CMSIS DSP library
Dependents: performance_timer Surfboard_ gps2rtty Capstone ... more
arm_cmplx_conj_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010-2014 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 19. March 2015 00005 * $Revision: V.1.4.5 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_cmplx_conj_f32.c 00009 * 00010 * Description: Floating-point complex conjugate. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * ---------------------------------------------------------------------------- */ 00040 #include "arm_math.h" 00041 00042 /** 00043 * @ingroup groupCmplxMath 00044 */ 00045 00046 /** 00047 * @defgroup cmplx_conj Complex Conjugate 00048 * 00049 * Conjugates the elements of a complex data vector. 00050 * 00051 * The <code>pSrc</code> points to the source data and 00052 * <code>pDst</code> points to the where the result should be written. 00053 * <code>numSamples</code> specifies the number of complex samples 00054 * and the data in each array is stored in an interleaved fashion 00055 * (real, imag, real, imag, ...). 00056 * Each array has a total of <code>2*numSamples</code> values. 00057 * The underlying algorithm is used: 00058 * 00059 * <pre> 00060 * for(n=0; n<numSamples; n++) { 00061 * pDst[(2*n)+0)] = pSrc[(2*n)+0]; // real part 00062 * pDst[(2*n)+1)] = -pSrc[(2*n)+1]; // imag part 00063 * } 00064 * </pre> 00065 * 00066 * There are separate functions for floating-point, Q15, and Q31 data types. 00067 */ 00068 00069 /** 00070 * @addtogroup cmplx_conj 00071 * @{ 00072 */ 00073 00074 /** 00075 * @brief Floating-point complex conjugate. 00076 * @param *pSrc points to the input vector 00077 * @param *pDst points to the output vector 00078 * @param numSamples number of complex samples in each vector 00079 * @return none. 00080 */ 00081 00082 void arm_cmplx_conj_f32( 00083 float32_t * pSrc, 00084 float32_t * pDst, 00085 uint32_t numSamples) 00086 { 00087 uint32_t blkCnt; /* loop counter */ 00088 00089 #ifndef ARM_MATH_CM0_FAMILY 00090 00091 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00092 float32_t inR1, inR2, inR3, inR4; 00093 float32_t inI1, inI2, inI3, inI4; 00094 00095 /*loop Unrolling */ 00096 blkCnt = numSamples >> 2u; 00097 00098 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00099 ** a second loop below computes the remaining 1 to 3 samples. */ 00100 while(blkCnt > 0u) 00101 { 00102 /* C[0]+jC[1] = A[0]+ j (-1) A[1] */ 00103 /* Calculate Complex Conjugate and then store the results in the destination buffer. */ 00104 /* read real input samples */ 00105 inR1 = pSrc[0]; 00106 /* store real samples to destination */ 00107 pDst[0] = inR1; 00108 inR2 = pSrc[2]; 00109 pDst[2] = inR2; 00110 inR3 = pSrc[4]; 00111 pDst[4] = inR3; 00112 inR4 = pSrc[6]; 00113 pDst[6] = inR4; 00114 00115 /* read imaginary input samples */ 00116 inI1 = pSrc[1]; 00117 inI2 = pSrc[3]; 00118 00119 /* conjugate input */ 00120 inI1 = -inI1; 00121 00122 /* read imaginary input samples */ 00123 inI3 = pSrc[5]; 00124 00125 /* conjugate input */ 00126 inI2 = -inI2; 00127 00128 /* read imaginary input samples */ 00129 inI4 = pSrc[7]; 00130 00131 /* conjugate input */ 00132 inI3 = -inI3; 00133 00134 /* store imaginary samples to destination */ 00135 pDst[1] = inI1; 00136 pDst[3] = inI2; 00137 00138 /* conjugate input */ 00139 inI4 = -inI4; 00140 00141 /* store imaginary samples to destination */ 00142 pDst[5] = inI3; 00143 00144 /* increment source pointer by 8 to process next sampels */ 00145 pSrc += 8u; 00146 00147 /* store imaginary sample to destination */ 00148 pDst[7] = inI4; 00149 00150 /* increment destination pointer by 8 to store next samples */ 00151 pDst += 8u; 00152 00153 /* Decrement the loop counter */ 00154 blkCnt--; 00155 } 00156 00157 /* If the numSamples is not a multiple of 4, compute any remaining output samples here. 00158 ** No loop unrolling is used. */ 00159 blkCnt = numSamples % 0x4u; 00160 00161 #else 00162 00163 /* Run the below code for Cortex-M0 */ 00164 blkCnt = numSamples; 00165 00166 #endif /* #ifndef ARM_MATH_CM0_FAMILY */ 00167 00168 while(blkCnt > 0u) 00169 { 00170 /* realOut + j (imagOut) = realIn + j (-1) imagIn */ 00171 /* Calculate Complex Conjugate and then store the results in the destination buffer. */ 00172 *pDst++ = *pSrc++; 00173 *pDst++ = -*pSrc++; 00174 00175 /* Decrement the loop counter */ 00176 blkCnt--; 00177 } 00178 } 00179 00180 /** 00181 * @} end of cmplx_conj group 00182 */
Generated on Tue Jul 12 2022 11:59:15 by
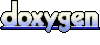