint32_t blkCnt; /* loop counter */ 00076 q31_t in1, in2, in3, in4; 00077 q31_t acc2, acc3; 00078 00079 00080 /*loop Unrolling */ 00081 blkCnt = numSamples >> 2u; 00082 00083 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00084 ** a second loop below computes the remaining 1 to 3 samples. */ 00085 while(blkCnt > 0u) 00086 { 00087 00088 /* C[0] = sqrt(A[0] * A[0] + A[1] * A[1]) */ 00089 in1 = *__SIMD32(pSrc)++; 00090 in2 = *__SIMD32(pSrc)++; 00091 in3 = *__SIMD32(pSrc)++; 00092 in4 = *__SIMD32(pSrc)++; 00093 00094 acc0 = __SMUAD(in1, in1); 00095 acc1 = __SMUAD(in2, in2); 00096 acc2 = __SMUAD(in3, in3); 00097 acc3 = __SMUAD(in4, in4); 00098 00099 /* store the result in 2.14 format in the destination buffer. */ 00100 arm_sqrt_q15((q15_t) ((acc0) >> 17), pDst++); 00101 arm_sqrt_q15((q15_t) ((acc1) >> 17), pDst++); 00102 arm_sqrt_q15((q15_t) ((acc2) >> 17), pDst++); 00103 arm_sqrt_q15((q15_t) ((acc3) >> 17), pDst++); 00104 00105 /* Decrement the loop counter */ 00106 blkCnt--; 00107 } 00108 00109 /* If the numSamples is not a multiple of 4, compute any remaining output samples here. 00110 ** No loop unrolling is used. */ 00111 blkCnt = numSamples % 0x4u; 00112 00113 while(blkCnt > 0u) 00114 { 00115 /* C[0] = sqrt(A[0] * A[0] + A[1] * A[1]) */ 00116 in1 = *__SIMD32(pSrc)++; 00117 acc0 = __SMUAD(in1, in1); 00118 00119 /* store the result in 2.14 format in the destination buffer. */ 00120 arm_sqrt_q15((q15_t) (acc0 >> 17), pDst++); 00121 00122 /* Decrement the loop counter */ 00123 blkCnt--; 00124 } 00125 00126 #else 00127 00128 /* Run the below code for Cortex-M0 */ 00129 q15_t real, imag; /* Temporary variables to hold input values */ 00130 00131 while(numSamples > 0u) 00132 { 00133 /* out = sqrt(real * real + imag * imag) */ 00134 real = *pSrc++; 00135 imag = *pSrc++; 00136 00137 acc0 = (real * real); 00138 acc1 = (imag * imag); 00139 00140 /* store the result in 2.14 format in the destination buffer. */ 00141 arm_sqrt_q15((q15_t) (((q63_t) acc0 + acc1) >> 17), pDst++); 00142 00143 /* Decrement the loop counter */ 00144 numSamples--; 00145 } 00146 00147 #endif /* #ifndef ARM_MATH_CM0_FAMILY */ 00148 00149 } 00150 00151 /** 00152 * @} end of cmplx_mag group 00153 */
Generated on Tue Jul 12 2022 11:59:15 by
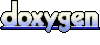